2400. Number of Ways to Reach a Position After Exactly k Steps LeetCode Solution
In this guide, you will get 2400. Number of Ways to Reach a Position After Exactly k Steps LeetCode Solution with the best time and space complexity. The solution to Number of Ways to Reach a Position After Exactly k Steps problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Number of Ways to Reach a Position After Exactly k Steps solution in C++
- Number of Ways to Reach a Position After Exactly k Steps solution in Java
- Number of Ways to Reach a Position After Exactly k Steps solution in Python
- Additional Resources
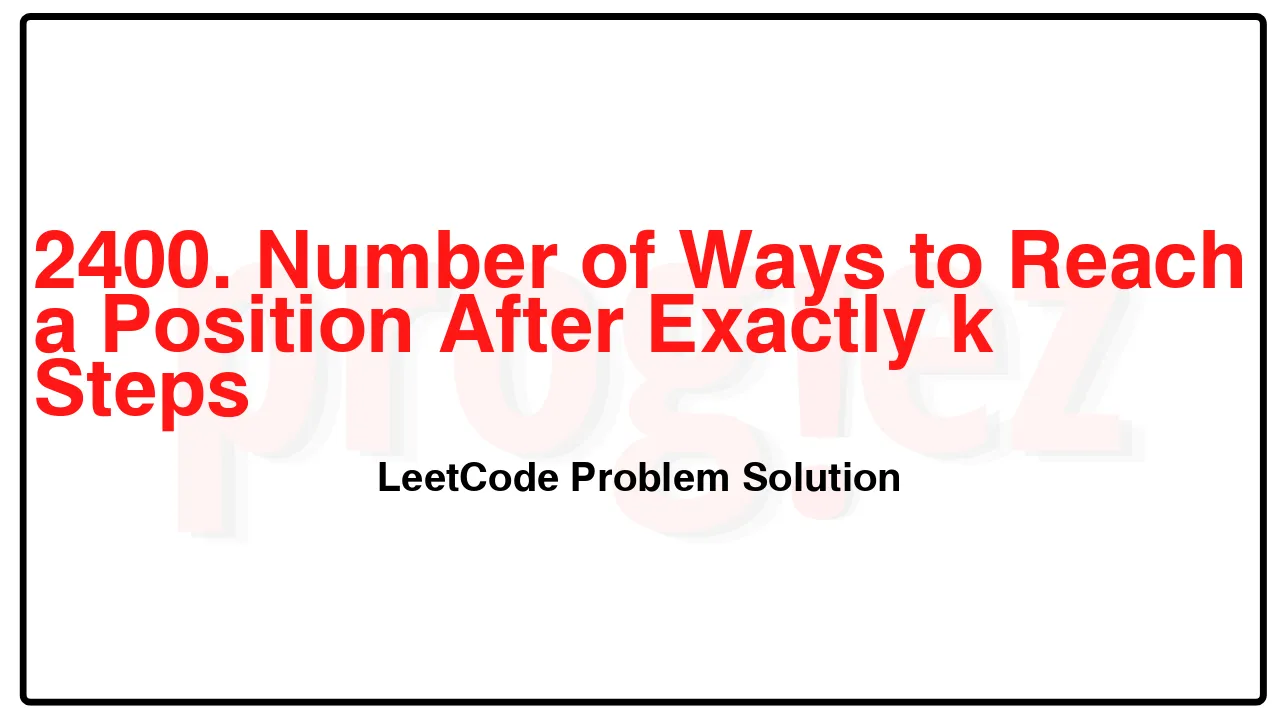
Problem Statement of Number of Ways to Reach a Position After Exactly k Steps
You are given two positive integers startPos and endPos. Initially, you are standing at position startPos on an infinite number line. With one step, you can move either one position to the left, or one position to the right.
Given a positive integer k, return the number of different ways to reach the position endPos starting from startPos, such that you perform exactly k steps. Since the answer may be very large, return it modulo 109 + 7.
Two ways are considered different if the order of the steps made is not exactly the same.
Note that the number line includes negative integers.
Example 1:
Input: startPos = 1, endPos = 2, k = 3
Output: 3
Explanation: We can reach position 2 from 1 in exactly 3 steps in three ways:
– 1 -> 2 -> 3 -> 2.
– 1 -> 2 -> 1 -> 2.
– 1 -> 0 -> 1 -> 2.
It can be proven that no other way is possible, so we return 3.
Example 2:
Input: startPos = 2, endPos = 5, k = 10
Output: 0
Explanation: It is impossible to reach position 5 from position 2 in exactly 10 steps.
Constraints:
1 <= startPos, endPos, k <= 1000
Complexity Analysis
- Time Complexity: O(nk)
- Space Complexity: O(k)
2400. Number of Ways to Reach a Position After Exactly k Steps LeetCode Solution in C++
class Solution {
public:
int numberOfWays(int startPos, int endPos, int k) {
// leftStep + rightStep = k
// rightStep - leftStep = endPos - startPos
// 2 * rightStep = k + endPos - startPos
// rightStep = (k + endPos - startPos) / 2
const int val = k + endPos - startPos;
if (val < 0 || val % 2 == 1)
return 0;
const int rightStep = val / 2;
const int leftStep = k - rightStep;
if (leftStep < 0)
return 0;
return nCk(leftStep + rightStep, min(leftStep, rightStep));
}
private:
static constexpr int kMod = 1'000'000'007;
// C(n, k) = C(n - 1, k) + C(n - 1, k - 1)
int nCk(int n, int k) {
// dp[i] := C(n so far, i)
vector<int> dp(k + 1);
dp[0] = 1;
while (n-- > 0) // Calculate n times.
for (int j = k; j > 0; --j) {
dp[j] += dp[j - 1];
dp[j] %= kMod;
}
return dp[k];
}
};
/* code provided by PROGIEZ */
2400. Number of Ways to Reach a Position After Exactly k Steps LeetCode Solution in Java
class Solution {
public int numberOfWays(int startPos, int endPos, int k) {
// leftStep + rightStep = k
// rightStep - leftStep = endPos - startPos
// 2 * rightStep = k + endPos - startPos
// rightStep = (k + endPos - startPos) / 2
final int val = k + endPos - startPos;
if (val < 0 || val % 2 == 1)
return 0;
final int rightStep = val / 2;
final int leftStep = k - rightStep;
if (leftStep < 0)
return 0;
return nCk(leftStep + rightStep, Math.min(leftStep, rightStep));
}
// C(n, k) = C(n - 1, k) + C(n - 1, k - 1)
private int nCk(int n, int k) {
final int kMod = 1_000_000_007;
// dp[i] := C(n so far, i)
int[] dp = new int[k + 1];
dp[0] = 1;
while (n-- > 0) // Calculate n times.
for (int j = k; j > 0; --j) {
dp[j] += dp[j - 1];
dp[j] %= kMod;
}
return dp[k];
}
}
// code provided by PROGIEZ
2400. Number of Ways to Reach a Position After Exactly k Steps LeetCode Solution in Python
class Solution:
def numberOfWays(self, startPos: int, endPos: int, k: int) -> int:
# leftStep + rightStep = k
# rightStep - leftStep = endPos - startPos
# 2 * rightStep = k + endPos - startPos
# rightStep = (k + endPos - startPos) // 2
val = k + endPos - startPos
if val < 0 or val % 2 == 1:
return 0
rightStep = val // 2
leftStep = k - rightStep
if leftStep < 0:
return 0
return self._nCk(leftStep + rightStep, min(leftStep, rightStep))
# C(n, k) = C(n - 1, k) + C(n - 1, k - 1)
def _nCk(self, n: int, k: int) -> int:
kMod = 1_000_000_007
# dp[i] := C(n so far, i)
dp = [1] + [0] * k
for _ in range(n): # Calculate n times.
for j in range(k, 0, -1):
dp[j] += dp[j - 1]
dp[j] %= kMod
return dp[k]
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.