1161. Maximum Level Sum of a Binary Tree LeetCode Solution
In this guide, you will get 1161. Maximum Level Sum of a Binary Tree LeetCode Solution with the best time and space complexity. The solution to Maximum Level Sum of a Binary Tree problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Maximum Level Sum of a Binary Tree solution in C++
- Maximum Level Sum of a Binary Tree solution in Java
- Maximum Level Sum of a Binary Tree solution in Python
- Additional Resources
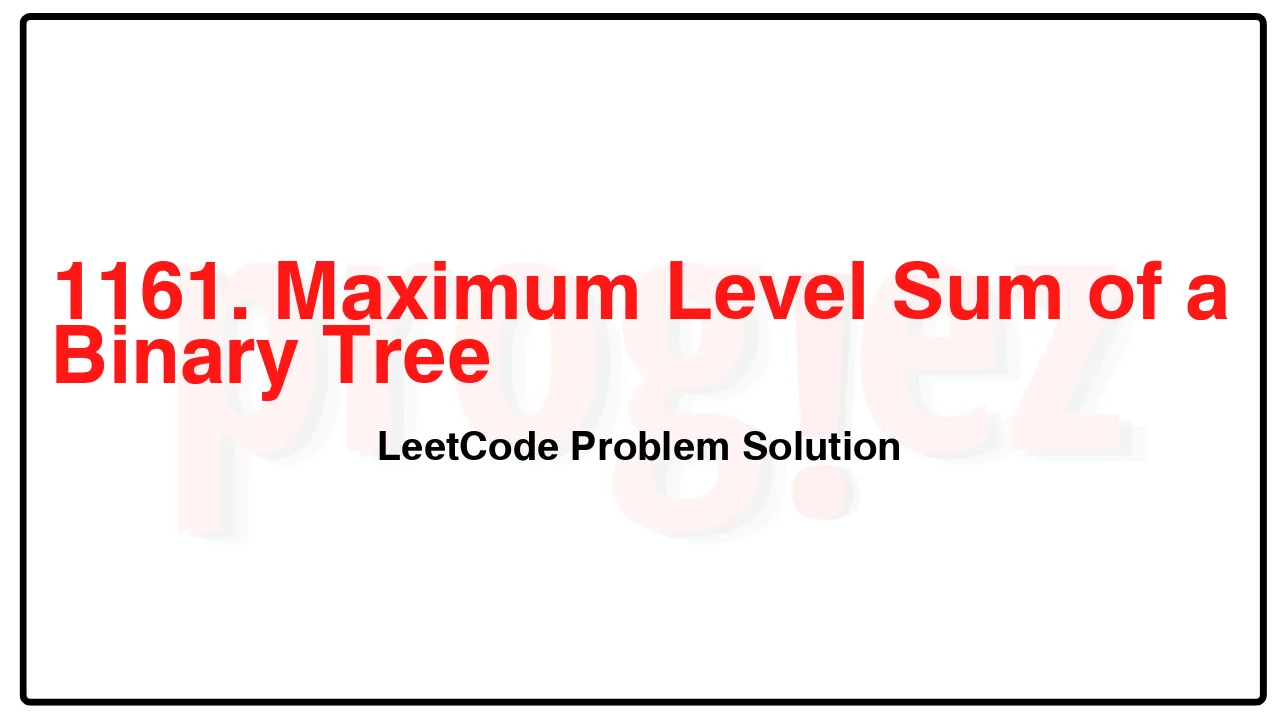
Problem Statement of Maximum Level Sum of a Binary Tree
Given the root of a binary tree, the level of its root is 1, the level of its children is 2, and so on.
Return the smallest level x such that the sum of all the values of nodes at level x is maximal.
Example 1:
Input: root = [1,7,0,7,-8,null,null]
Output: 2
Explanation:
Level 1 sum = 1.
Level 2 sum = 7 + 0 = 7.
Level 3 sum = 7 + -8 = -1.
So we return the level with the maximum sum which is level 2.
Example 2:
Input: root = [989,null,10250,98693,-89388,null,null,null,-32127]
Output: 2
Constraints:
The number of nodes in the tree is in the range [1, 104].
-105 <= Node.val <= 105
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
1161. Maximum Level Sum of a Binary Tree LeetCode Solution in C++
class Solution {
public:
int maxLevelSum(TreeNode* root) {
int ans = 0;
int maxLevelSum = INT_MIN;
queue<TreeNode*> q{{root}};
for (int level = 1; !q.empty(); ++level) {
int levelSum = 0;
for (int sz = q.size(); sz > 0; --sz) {
TreeNode* node = q.front();
q.pop();
levelSum += node->val;
if (node->left != nullptr)
q.push(node->left);
if (node->right != nullptr)
q.push(node->right);
}
if (levelSum > maxLevelSum) {
maxLevelSum = levelSum;
ans = level;
}
}
return ans;
}
};
/* code provided by PROGIEZ */
1161. Maximum Level Sum of a Binary Tree LeetCode Solution in Java
class Solution {
public int maxLevelSum(TreeNode root) {
int ans = 0;
int maxLevelSum = Integer.MIN_VALUE;
Queue<TreeNode> q = new LinkedList<>(Arrays.asList(root));
for (int level = 1; !q.isEmpty(); ++level) {
int levelSum = 0;
for (int sz = q.size(); sz > 0; --sz) {
TreeNode node = q.poll();
levelSum += node.val;
if (node.left != null)
q.offer(node.left);
if (node.right != null)
q.offer(node.right);
}
if (levelSum > maxLevelSum) {
maxLevelSum = levelSum;
ans = level;
}
}
return ans;
}
}
// code provided by PROGIEZ
1161. Maximum Level Sum of a Binary Tree LeetCode Solution in Python
class Solution:
def maxLevelSum(self, root: TreeNode | None) -> int:
ans = 0
maxLevelSum = -math.inf
q = collections.deque([root])
level = 1
while q:
levelSum = 0
for _ in range(len(q)):
node = q.popleft()
levelSum += node.val
if node.left:
q.append(node.left)
if node.right:
q.append(node.right)
if levelSum > maxLevelSum:
maxLevelSum = levelSum
ans = level
level += 1
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.