1071. Greatest Common Divisor of Strings LeetCode Solution
In this guide, you will get 1071. Greatest Common Divisor of Strings LeetCode Solution with the best time and space complexity. The solution to Greatest Common Divisor of Strings problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Greatest Common Divisor of Strings solution in C++
- Greatest Common Divisor of Strings solution in Java
- Greatest Common Divisor of Strings solution in Python
- Additional Resources
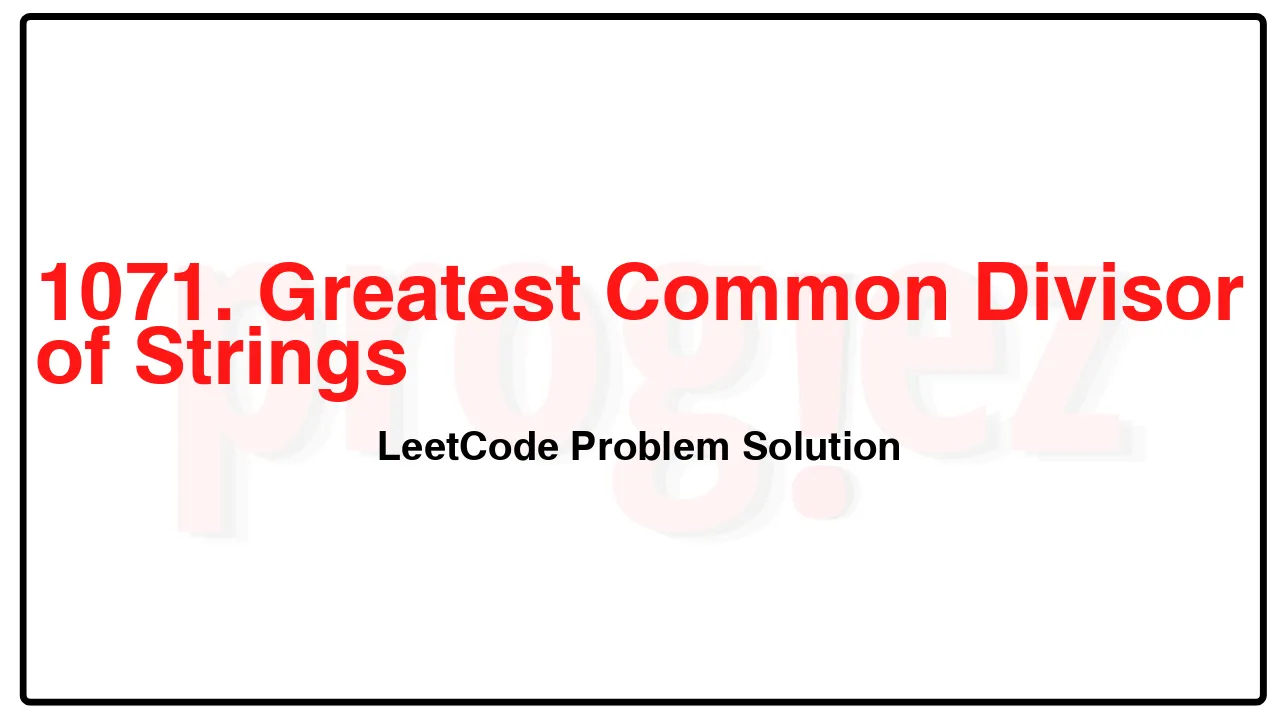
Problem Statement of Greatest Common Divisor of Strings
For two strings s and t, we say “t divides s” if and only if s = t + t + t + … + t + t (i.e., t is concatenated with itself one or more times).
Given two strings str1 and str2, return the largest string x such that x divides both str1 and str2.
Example 1:
Input: str1 = “ABCABC”, str2 = “ABC”
Output: “ABC”
Example 2:
Input: str1 = “ABABAB”, str2 = “ABAB”
Output: “AB”
Example 3:
Input: str1 = “LEET”, str2 = “CODE”
Output: “”
Constraints:
1 <= str1.length, str2.length <= 1000
str1 and str2 consist of English uppercase letters.
Complexity Analysis
- Time Complexity:
- Space Complexity:
1071. Greatest Common Divisor of Strings LeetCode Solution in C++
class Solution {
public:
string gcdOfStrings(string str1, string str2) {
if (str1.length() < str2.length())
return gcdOfStrings(str2, str1);
if (str1.find(str2) == string::npos)
return "";
if (str2.empty())
return str1;
return gcdOfStrings(str2, mod(str1, str2));
}
private:
string mod(string& s1, const string& s2) {
while (s1.find(s2) == 0)
s1 = s1.substr(s2.length());
return s1;
}
};
/* code provided by PROGIEZ */
1071. Greatest Common Divisor of Strings LeetCode Solution in Java
class Solution {
public String gcdOfStrings(String str1, String str2) {
if (str1.length() < str2.length())
return gcdOfStrings(str2, str1);
if (!str1.startsWith(str2))
return "";
if (str2.isEmpty())
return str1;
return gcdOfStrings(str2, mod(str1, str2));
}
private String mod(String s1, final String s2) {
while (s1.startsWith(s2))
s1 = s1.substring(s2.length());
return s1;
}
}
// code provided by PROGIEZ
1071. Greatest Common Divisor of Strings LeetCode Solution in Python
class Solution:
def gcdOfStrings(self, str1: str, str2: str) -> str:
def mod(s1: str, s2: str) -> str:
while s1.startswith(s2):
s1 = s1[len(s2):]
return s1
if len(str1) < len(str2):
return self.gcdOfStrings(str2, str1)
if not str1.startswith(str2):
return ''
if not str2:
return str1
return self.gcdOfStrings(str2, mod(str1, str2))
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.