108. Convert Sorted Array to Binary Search Tree LeetCode Solution
In this guide, you will get 108. Convert Sorted Array to Binary Search Tree LeetCode Solution with the best time and space complexity. The solution to Convert Sorted Array to Binary Search Tree problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Convert Sorted Array to Binary Search Tree solution in C++
- Convert Sorted Array to Binary Search Tree solution in Java
- Convert Sorted Array to Binary Search Tree solution in Python
- Additional Resources
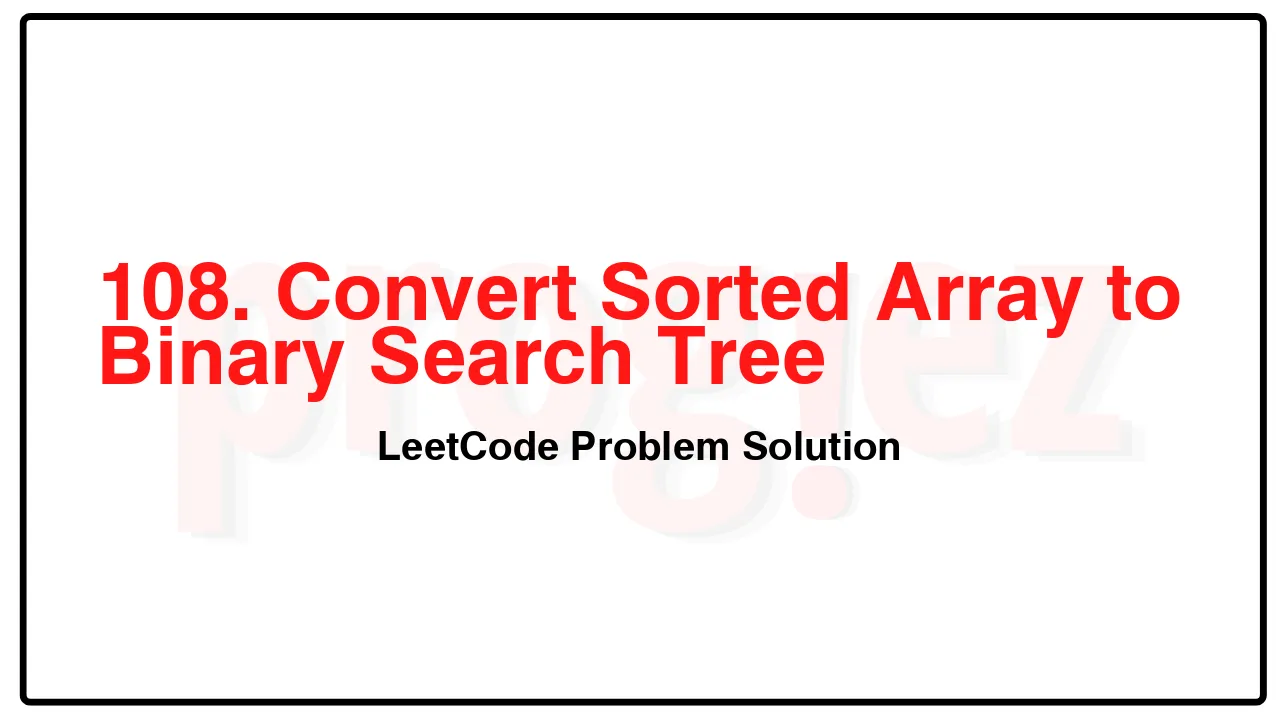
Problem Statement of Convert Sorted Array to Binary Search Tree
Given an integer array nums where the elements are sorted in ascending order, convert it to a height-balanced binary search tree.
Example 1:
Input: nums = [-10,-3,0,5,9]
Output: [0,-3,9,-10,null,5]
Explanation: [0,-10,5,null,-3,null,9] is also accepted:
Example 2:
Input: nums = [1,3]
Output: [3,1]
Explanation: [1,null,3] and [3,1] are both height-balanced BSTs.
Constraints:
1 <= nums.length <= 104
-104 <= nums[i] <= 104
nums is sorted in a strictly increasing order.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(\log n)
108. Convert Sorted Array to Binary Search Tree LeetCode Solution in C++
class Solution {
public:
TreeNode* sortedArrayToBST(vector<int>& nums) {
return build(nums, 0, nums.size() - 1);
}
private:
TreeNode* build(const vector<int>& nums, int l, int r) {
if (l > r)
return nullptr;
const int m = (l + r) / 2;
return new TreeNode(nums[m], build(nums, l, m - 1), build(nums, m + 1, r));
}
};
/* code provided by PROGIEZ */
108. Convert Sorted Array to Binary Search Tree LeetCode Solution in Java
class Solution {
public TreeNode sortedArrayToBST(int[] nums) {
return build(nums, 0, nums.length - 1);
}
private TreeNode build(int[] nums, int l, int r) {
if (l > r)
return null;
final int m = (l + r) / 2;
return new TreeNode(nums[m], build(nums, l, m - 1), build(nums, m + 1, r));
}
}
// code provided by PROGIEZ
108. Convert Sorted Array to Binary Search Tree LeetCode Solution in Python
class Solution:
def sortedArrayToBST(self, nums: list[int]) -> TreeNode | None:
def build(l: int, r: int) -> TreeNode | None:
if l > r:
return None
m = (l + r) // 2
return TreeNode(nums[m],
build(l, m - 1),
build(m + 1, r))
return build(0, len(nums) - 1)
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.