1103. Distribute Candies to People LeetCode Solution
In this guide, you will get 1103. Distribute Candies to People LeetCode Solution with the best time and space complexity. The solution to Distribute Candies to People problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Distribute Candies to People solution in C++
- Distribute Candies to People solution in Java
- Distribute Candies to People solution in Python
- Additional Resources
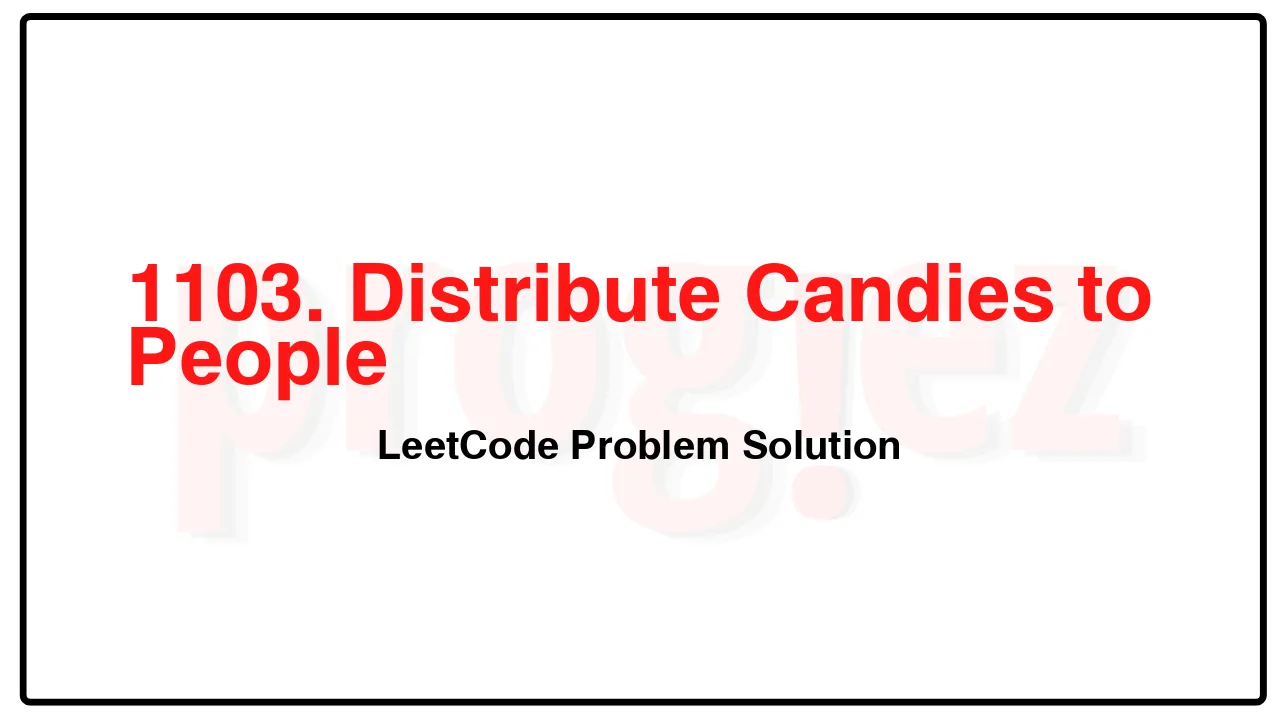
Problem Statement of Distribute Candies to People
We distribute some number of candies, to a row of n = num_people people in the following way:
We then give 1 candy to the first person, 2 candies to the second person, and so on until we give n candies to the last person.
Then, we go back to the start of the row, giving n + 1 candies to the first person, n + 2 candies to the second person, and so on until we give 2 * n candies to the last person.
This process repeats (with us giving one more candy each time, and moving to the start of the row after we reach the end) until we run out of candies. The last person will receive all of our remaining candies (not necessarily one more than the previous gift).
Return an array (of length num_people and sum candies) that represents the final distribution of candies.
Example 1:
Input: candies = 7, num_people = 4
Output: [1,2,3,1]
Explanation:
On the first turn, ans[0] += 1, and the array is [1,0,0,0].
On the second turn, ans[1] += 2, and the array is [1,2,0,0].
On the third turn, ans[2] += 3, and the array is [1,2,3,0].
On the fourth turn, ans[3] += 1 (because there is only one candy left), and the final array is [1,2,3,1].
Example 2:
Input: candies = 10, num_people = 3
Output: [5,2,3]
Explanation:
On the first turn, ans[0] += 1, and the array is [1,0,0].
On the second turn, ans[1] += 2, and the array is [1,2,0].
On the third turn, ans[2] += 3, and the array is [1,2,3].
On the fourth turn, ans[0] += 4, and the final array is [5,2,3].
Constraints:
1 <= candies <= 10^9
1 <= num_people <= 1000
Complexity Analysis
- Time Complexity:
- Space Complexity:
1103. Distribute Candies to People LeetCode Solution in C++
class Solution {
public:
vector<int> distributeCandies(int candies, long n) {
vector<int> ans(n);
int rows = (-n + sqrt(n * n + 8 * n * n * candies)) / (2 * n * n);
int accumN = rows * (rows - 1) * n / 2;
for (int i = 0; i < n; ++i)
ans[i] = accumN + rows * (i + 1);
int givenCandies = (n * n * rows * rows + n * rows) / 2;
candies -= givenCandies;
for (int i = 0, lastGiven = rows * n + 1; candies > 0; ++i, ++lastGiven) {
int actualGiven = min(lastGiven, candies);
candies -= actualGiven;
ans[i] += actualGiven;
}
return ans;
}
};
/* code provided by PROGIEZ */
1103. Distribute Candies to People LeetCode Solution in Java
class Solution {
public int[] distributeCandies(int candies, int num_people) {
int[] ans = new int[num_people];
long c = (long) candies;
long n = (long) num_people;
int rows = (int) (-n + Math.sqrt(n * n + 8 * n * n * c)) / (int) (2 * n * n);
int accumN = rows * (rows - 1) * num_people / 2;
for (int i = 0; i < n; ++i)
ans[i] = accumN + rows * (i + 1);
int givenCandies = (num_people * num_people * rows * rows + num_people * rows) / 2;
candies -= givenCandies;
for (int i = 0, lastGiven = rows * num_people + 1; candies > 0; ++i, ++lastGiven) {
int actualGiven = Math.min(lastGiven, candies);
candies -= actualGiven;
ans[i] += actualGiven;
}
return ans;
}
}
// code provided by PROGIEZ
1103. Distribute Candies to People LeetCode Solution in Python
class Solution:
def distributeCandies(self, candies: int, n: int) -> list[int]:
ans = [0] * n
rows = int((-n + (n**2 + 8 * n**2 * candies)**0.5) / (2 * n**2))
accumN = rows * (rows - 1) * n // 2
for i in range(n):
ans[i] = accumN + rows * (i + 1)
givenCandies = (n**2 * rows**2 + n * rows) // 2
candies -= givenCandies
lastGiven = rows * n
i = 0
while candies > 0:
lastGiven += 1
actualGiven = min(lastGiven, candies)
candies -= actualGiven
ans[i] += actualGiven
i += 1
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.