3099. Harshad Number LeetCode Solution
In this guide, you will get 3099. Harshad Number LeetCode Solution with the best time and space complexity. The solution to Harshad Number problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Harshad Number solution in C++
- Harshad Number solution in Java
- Harshad Number solution in Python
- Additional Resources
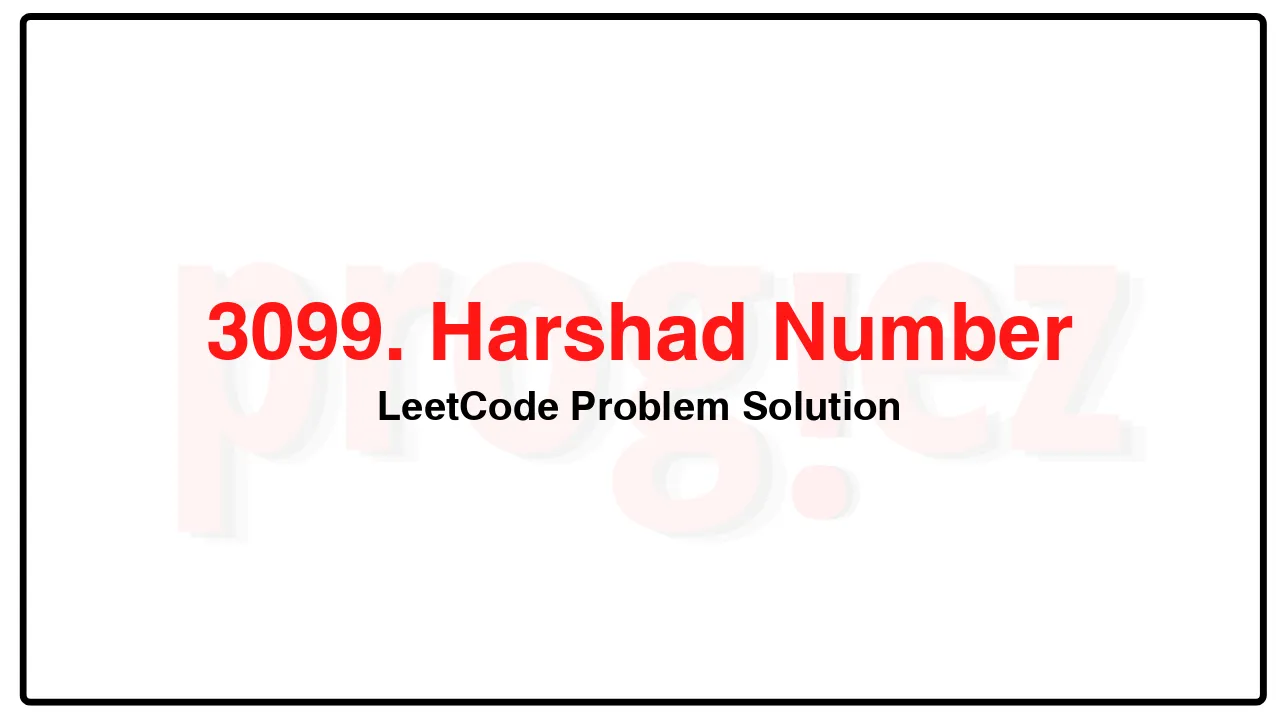
Problem Statement of Harshad Number
An integer divisible by the sum of its digits is said to be a Harshad number. You are given an integer x. Return the sum of the digits of x if x is a Harshad number, otherwise, return -1.
Example 1:
Input: x = 18
Output: 9
Explanation:
The sum of digits of x is 9. 18 is divisible by 9. So 18 is a Harshad number and the answer is 9.
Example 2:
Input: x = 23
Output: -1
Explanation:
The sum of digits of x is 5. 23 is not divisible by 5. So 23 is not a Harshad number and the answer is -1.
Constraints:
1 <= x <= 100
Complexity Analysis
- Time Complexity: O(\log x)
- Space Complexity: O(1)
3099. Harshad Number LeetCode Solution in C++
class Solution {
public:
int sumOfTheDigitsOfHarshadNumber(int x) {
const int digitSum = getDigitSum(x);
return x % digitSum == 0 ? digitSum : -1;
}
private:
int getDigitSum(int x) {
int digitSum = 0;
while (x > 0) {
digitSum += x % 10;
x /= 10;
}
return digitSum;
}
};
/* code provided by PROGIEZ */
3099. Harshad Number LeetCode Solution in Java
class Solution {
public int sumOfTheDigitsOfHarshadNumber(int x) {
final int digitSum = getDigitSum(x);
return x % digitSum == 0 ? digitSum : -1;
}
private int getDigitSum(int x) {
int digitSum = 0;
while (x > 0) {
digitSum += x % 10;
x /= 10;
}
return digitSum;
}
}
// code provided by PROGIEZ
3099. Harshad Number LeetCode Solution in Python
class Solution:
def sumOfTheDigitsOfHarshadNumber(self, x: int) -> int:
digitSum = self._getDigitSum(x)
return digitSum if x % digitSum == 0 else -1
def _getDigitSum(self, num: int) -> int:
return sum(int(digit) for digit in str(num))
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.