3372. Maximize the Number of Target Nodes After Connecting Trees I LeetCode Solution
In this guide, you will get 3372. Maximize the Number of Target Nodes After Connecting Trees I LeetCode Solution with the best time and space complexity. The solution to Maximize the Number of Target Nodes After Connecting Trees I problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Maximize the Number of Target Nodes After Connecting Trees I solution in C++
- Maximize the Number of Target Nodes After Connecting Trees I solution in Java
- Maximize the Number of Target Nodes After Connecting Trees I solution in Python
- Additional Resources
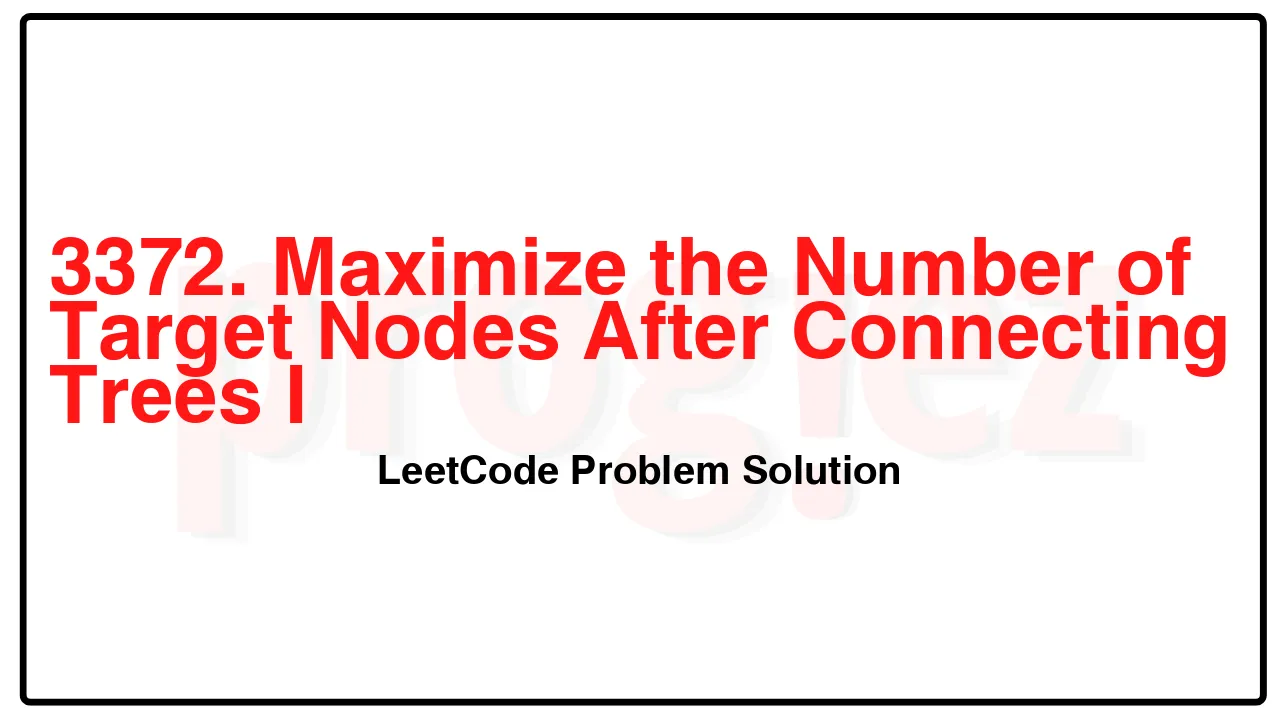
Problem Statement of Maximize the Number of Target Nodes After Connecting Trees I
There exist two undirected trees with n and m nodes, with distinct labels in ranges [0, n – 1] and [0, m – 1], respectively.
You are given two 2D integer arrays edges1 and edges2 of lengths n – 1 and m – 1, respectively, where edges1[i] = [ai, bi] indicates that there is an edge between nodes ai and bi in the first tree and edges2[i] = [ui, vi] indicates that there is an edge between nodes ui and vi in the second tree. You are also given an integer k.
Node u is target to node v if the number of edges on the path from u to v is less than or equal to k. Note that a node is always target to itself.
Return an array of n integers answer, where answer[i] is the maximum possible number of nodes target to node i of the first tree if you have to connect one node from the first tree to another node in the second tree.
Note that queries are independent from each other. That is, for every query you will remove the added edge before proceeding to the next query.
Example 1:
Input: edges1 = [[0,1],[0,2],[2,3],[2,4]], edges2 = [[0,1],[0,2],[0,3],[2,7],[1,4],[4,5],[4,6]], k = 2
Output: [9,7,9,8,8]
Explanation:
For i = 0, connect node 0 from the first tree to node 0 from the second tree.
For i = 1, connect node 1 from the first tree to node 0 from the second tree.
For i = 2, connect node 2 from the first tree to node 4 from the second tree.
For i = 3, connect node 3 from the first tree to node 4 from the second tree.
For i = 4, connect node 4 from the first tree to node 4 from the second tree.
Example 2:
Input: edges1 = [[0,1],[0,2],[0,3],[0,4]], edges2 = [[0,1],[1,2],[2,3]], k = 1
Output: [6,3,3,3,3]
Explanation:
For every i, connect node i of the first tree with any node of the second tree.
Constraints:
2 <= n, m <= 1000
edges1.length == n – 1
edges2.length == m – 1
edges1[i].length == edges2[i].length == 2
edges1[i] = [ai, bi]
0 <= ai, bi < n
edges2[i] = [ui, vi]
0 <= ui, vi < m
The input is generated such that edges1 and edges2 represent valid trees.
0 <= k <= 1000
Complexity Analysis
- Time Complexity: O(k(n + m))
- Space Complexity: O(n + m)
3372. Maximize the Number of Target Nodes After Connecting Trees I LeetCode Solution in C++
class Solution {
public:
vector<int> maxTargetNodes(vector<vector<int>>& edges1,
vector<vector<int>>& edges2, int k) {
vector<int> ans;
const vector<vector<int>> graph1 = buildGraph(edges1);
const vector<vector<int>> graph2 = buildGraph(edges2);
int maxReachableInGraph2 = 0;
if (k > 0)
for (int i = 0; i < edges2.size() + 1; ++i)
maxReachableInGraph2 =
max(maxReachableInGraph2, dfs(graph2, i, -1, k - 1));
for (int i = 0; i < edges1.size() + 1; ++i)
ans.push_back(maxReachableInGraph2 + dfs(graph1, i, -1, k));
return ans;
}
private:
// Returns the number of nodes that can be reached from u with k steps.
int dfs(const vector<vector<int>>& graph, int u, int prev, int k) {
if (k == 0)
return 1;
int res = 0;
for (const int v : graph[u])
if (v != prev)
res += dfs(graph, v, u, k - 1);
return 1 + res;
}
vector<vector<int>> buildGraph(const vector<vector<int>>& edges) {
vector<vector<int>> graph(edges.size() + 1);
for (const vector<int>& edge : edges) {
const int u = edge[0];
const int v = edge[1];
graph[u].push_back(v);
graph[v].push_back(u);
}
return graph;
}
};
/* code provided by PROGIEZ */
3372. Maximize the Number of Target Nodes After Connecting Trees I LeetCode Solution in Java
class Solution {
public int[] maxTargetNodes(int[][] edges1, int[][] edges2, int k) {
int[] ans = new int[edges1.length + 1];
List<Integer>[] graph1 = buildGraph(edges1);
List<Integer>[] graph2 = buildGraph(edges2);
int maxReachableInGraph2 = 0;
if (k > 0)
for (int i = 0; i < edges2.length + 1; ++i)
maxReachableInGraph2 = Math.max(maxReachableInGraph2, dfs(graph2, i, -1, k - 1));
for (int i = 0; i < edges1.length + 1; ++i)
ans[i] = maxReachableInGraph2 + dfs(graph1, i, -1, k);
return ans;
}
// Returns the number of nodes that can be reached from u with k steps.
private int dfs(List<Integer>[] graph, int u, int prev, int k) {
if (k == 0)
return 1;
int res = 0;
for (final int v : graph[u])
if (v != prev)
res += dfs(graph, v, u, k - 1);
return 1 + res;
}
private List<Integer>[] buildGraph(int[][] edges) {
List<Integer>[] graph = new ArrayList[edges.length + 1];
for (int i = 0; i < edges.length + 1; ++i)
graph[i] = new ArrayList<>();
for (int[] edge : edges) {
final int u = edge[0];
final int v = edge[1];
graph[u].add(v);
graph[v].add(u);
}
return graph;
}
}
// code provided by PROGIEZ
3372. Maximize the Number of Target Nodes After Connecting Trees I LeetCode Solution in Python
class Solution:
def maxTargetNodes(
self,
edges1: list[list[int]],
edges2: list[list[int]],
k: int
) -> list[int]:
graph1 = self._buildGraph(edges1)
graph2 = self._buildGraph(edges2)
maxReachableInGraph2 = 0
if k > 0:
for i in range(len(edges2) + 1):
maxReachableInGraph2 = max(maxReachableInGraph2,
self._dfs(graph2, i, -1, k - 1))
return [maxReachableInGraph2 + self._dfs(graph1, i, -1, k)
for i in range(len(edges1) + 1)]
def _dfs(self, graph: list[list[int]], u: int, prev: int, k: int) -> int:
"""Returns the number of nodes that can be reached from u with k steps."""
if k == 0:
return 1
res = 0
for v in graph[u]:
if v != prev:
res += self._dfs(graph, v, u, k - 1)
return 1 + res
def _buildGraph(self, edges: list[list[int]]) -> list[list[int]]:
graph = [[] for _ in range(len(edges) + 1)]
for u, v in edges:
graph[u].append(v)
graph[v].append(u)
return graph
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.