Programming in Modern C++ Week 6 Assignment Answers
Are you looking for the NPTEL Programming in Modern C++ Week 6 Assignment Answers? You’ve come to the right place! This resource provides comprehensive solutions to all the questions from the Week 6 assignment, helping you navigate through the complexities of modern C++ programming.
Course Link: Click Here
Table of Contents
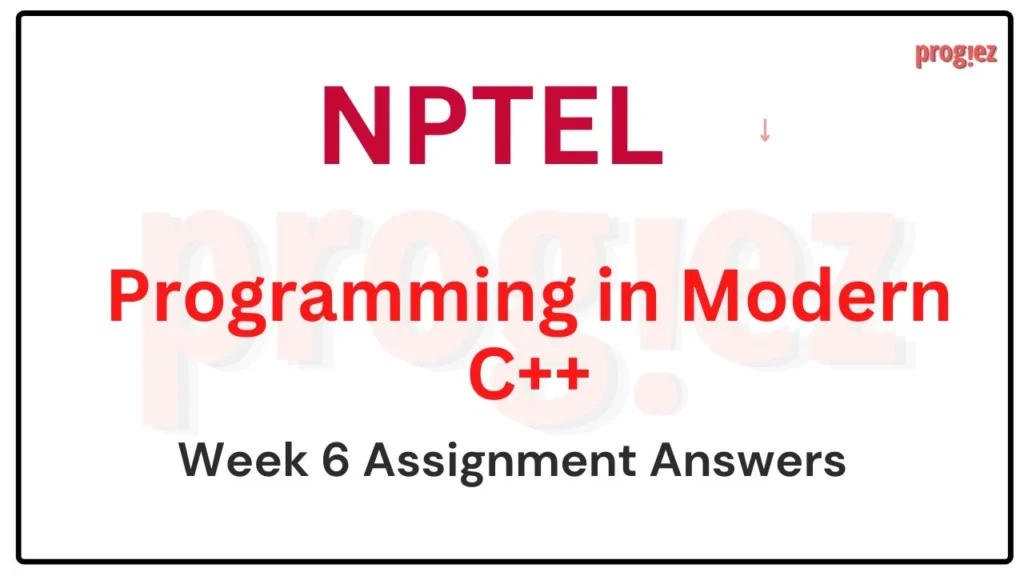
Programming in Modern C++ Week 6 Assignment Answers (July-Dec 2024)
Q1.Which line/s will give error?
a) AC
b) AD
c) BC
a) BD
Answer: b) AD
Q2 Consider the following code segment.
What will be the output /error?
a) Mammal Labrador
b) Mammal 0
c) Compilation error at LINE-1: class Dog has no member named ’displayType’
d) Compilation error at LINE-2: class Animal has no member named ’displayBreed’
Answer: d) Compilation error at LINE-2: class Animal has no member named ’displayBreed’
For answers or latest updates join our telegram channel: Click here to join
Q3.Consider the following code segment.
What will be the output?
a) XYZ ~Z~Y ~X
b) XYZ~ Z ~Y
c) XYZ ~Y ~X
d) XYZ ~X
Answer: d) XYZ ~X
Q4.Consider the following code segment.
Fill in the blank at LINE-1 such that the program runs successfully and prints display ()
a) Base: :display();
b) using Base: :display;
c) Base.display();
d) void display();
Answer: b) using Base: :display;
For answers or latest updates join our telegram channel: Click here to join
Q5.Consider the following code segment.
‘What will be the output?
a) B1 B2 B1 F D1 D2 D1
b) B1 B2 F D1 D2 D1
c) B1 B2 F D2 D1
d) BL B2 F D2
Answer: b) B1 B2 F D1 D2 D1
Q6. Consider the following code segment.
Fill in the blank at LINE-1 such that the program gives output as
Circle: :draw
a) void draw() = 0
b) virtual void draw()
c) virtual void draw() = 0
d) void draw()
Answer: c) virtual void draw() = 0
For answers or latest updates join our telegram channel: Click here to join
Q7. Consider the following code segment.
‘What will be the output?
a) 0
b) 3
c) 4
d) Garbage
Answer: a) 0
Q8. Consider the following code segment.
What will be the output?
a) X::display()
b) Y::display()
c) Z::display()
d) Compilation error: cannot call member function of class ‘Y’
Answer: b) Y::display()
For answers or latest updates join our telegram channel: Click here to join
Q9.Consider the following code segment.
What will be the output /error?
a) Base2::show()Base2::display()Basei::printBase2: :show()Base2: :display()Basei::print
b) Base2::show()Basei::display()Base2: :printBase2: :show()Base2: :display()Basei: :print
c) Base2::show()Base2: :display()Basel::printBase2: :show()Basel::display()Base2: :print
d) compile time error
Answer: a) Base2::show()Base2::display()Basei::printBase2: :show()Base2: :display()Basei::print
For answers or latest updates join our telegram channel: Click here to join
For answers to additional Nptel courses, please refer to this link: NPTEL Assignment
Programming in Modern C++ Week 6 Assignment Answers (JAN-APR 2024)
Course Name: Programming in Modern C++
Course Link: Click Here
For answers or latest updates join our telegram channel: Click here to join
These are Nptel Programming in Modern C++ Assignment 6 Answers
Quiz
Q1. Consider the following code segment.
What will be the output?
a) A1B1
b) A1B2
c) A2B1
d) A2B2
Answer: b) A1B2
Q2. Consider the following code segment.
What will be the output?
a) 8 8 1
b) 8 8 4
c) 1 8 4
d) 0 1 4
Answer: b) 8 8 4
Q3. Consider the following code segment.
What will be the output?
a) A B C ~C ~B ~A
b) A B C ~C ~B
c) A B C ~B ~A
d) A B C ~A
Answer: d) A B C ~A
For answers or latest updates join our telegram channel: Click here to join
These are Nptel Programming in Modern C++ Assignment 6 Answers
Q4. Consider the following code segment.
Which line/s will NOT give you error?
a) LINE-1
b) LINE-2
c) LINE-3
d) LINE-4
Answer: a) LINE-1
Q5. Consider the following code segment.
Which line/s will give you error?
a) LINE-1
b) LINE-2
c) LINE-3
d) LINE-4
Answer: a), c)
Q6. Consider the following code segment.
Fill in the blank at LINE-1 such that the program will print Class2: : fun().
a) new Class1
b) new Class2
c) new Class3
d) It cannot be printed
Answer: b) new Class2
For answers or latest updates join our telegram channel: Click here to join
These are Nptel Programming in Modern C++ Assignment 6 Answers
Q7. Consider the following code segment.
What will be the output?
a) 0
b) 1
c) 2
d) Garbage values
Answer: a) 0
Q8. Consider the following code segment.
Fill in the blank at LINE-1 so that the program will print 1.
a) cb-›f()
b) classA: : cb->f (
c) cb->classA: :f ()
d) classA: :f ()
Answer: c) cb->classA: :f ()
Q9. Identify the abstract class/es from the following code snippet.
a) Flower, FlowerWSmell, FlowerWOSmell
b) Flower, FlowerWOSmell, Dahlia
c) Flower, FlowerWSmell, FlowerWOSmell, Sunflower
d) Flower
Answer: b) Flower, FlowerWOSmell, Dahlia
For answers or latest updates join our telegram channel: Click here to join
These are Nptel Programming in Modern C++ Assignment 6 Answers
Programming Assignment
Question 1
Complete the program with the following instructions.
• Fill in the blank at LINE-1 with appropriate keyword,
• Fill in the blanks at LINE-2 to declare fun () as a pure virtual function,
• fill in the blank at LINE-3 with approriate object instantiation,
The program must satisfy the given test cases.
Solution:
#include<iostream>
using namespace std;
class Base{
protected:
int s;
public:
Base(int i=0) : s(i){}
virtual ~Base(){ }
virtual int fun() = 0;
};
class Derived : public Base{
int x;
public:
Derived(int i, int j) : Base(i), x(j) {}
~Derived();
int fun(){
return s*x;
}
};
class Wrapper{
public:
void fun(int a, int b){
Base *t = new Derived(a, b);
int i = t->fun();
cout << i << " ";
delete t;
}
};
For answers or latest updates join our telegram channel: Click here to join
These are Nptel Programming in Modern C++ Assignment 6 Answers
Question 2
Consider the following program with the following instructions.
• Fill in the blank at LINE-1 and LINE-2 with appropriate return statements
The program must satisfy the sample input and output.
Solution:
double Customer::getMaturity(){
return principal + getInterest();
}
double Employee::getMaturity(){
return principal + getInterest() + getExtraAmt();
}
For answers or latest updates join our telegram channel: Click here to join
These are Nptel Programming in Modern C++ Assignment 6 Answers
Question 3
Consider the following program. Fill in the blanks as per the instructions given below :
• Fill in the blank at LINE-1 with an appropriate destructor declaration.
• Fill in the blank at LINE-2 with an appropriate constructor statement.
such that it will satisfy the given test cases.
Solution:
#include<iostream>
using namespace std;
class A{
public:
A(){ cout << "11 "; }
A(int n){ cout << n + 2 << " "; }
virtual ~A();
};
class B : public A{
public:
B(int n) : A(n)
{
cout << n + 5 << " ";
}
B(){ cout << "21 "; }
virtual ~B(){ cout << "22 "; }
};
For answers or latest updates join our telegram channel: Click here to join
These are Nptel Programming in Modern C++ Assignment 6 Answers
More Weeks of Programming in Modern C++: Click here
More Nptel Courses: Click here