1207. Unique Number of Occurrences LeetCode Solution
In this guide, you will get 1207. Unique Number of Occurrences LeetCode Solution with the best time and space complexity. The solution to Unique Number of Occurrences problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Unique Number of Occurrences solution in C++
- Unique Number of Occurrences solution in Java
- Unique Number of Occurrences solution in Python
- Additional Resources
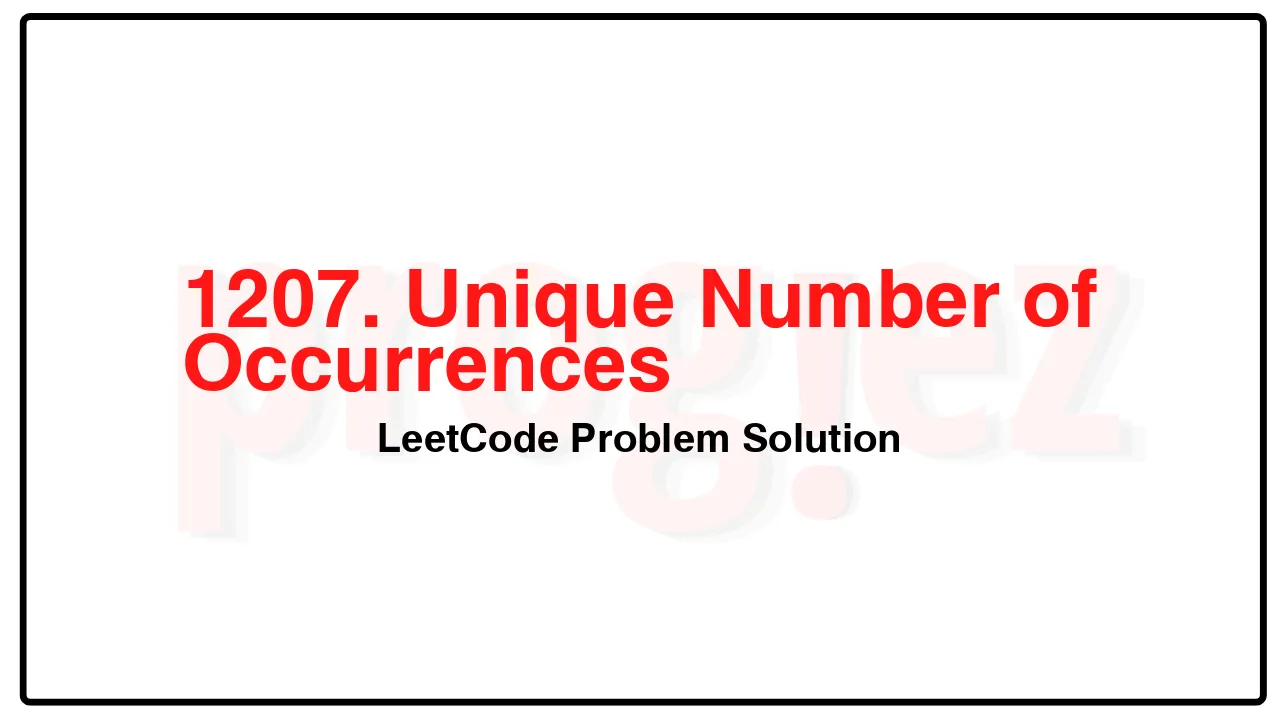
Problem Statement of Unique Number of Occurrences
Given an array of integers arr, return true if the number of occurrences of each value in the array is unique or false otherwise.
Example 1:
Input: arr = [1,2,2,1,1,3]
Output: true
Explanation: The value 1 has 3 occurrences, 2 has 2 and 3 has 1. No two values have the same number of occurrences.
Example 2:
Input: arr = [1,2]
Output: false
Example 3:
Input: arr = [-3,0,1,-3,1,1,1,-3,10,0]
Output: true
Constraints:
1 <= arr.length <= 1000
-1000 <= arr[i] <= 1000
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
1207. Unique Number of Occurrences LeetCode Solution in C++
class Solution {
public:
bool uniqueOccurrences(vector<int>& arr) {
unordered_map<int, int> count;
unordered_set<int> occurrences;
for (const int a : arr)
++count[a];
for (const auto& [_, value] : count)
if (!occurrences.insert(value).second)
return false;
return true;
}
};
/* code provided by PROGIEZ */
1207. Unique Number of Occurrences LeetCode Solution in Java
class Solution {
public boolean uniqueOccurrences(int[] arr) {
Map<Integer, Integer> count = new HashMap<>();
Set<Integer> occurrences = new HashSet<>();
for (final int a : arr)
count.merge(a, 1, Integer::sum);
for (final int value : count.values())
if (!occurrences.add(value))
return false;
return true;
}
}
// code provided by PROGIEZ
1207. Unique Number of Occurrences LeetCode Solution in Python
class Solution:
def uniqueOccurrences(self, arr: list[int]) -> bool:
count = collections.Counter(arr)
occurrences = set()
for value in count.values():
if value in occurrences:
return False
occurrences.add(value)
return True
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.