3373. Maximize the Number of Target Nodes After Connecting Trees II LeetCode Solution
In this guide, you will get 3373. Maximize the Number of Target Nodes After Connecting Trees II LeetCode Solution with the best time and space complexity. The solution to Maximize the Number of Target Nodes After Connecting Trees II problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Maximize the Number of Target Nodes After Connecting Trees II solution in C++
- Maximize the Number of Target Nodes After Connecting Trees II solution in Java
- Maximize the Number of Target Nodes After Connecting Trees II solution in Python
- Additional Resources
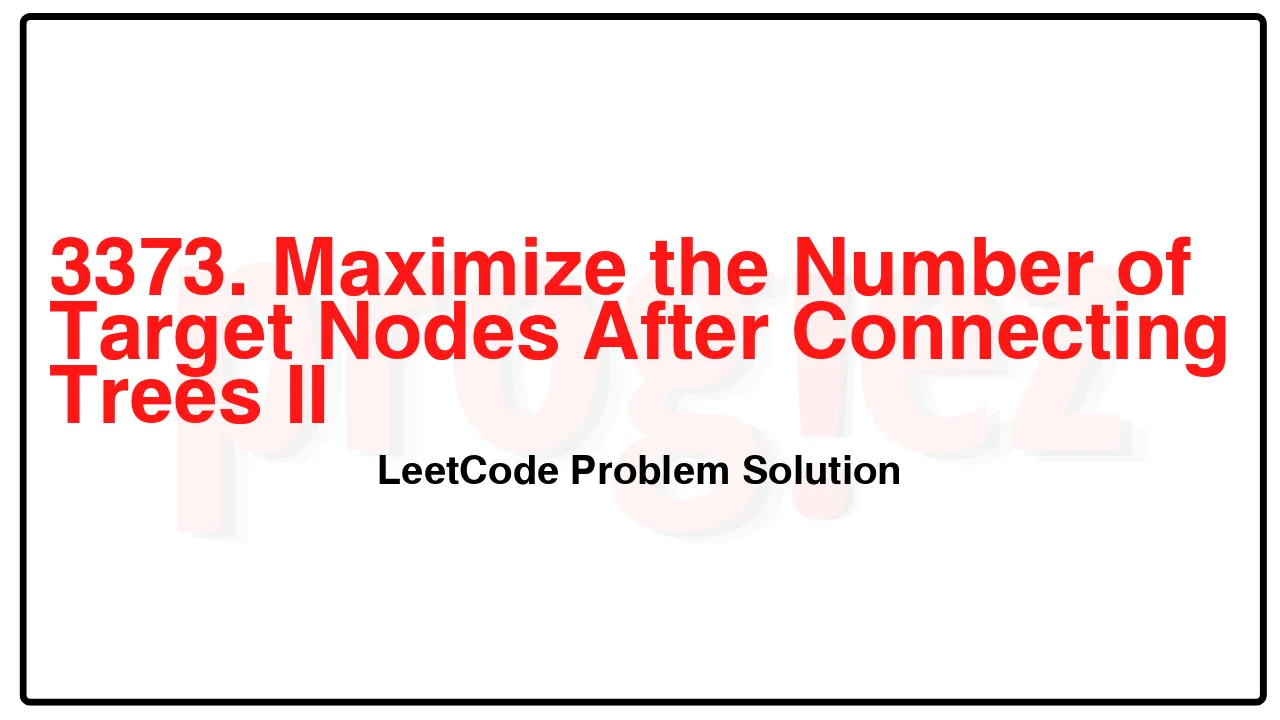
Problem Statement of Maximize the Number of Target Nodes After Connecting Trees II
There exist two undirected trees with n and m nodes, labeled from [0, n – 1] and [0, m – 1], respectively.
You are given two 2D integer arrays edges1 and edges2 of lengths n – 1 and m – 1, respectively, where edges1[i] = [ai, bi] indicates that there is an edge between nodes ai and bi in the first tree and edges2[i] = [ui, vi] indicates that there is an edge between nodes ui and vi in the second tree.
Node u is target to node v if the number of edges on the path from u to v is even. Note that a node is always target to itself.
Return an array of n integers answer, where answer[i] is the maximum possible number of nodes that are target to node i of the first tree if you had to connect one node from the first tree to another node in the second tree.
Note that queries are independent from each other. That is, for every query you will remove the added edge before proceeding to the next query.
Example 1:
Input: edges1 = [[0,1],[0,2],[2,3],[2,4]], edges2 = [[0,1],[0,2],[0,3],[2,7],[1,4],[4,5],[4,6]]
Output: [8,7,7,8,8]
Explanation:
For i = 0, connect node 0 from the first tree to node 0 from the second tree.
For i = 1, connect node 1 from the first tree to node 4 from the second tree.
For i = 2, connect node 2 from the first tree to node 7 from the second tree.
For i = 3, connect node 3 from the first tree to node 0 from the second tree.
For i = 4, connect node 4 from the first tree to node 4 from the second tree.
Example 2:
Input: edges1 = [[0,1],[0,2],[0,3],[0,4]], edges2 = [[0,1],[1,2],[2,3]]
Output: [3,6,6,6,6]
Explanation:
For every i, connect node i of the first tree with any node of the second tree.
Constraints:
2 <= n, m <= 105
edges1.length == n – 1
edges2.length == m – 1
edges1[i].length == edges2[i].length == 2
edges1[i] = [ai, bi]
0 <= ai, bi < n
edges2[i] = [ui, vi]
0 <= ui, vi < m
The input is generated such that edges1 and edges2 represent valid trees.
Complexity Analysis
- Time Complexity: O(n + m)
- Space Complexity: O(n + m)
3373. Maximize the Number of Target Nodes After Connecting Trees II LeetCode Solution in C++
class Solution {
public:
vector<int> maxTargetNodes(vector<vector<int>>& edges1,
vector<vector<int>>& edges2) {
const int n = edges1.size() + 1;
const int m = edges2.size() + 1;
vector<int> ans;
const vector<vector<int>> graph1 = buildGraph(edges1);
const vector<vector<int>> graph2 = buildGraph(edges2);
vector<bool> parity1(n);
vector<bool> parity2(m); // placeholder (parity2 is not used)
const int even1 = dfs(graph1, 0, -1, parity1, /*isEven=*/true);
const int even2 = dfs(graph2, 0, -1, parity2, /*isEven=*/true);
const int odd1 = n - even1;
const int odd2 = m - even2;
for (int i = 0; i < n; ++i) {
const int tree1 = parity1[i] ? even1 : odd1;
// Can connect the current node in tree1 to either an even node or an odd
// node in tree2.
const int tree2 = max(even2, odd2);
ans.push_back(tree1 + tree2);
}
return ans;
}
private:
// Returns the number of nodes that can be reached from u with even steps.
int dfs(const vector<vector<int>>& graph, int u, int prev,
vector<bool>& parity, bool isEven) {
int res = isEven ? 1 : 0;
parity[u] = isEven;
for (const int v : graph[u])
if (v != prev)
res += dfs(graph, v, u, parity, !isEven);
return res;
}
vector<vector<int>> buildGraph(const vector<vector<int>>& edges) {
vector<vector<int>> graph(edges.size() + 1);
for (const vector<int>& edge : edges) {
const int u = edge[0];
const int v = edge[1];
graph[u].push_back(v);
graph[v].push_back(u);
}
return graph;
}
};
/* code provided by PROGIEZ */
3373. Maximize the Number of Target Nodes After Connecting Trees II LeetCode Solution in Java
class Solution {
public int[] maxTargetNodes(int[][] edges1, int[][] edges2) {
final int n = edges1.length + 1;
final int m = edges2.length + 1;
List<Integer>[] graph1 = buildGraph(edges1, n);
List<Integer>[] graph2 = buildGraph(edges2, m);
boolean[] parity1 = new boolean[n];
boolean[] parity2 = new boolean[m]; // Placeholder (not used)
final int even1 = dfs(graph1, 0, -1, parity1, true);
final int even2 = dfs(graph2, 0, -1, parity2, true);
final int odd1 = n - even1;
final int odd2 = m - even2;
int[] ans = new int[n];
for (int i = 0; i < n; i++) {
final int tree1 = parity1[i] ? even1 : odd1;
// Can connect the current node in tree1 to either an even or an odd node
// in tree2.
final int tree2 = Math.max(even2, odd2);
ans[i] = tree1 + tree2;
}
return ans;
}
// Returns the number of nodes that can be reached from u with even steps.
private int dfs(List<Integer>[] graph, int u, int prev, boolean[] parity, boolean isEven) {
int res = isEven ? 1 : 0;
parity[u] = isEven;
for (final int v : graph[u])
if (v != prev)
res += dfs(graph, v, u, parity, !isEven);
return res;
}
private List<Integer>[] buildGraph(int[][] edges, int n) {
List<Integer>[] graph = new ArrayList[n];
for (int i = 0; i < n; i++)
graph[i] = new ArrayList<>();
for (int[] edge : edges) {
final int u = edge[0];
final int v = edge[1];
graph[u].add(v);
graph[v].add(u);
}
return graph;
}
}
// code provided by PROGIEZ
3373. Maximize the Number of Target Nodes After Connecting Trees II LeetCode Solution in Python
class Solution:
def maxTargetNodes(
self,
edges1: list[list[int]],
edges2: list[list[int]]
) -> list[int]:
n = len(edges1) + 1
m = len(edges2) + 1
graph1 = self._buildGraph(edges1)
graph2 = self._buildGraph(edges2)
parity1 = [False] * n
parity2 = [False] * m # placeholder (parity2 is not used)
even1 = self._dfs(graph1, 0, -1, parity1, True)
even2 = self._dfs(graph2, 0, -1, parity2, True)
odd1 = n - even1
odd2 = m - even2
# Can connect the current node in tree1 to either an even node or an odd
# node in tree2.
return [(even1 if parity1[i] else odd1) + max(even2, odd2)
for i in range(n)]
def _dfs(
self,
graph: list[list[int]],
u: int,
prev: int,
parity: list[bool],
isEven: bool
) -> int:
"""
Returns the number of nodes that can be reached from u with even steps.
"""
res = 1 if isEven else 0
parity[u] = isEven
for v in graph[u]:
if v != prev:
res += self._dfs(graph, v, u, parity, not isEven)
return res
def _buildGraph(self, edges: list[list[int]]) -> list[list[int]]:
graph = [[] for _ in range(len(edges) + 1)]
for u, v in edges:
graph[u].append(v)
graph[v].append(u)
return graph
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.