The Joy of Computing Using Python NPTEL Week 7 Answers
Are you looking for The Joy of Computing Using Python NPTEL Week 7 Answers? You’ve come to the right place! Access the latest and most accurate solutions for your Assignment 7 in The Joy of Computing Using Python course
Course Link: Click Here
Table of Contents
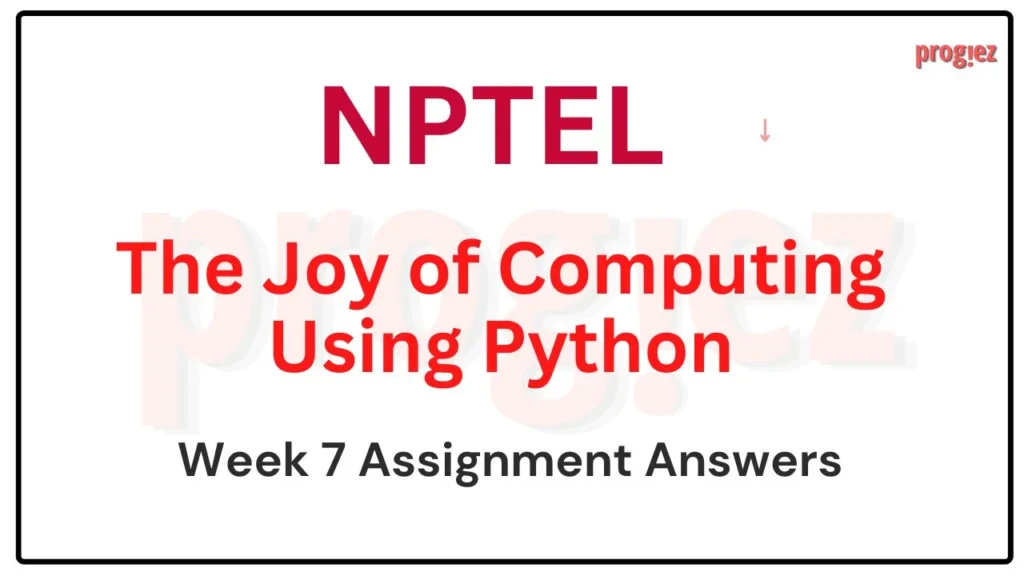
The Joy of Computing Using Python NPTEL Week 7 Answers (July-Dec 2024)
1. Which of the following methods is used to read the content of a CSV file in Python using the csv module?
A) csv.reader()
B) csv.write()
C) csv.load()
D) csv.readfile()
Answer: A) csv.reader()
2. Which command is used to install a Python package using pip?
A) pip install package-name
B) install pip package-name
C) python install package-name
D) pip package-name install
Answer: A) pip install package-name
3. What is the primary purpose of the gmplot library in Python?
A) To create 3D plots
B) To plot data on Google Maps
C) To generate matplotlib graphs
D) To create dashboards
Answer: B) To plot data on Google Maps
4. In a game of Snakes and Ladders, a player is currently on square 96. There is a snake on square 99 that sends the player back to square 78. If the player wishes to reach square 100 in one dice throw, what number must they roll on the dice?
Answer: 4
5. In the same scenario, where the player is on square 96 and needs to roll a 4 to reach square 100, what is the probability of rolling this number on a fair six-sided die?
A) 1/2
B) 1/3
C) 1/4
D) 1/6
Answer: D) 1/6
These are The Joy of Computing Using Python NPTEL Week 7 Answers
6. Which of the following commands will draw a square using Python’s turtle module?
Turtle is imported in the following way:
import turtle as t
turtle = t.Turtle()
A) for i in range(4):
turtle.forward(100)
turtle.left(90)
B) for i in range(4):
turtle.forward(100)
turtle.right(90)
C) for i in range(4):
turtle.backward(100)
turtle.left(90)
D) for i in range(4):
turtle.backward(100)
turtle.right(90)
Answer: A) for i in range(4):
turtle.forward(100)
turtle.left(90)
B) for i in range(4):
turtle.forward(100)
turtle.right(90)
C) for i in range(4):
turtle.backward(100)
turtle.left(90)
D) for i in range(4):
turtle.backward(100)
turtle.right(90)
7. Does the turtle module in Python allow you to draw complex shapes on the screen?
A) Yes
B) No
Answer: A) Yes
8. What is the purpose of the turtle.penup()
and turtle.pendown()
commands in Python’s turtle module?
A) To stop the turtle from drawing and then resume drawing at a new position
B) To change the color of the turtle’s pen
C) To speed up or slow down the drawing speed of the turtle
D) To reset the turtle’s position
Answer: A) To stop the turtle from drawing and then resume drawing at a new position
9. What is the default drawing state of the turtle when a new turtle object is created in Python’s turtle module?
A) The turtle’s pen is up, so it does not draw while moving.
B) The turtle’s pen is down, so it draws while moving.
C) The turtle is hidden, so it does not appear on the screen.
D) The turtle starts with a circular shape.
Answer: B) The turtle’s pen is down, so it draws while moving.
10. Which of the following commands is used to open an image file using Python’s PIL (Pillow) library?
A) img = PIL.Image.open(’image.jpg’)
B) img = Image.open(’image.jpg’)
C) img = open_image(’image.jpg’)
D) img = PIL.open_image(’image.jpg’)
Answer: A) img = PIL.Image.open(’image.jpg’)
These are The Joy of Computing Using Python NPTEL Week 7 Answers
All Weeks of The Joy of Computing Using Python: Click here
For answers to additional Nptel courses, please refer to this link: NPTEL Assignment Answers
The Joy of Computing Using Python NPTEL Week 7 Answers(JAN-APR 2024)
Course Name: The Joy of Computing using Python
Course Link: Click Here
For answers or latest updates join our telegram channel: Click here to join
These are the Joy of Computing using Python Assignment 7 Answers
Q1. The Chaupar game, also known as Pachisi, involves a player moving their pieces around a cross-shaped board based on the throw of dice (or cowrie shells). The objective is to reach the centre of the board. The steps required to implement the game are given below
Arrange them in correct order of implementation. The answer should be a sequence with the step numbers (Example :132)
1. Roll the dice (or simulate the throw of cowrie shells) to determine the number of steps the player can take. Move the player’s piece based on the dice roll. Check if the new position is within the board limits. If it is, proceed; otherwise, stay at the current position.
2. Create a cross-shaped board with four arms, each having three columns of eight squares. Initialize the player’s position to be inside the board at position 0..Define the centre of the board (position 24) as the goal.
3. Check if the player has reached or exceeded the centre of the board (position 24). If the player has reached the centre, declare them the winner. If not, proceed to the next players turn and roll the die again and repeat the steps for each player
Answer: 213
Q2. Given Below is a simple algorithm for a Snake and Ladder game
I)Create a board with positions numbered from 1 to 100. Define the positions of snakes and ladders on the board. Each snake or ladder connects two positions.
II) Roll the dice to get a random number between 1 and 6. Move the player’s piece forward by the number obtained from the dice roll.
Check if the new position contains the head of a snake or the bottom of a ladder. If it does, move the player to the corresponding position as per the snake or ladder.
III)Check if the player has reached or exceeded position 100. If the player has reached or exceeded 100 declare them the winner. If not, proceed to the next player’s turn. Repeat the process for each player’s turn until a player wins.
The following variations were suggested for the algorithm.
A. If we are using a octahedral dice instead of a normal die, Step II) has to be changed to generate a random number between 1 and 8
B. Modification is required only in step I) if we use a board consisting of 200 tiles instead of 100
C. Modification is required in both steps I) and III) if we use a board consisting of 200 tiles instead of 100
Identify the correct Statements
A and B
Only B
A and C
Only C
Answer: A and C
For answers or latest updates join our telegram channel: Click here to join
These are the Joy of Computing using Python Assignment 7 Answers
Q3. Nishan enjoys the game of snake and ladders but feels that the player should be given an extra chance if the player lands on a prime numbered tile (Assume that no prime-numbered tile has a snake or ladder in it).
He generates the following function is_prime(position) to check if the position of the player is prime or not. However, there is a chance that there could be a small error in the code. Identify the line number corresponding to the error, in the absence of an error answer -1
Answer: 2
Q4. Find the error in the line of code written using the library turtle to draw a square. In the absence of an error enter the answer as -1.
Answer: 7
For answers or latest updates join our telegram channel: Click here to join
These are the Joy of Computing using Python Assignment 7 Answers
Q5. Like the spiral pattern, the matrix can be printed in many patterns and forms. In the snake pattern, the elements of the row are first printed from left to right and then the elements of the next row are printed from right to left and so on.
A crucial step in the algorithm to print the snake pattern is to check the row parity (remainder when the row number is divided by 2). Identify the correct statements regarding this step. (Assume the normal row indexing in a matrix starting from 0) [MSQ]
When parity is 0, we print the elements of the row from left to right
When parity is 1, we print the elements of the row from left to right
When parity is 0, we print the elements of the row from right to left
When parity is 1, we print the elements of the row from right to left
Answer: a), d)
Q6. Which of these conditions guarantee that the element is a boundary element of the matrix of order m by n? Take i as the row index and j as the column index of the element of to be checked [MSQ]
i==0
i== m-1
j==0
j==n-1
Answer: a), b), c), d)
For answers or latest updates join our telegram channel: Click here to join
These are the Joy of Computing using Python Assignment 7 Answers
Q7. Given a set of 4 integers as input, the following code tries to print the elements in the form of a square matrix of order 2 . But the code might contain errors. Identify the line number corresponding to the error, In the absence of an error give your answer as -1
Answer: -1
Q8. How can you write data to a CSV file using the csv
module?
write_csv_file()
csv.writer()
csv.write()
write_csv()
Answer: csv.writer()
For answers or latest updates join our telegram channel: Click here to join
These are the Joy of Computing using Python Assignment 7 Answers
Q9. Which object is commonly used for reading rows from a CSV file in Python?
‘csv.parser’
‘csv.handler’
‘csv.reader’
‘csv.iterator’
Answer: ‘csv.reader’
Q10. Identify the correct statement(s) regarding the gmplot library
I) gmplot is a Python library that allows you to plot data on Google Maps.
II) The syntax for creating a base map with gmplot is
gmplot.GoogleMapPlotter(latitude, longitude, zoom)
the parameters are the geographical coordinates i.e., Latitude and Longitude and the zoom resolution
I only
II only
Both I and II
Neither I nor II
Answer: Both I and II
For answers or latest updates join our telegram channel: Click here to join
These are the Joy of Computing using Python Assignment 7 Answers
The Joy of Computing using Python Programming Assignment
Question 1
In spreadsheets, columns are labelled as follows:
A is the first column, B is the second column, Z is the 26th column, and so on. Using the table given above, deduce the mapping between column labels and their corresponding numbers. Accept the column label as input and print the corresponding column number as output.
Solution:
def letter_value(letter):
return ord(letter) - ord('A') + 1
def string_value(s):
n = len(s)
value = 0
for i in range(n):
value += letter_value(s[i]) * (26 ** (n - i - 1))
return value
code = input().upper()
print(string_value(code),end="")
For answers or latest updates join our telegram channel: Click here to join
These are the Joy of Computing using Python Assignment 7 Answers
Question 2
A queue at a fast-food restaurant operates in the following manner. The queue is a single line with its head at the left-end and tail at the right-end. A person can exit the queue only at the head (left) and join the queue only at the tail (right). At the point of entry, each person is assigned a unique token.
You have been asked to manage the queue. The following operations are permitted on it:
The queue is empty to begin with. Keep accepting an operation as input from the user until the string “END” is entered.
Solution:
class Queue:
def __init__(self):
self.queue = []
def join(self, token):
self.queue.append(token)
def leave(self):
if self.queue:
self.queue.pop(0)
def move(self, token, position):
if token in self.queue:
self.queue.remove(token)
if position.upper() == 'HEAD':
self.queue.insert(0, token)
elif position.upper() == 'TAIL':
self.queue.append(token)
def print_queue(self):
print(",".join(map(str, self.queue)))
def main():
q = Queue()
while True:
operation = input().split(",")
if operation[0].upper() == "END":
break
elif operation[0].upper() == "JOIN":
q.join(operation[1])
elif operation[0].upper() == "LEAVE":
q.leave()
elif operation[0].upper() == "MOVE":
q.move(operation[1], operation[2])
elif operation[0].upper() == "PRINT":
q.print_queue()
chalo=main()
For answers or latest updates join our telegram channel: Click here to join
These are the Joy of Computing using Python Assignment 7 Answers
Question 3
Accept a string of lowercase letters from the user and encrypt it using the following image:
Each letter in the string that appears in the upper half should be replaced with the corresponding letter in the lower half and vice versa. Print the encrypted string as output.
Solution:
letter_map = {
'a': 'z', 'b': 'y', 'c': 'x', 'd': 'w', 'e': 'v', 'f': 'u', 'g': 't', 'h': 's', 'i': 'r', 'j': 'q', 'k': 'p', 'l': 'o', 'm': 'n', 'n': 'm', 'o': 'l', 'p': 'k', 'q': 'j', 'r': 'i', 's': 'h', 't': 'g', 'u': 'f', 'v': 'e', 'w': 'd', 'x': 'c', 'y': 'b', 'z': 'a'
}
def encrypt_message(message):
encrypted_message = ""
for char in message:
if char.isalpha():
encrypted_message += letter_map[char]
else:
encrypted_message += char
return encrypted_message
message = input()
encrypted_message = encrypt_message(message)
print( encrypted_message,end="")
For answers or latest updates join our telegram channel: Click here to join
These are the Joy of Computing using Python Assignment 7 Answers
More Weeks of The Joy of Computing Using Python: Click here
More Nptel Courses: Click here
The Joy of Computing Using Python NPTEL Week 7 Answers (July-Dec 2023)
Course Name: The Joy of Computing using Python
Course Link: Click Here
These are answers of The Joy of Computing using Python Assignment 7 Answers
Q1. Values of CSV files are separated by?
Commas
Colons
Semi-colons
Slash
Answer: Commas
Q2. What is the output of the following code?
1, 2, 3, 7, 11, 10, 9, 5, 6
1, 2, 3, 5, 6, 7, 9, 10, 11
1, 5, 9, 10, 11, 7, 3, 2, 6
1, 5, 9, 2, 6, 10, 3, 7, 11
Answer: 1, 5, 9, 10, 11, 7, 3, 2, 6
These are answers of The Joy of Computing using Python Assignment 7 Answers