138. Copy List with Random Pointer LeetCode Solution
In this guide, you will get 138. Copy List with Random Pointer LeetCode Solution with the best time and space complexity. The solution to Copy List with Random Pointer problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Copy List with Random Pointer solution in C++
- Copy List with Random Pointer solution in Java
- Copy List with Random Pointer solution in Python
- Additional Resources
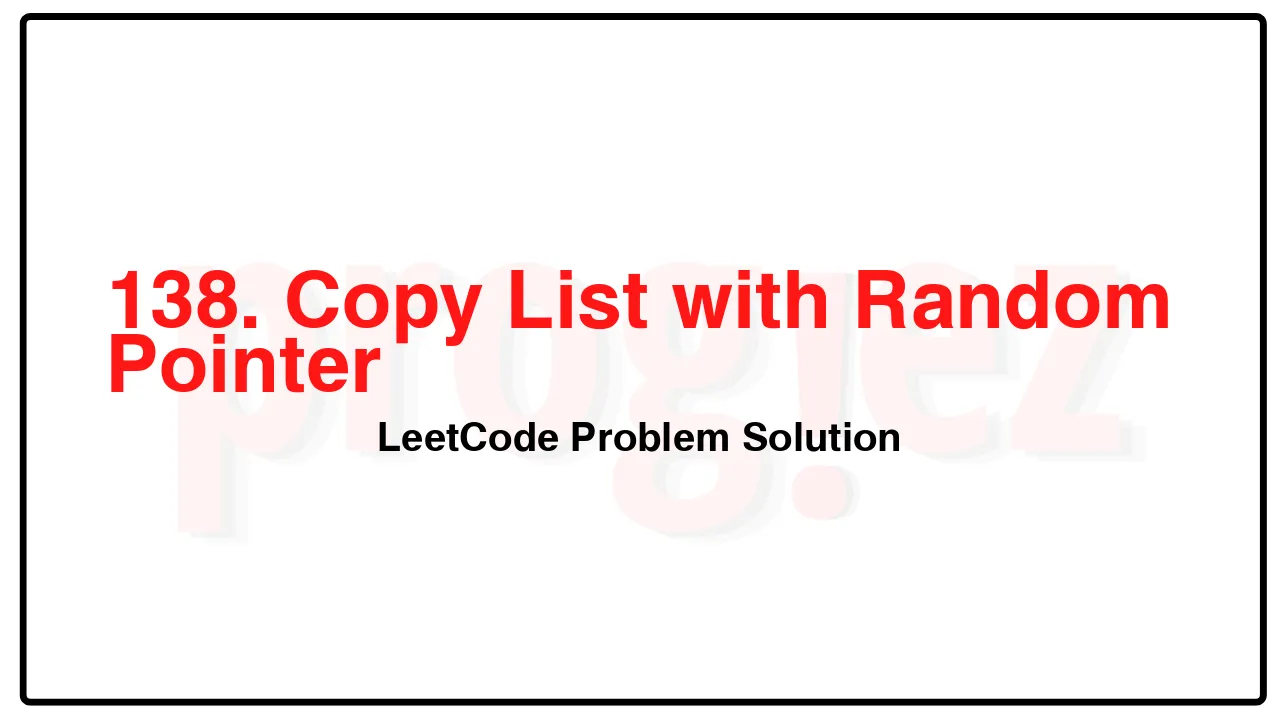
Problem Statement of Copy List with Random Pointer
A linked list of length n is given such that each node contains an additional random pointer, which could point to any node in the list, or null.
Construct a deep copy of the list. The deep copy should consist of exactly n brand new nodes, where each new node has its value set to the value of its corresponding original node. Both the next and random pointer of the new nodes should point to new nodes in the copied list such that the pointers in the original list and copied list represent the same list state. None of the pointers in the new list should point to nodes in the original list.
For example, if there are two nodes X and Y in the original list, where X.random –> Y, then for the corresponding two nodes x and y in the copied list, x.random –> y.
Return the head of the copied linked list.
The linked list is represented in the input/output as a list of n nodes. Each node is represented as a pair of [val, random_index] where:
val: an integer representing Node.val
random_index: the index of the node (range from 0 to n-1) that the random pointer points to, or null if it does not point to any node.
Your code will only be given the head of the original linked list.
Example 1:
Input: head = [[7,null],[13,0],[11,4],[10,2],[1,0]]
Output: [[7,null],[13,0],[11,4],[10,2],[1,0]]
Example 2:
Input: head = [[1,1],[2,1]]
Output: [[1,1],[2,1]]
Example 3:
Input: head = [[3,null],[3,0],[3,null]]
Output: [[3,null],[3,0],[3,null]]
Constraints:
0 <= n <= 1000
-104 <= Node.val <= 104
Node.random is null or is pointing to some node in the linked list.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
138. Copy List with Random Pointer LeetCode Solution in C++
class Solution {
public:
Node* copyRandomList(Node* head) {
if (head == nullptr)
return nullptr;
if (const auto it = map.find(head); it != map.cend())
return it->second;
Node* newNode = new Node(head->val);
map[head] = newNode;
newNode->next = copyRandomList(head->next);
newNode->random = copyRandomList(head->random);
return newNode;
}
private:
unordered_map<Node*, Node*> map;
};
/* code provided by PROGIEZ */
138. Copy List with Random Pointer LeetCode Solution in Java
class Solution {
public Node copyRandomList(Node head) {
if (head == null)
return null;
if (map.containsKey(head))
return map.get(head);
Node newNode = new Node(head.val);
map.put(head, newNode);
newNode.next = copyRandomList(head.next);
newNode.random = copyRandomList(head.random);
return newNode;
}
private Map<Node, Node> map = new HashMap<>();
}
// code provided by PROGIEZ
138. Copy List with Random Pointer LeetCode Solution in Python
class Solution:
def copyRandomList(self, head: 'Node') -> 'Node':
if not head:
return None
if head in self.map:
return self.map[head]
newNode = Node(head.val)
self.map[head] = newNode
newNode.next = self.copyRandomList(head.next)
newNode.random = self.copyRandomList(head.random)
return newNode
map = {}
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.