1007. Minimum Domino Rotations For Equal Row LeetCode Solution
In this guide, you will get 1007. Minimum Domino Rotations For Equal Row LeetCode Solution with the best time and space complexity. The solution to Minimum Domino Rotations For Equal Row problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Minimum Domino Rotations For Equal Row solution in C++
- Minimum Domino Rotations For Equal Row solution in Java
- Minimum Domino Rotations For Equal Row solution in Python
- Additional Resources
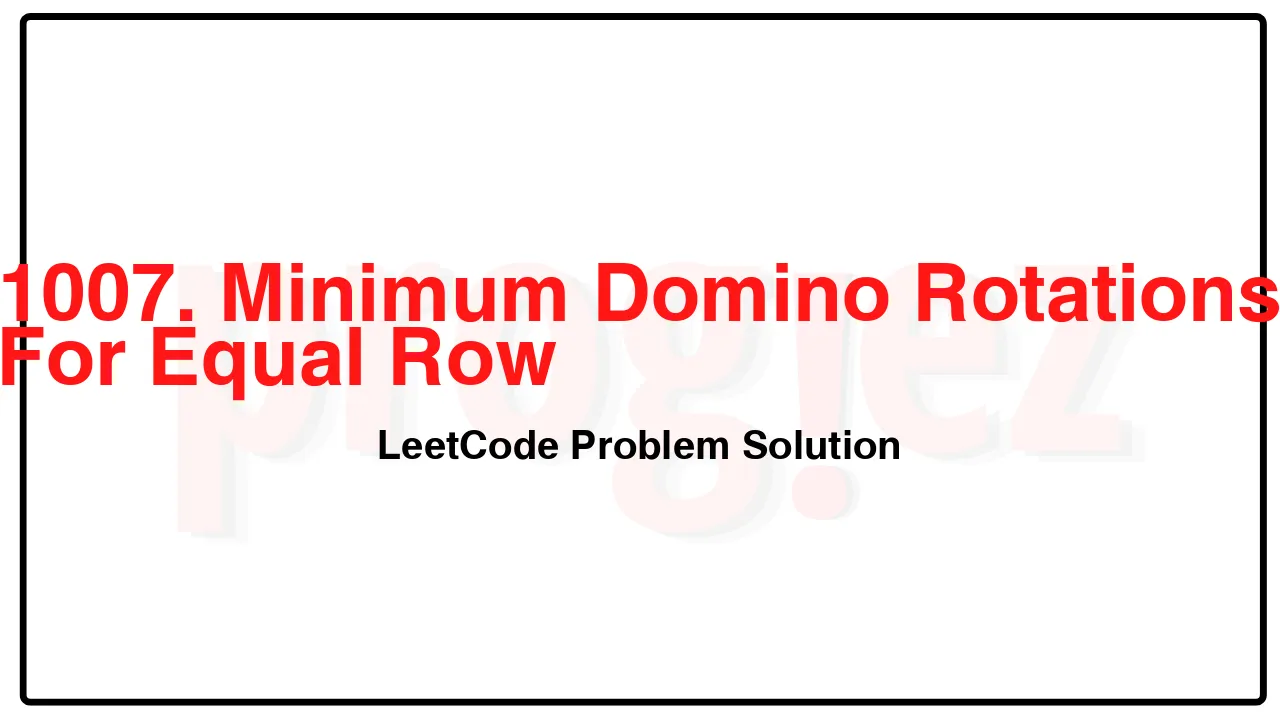
Problem Statement of Minimum Domino Rotations For Equal Row
In a row of dominoes, tops[i] and bottoms[i] represent the top and bottom halves of the ith domino. (A domino is a tile with two numbers from 1 to 6 – one on each half of the tile.)
We may rotate the ith domino, so that tops[i] and bottoms[i] swap values.
Return the minimum number of rotations so that all the values in tops are the same, or all the values in bottoms are the same.
If it cannot be done, return -1.
Example 1:
Input: tops = [2,1,2,4,2,2], bottoms = [5,2,6,2,3,2]
Output: 2
Explanation:
The first figure represents the dominoes as given by tops and bottoms: before we do any rotations.
If we rotate the second and fourth dominoes, we can make every value in the top row equal to 2, as indicated by the second figure.
Example 2:
Input: tops = [3,5,1,2,3], bottoms = [3,6,3,3,4]
Output: -1
Explanation:
In this case, it is not possible to rotate the dominoes to make one row of values equal.
Constraints:
2 <= tops.length <= 2 * 104
bottoms.length == tops.length
1 <= tops[i], bottoms[i] <= 6
Complexity Analysis
- Time Complexity:
- Space Complexity:
1007. Minimum Domino Rotations For Equal Row LeetCode Solution in C++
class Solution {
public:
int minDominoRotations(vector<int>& tops, vector<int>& bottoms) {
const int n = tops.size();
vector<int> countTops(7);
vector<int> countBottoms(7);
vector<int> countBoth(7);
for (int i = 0; i < n; ++i) {
++countTops[tops[i]];
++countBottoms[bottoms[i]];
if (tops[i] == bottoms[i])
++countBoth[tops[i]];
}
for (int i = 1; i <= 6; ++i)
if (countTops[i] + countBottoms[i] - countBoth[i] == n)
return n - max(countTops[i], countBottoms[i]);
return -1;
}
};
/* code provided by PROGIEZ */
1007. Minimum Domino Rotations For Equal Row LeetCode Solution in Java
class Solution {
public int minDominoRotations(int[] tops, int[] bottoms) {
final int n = tops.length;
int[] countTops = new int[7];
int[] countBottoms = new int[7];
int[] countBoth = new int[7];
for (int i = 0; i < n; ++i) {
++countTops[tops[i]];
++countBottoms[bottoms[i]];
if (tops[i] == bottoms[i])
++countBoth[tops[i]];
}
for (int i = 1; i <= 6; ++i)
if (countTops[i] + countBottoms[i] - countBoth[i] == n)
return n - Math.max(countTops[i], countBottoms[i]);
return -1;
}
}
// code provided by PROGIEZ
1007. Minimum Domino Rotations For Equal Row LeetCode Solution in Python
class Solution:
def minDominoRotations(self, tops: list[int], bottoms: list[int]) -> int:
for num in range(1, 7):
if all(num in pair for pair in zip(tops, bottoms)):
return len(tops) - max(tops.count(num), bottoms.count(num))
return -1
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.