93. Restore IP Addresses LeetCode Solution
In this guide, you will get 93. Restore IP Addresses LeetCode Solution with the best time and space complexity. The solution to Restore IP Addresses problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Restore IP Addresses solution in C++
- Restore IP Addresses solution in Java
- Restore IP Addresses solution in Python
- Additional Resources
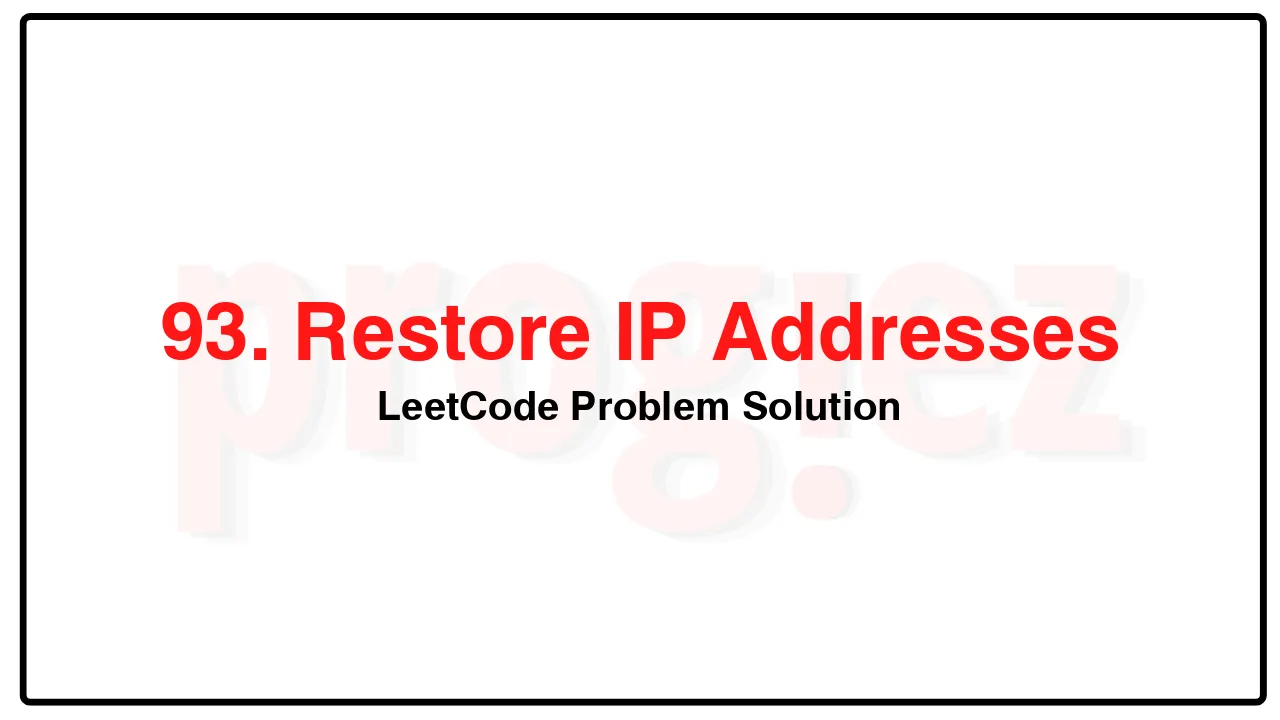
Problem Statement of Restore IP Addresses
A valid IP address consists of exactly four integers separated by single dots. Each integer is between 0 and 255 (inclusive) and cannot have leading zeros.
For example, “0.1.2.201” and “192.168.1.1” are valid IP addresses, but “0.011.255.245”, “192.168.1.312” and “192.168@1.1” are invalid IP addresses.
Given a string s containing only digits, return all possible valid IP addresses that can be formed by inserting dots into s. You are not allowed to reorder or remove any digits in s. You may return the valid IP addresses in any order.
Example 1:
Input: s = “25525511135”
Output: [“255.255.11.135″,”255.255.111.35”]
Example 2:
Input: s = “0000”
Output: [“0.0.0.0”]
Example 3:
Input: s = “101023”
Output: [“1.0.10.23″,”1.0.102.3″,”10.1.0.23″,”10.10.2.3″,”101.0.2.3”]
Constraints:
1 <= s.length <= 20
s consists of digits only.
Complexity Analysis
- Time Complexity: O(3^4)
- Space Complexity: O(1)
93. Restore IP Addresses LeetCode Solution in C++
class Solution {
public:
vector<string> restoreIpAddresses(const string& s) {
vector<string> ans;
dfs(s, 0, {}, ans);
return ans;
}
private:
void dfs(const string& s, int start, vector<string>&& path,
vector<string>& ans) {
if (path.size() == 4 && start == s.length()) {
ans.push_back(path[0] + "." + path[1] + "." + path[2] + "." + path[3]);
return;
}
if (path.size() == 4 || start == s.length())
return;
for (int length = 1; length <= 3; ++length) {
if (start + length > s.length())
return; // out-of-bounds
if (length > 1 && s[start] == '0')
return; // leading '0'
const string& num = s.substr(start, length);
if (stoi(num) > 255)
return;
path.push_back(num);
dfs(s, start + length, std::move(path), ans);
path.pop_back();
}
}
};
/* code provided by PROGIEZ */
93. Restore IP Addresses LeetCode Solution in Java
class Solution {
public List<String> restoreIpAddresses(final String s) {
List<String> ans = new ArrayList<>();
dfs(s, 0, new ArrayList<>(), ans);
return ans;
}
private void dfs(final String s, int start, List<String> path, List<String> ans) {
if (path.size() == 4 && start == s.length()) {
ans.add(String.join(".", path));
return;
}
if (path.size() == 4 || start == s.length())
return;
for (int length = 1; length <= 3; ++length) {
if (start + length > s.length()) // out-of-bounds
return;
if (length > 1 && s.charAt(start) == '0') // leading '0'
return;
final String num = s.substring(start, start + length);
if (Integer.parseInt(num) > 255)
return;
path.add(num);
dfs(s, start + length, path, ans);
path.remove(path.size() - 1);
}
}
}
// code provided by PROGIEZ
93. Restore IP Addresses LeetCode Solution in Python
class Solution:
def restoreIpAddresses(self, s: str) -> list[str]:
ans = []
def dfs(start: int, path: list[int]) -> None:
if len(path) == 4 and start == len(s):
ans.append(path[0] + '.' + path[1] + '.' + path[2] + '.' + path[3])
return
if len(path) == 4 or start == len(s):
return
for length in range(1, 4):
if start + length > len(s):
return # out-of-bounds
if length > 1 and s[start] == '0':
return # leading '0'
num = s[start: start + length]
if int(num) > 255:
return
dfs(start + length, path + [num])
dfs(0, [])
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.