20. Valid Parentheses LeetCode Solution
In this guide we will provide 20. Valid Parentheses LeetCode Solution with best time and space complexity. The solution to Valid Parentheses problem is provided in various programming languages like C++, Java and python. This will be helpful for you if you are preparing for placements, hackathon, interviews or practice purposes. The solutions provided here are very easy to follow and with detailed explanations.
Table of Contents
- Problem Statement
- Valid Parentheses solution in C++
- Valid Parentheses soution in Java
- Valid Parentheses solution Python
- Additional Resources
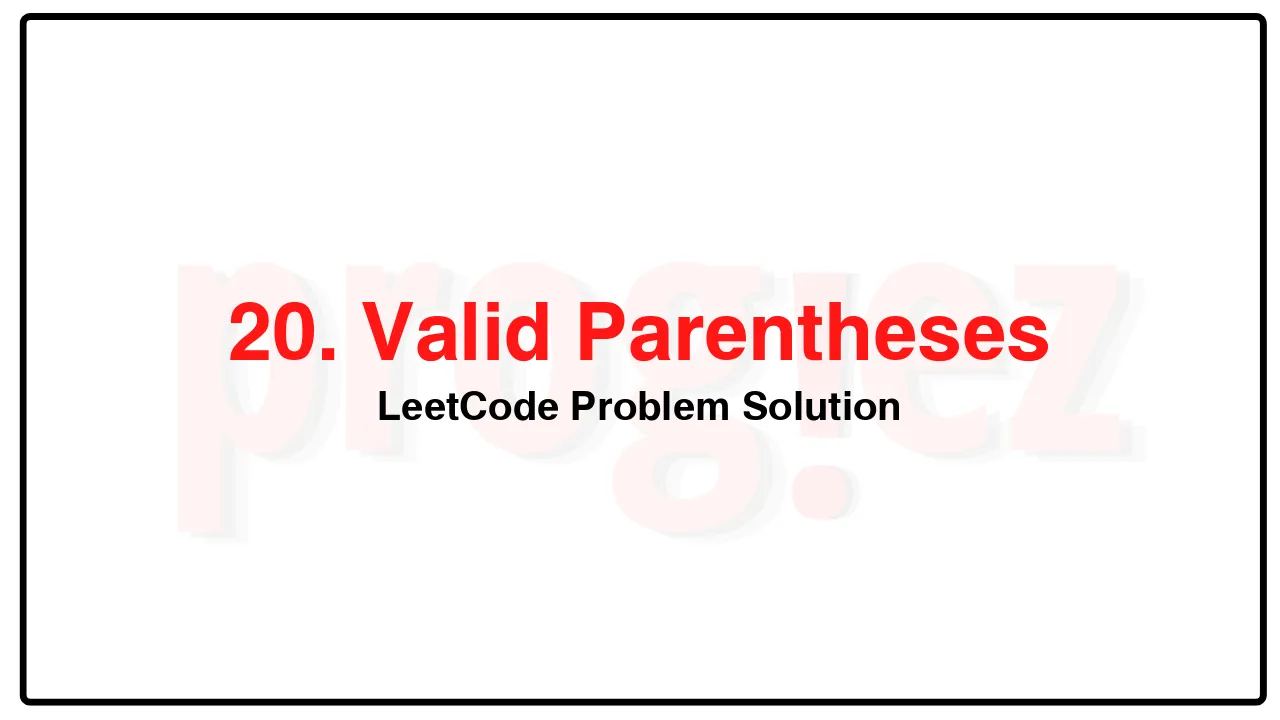
Problem Statement of Valid Parentheses
Given a string s containing just the characters ‘(‘, ‘)’, ‘{‘, ‘}’, ‘[‘ and ‘]’, determine if the input string is valid.
An input string is valid if:
Open brackets must be closed by the same type of brackets.
Open brackets must be closed in the correct order.
Every close bracket has a corresponding open bracket of the same type.
Example 1:
Input: s = “()”
Output: true
Example 2:
Input: s = “()[]{}”
Output: true
Example 3:
Input: s = “(]”
Output: false
Example 4:
Input: s = “([])”
Output: true
Constraints:
1 <= s.length <= 104
s consists of parentheses only '()[]{}'.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
20. Valid Parentheses LeetCode Solution in C++
class Solution {
public:
bool isValid(string s) {
stack<char> stack;
for (const char c : s)
if (c == '(')
stack.push(')');
else if (c == '{')
stack.push('}');
else if (c == '[')
stack.push(']');
else if (stack.empty() || pop(stack) != c)
return false;
return stack.empty();
}
private:
int pop(stack<char>& stack) {
const int c = stack.top();
stack.pop();
return c;
}
};
/* code provided by PROGIEZ */
20. Valid Parentheses LeetCode Solution in Java
class Solution {
public boolean isValid(String s) {
Deque<Character> stack = new ArrayDeque<>();
for (final char c : s.toCharArray())
if (c == '(')
stack.push(')');
else if (c == '{')
stack.push('}');
else if (c == '[')
stack.push(']');
else if (stack.isEmpty() || stack.pop() != c)
return false;
return stack.isEmpty();
}
}
// code provided by PROGIEZ
20. Valid Parentheses LeetCode Solution in Python
class Solution:
def isValid(self, s: str) -> bool:
stack = []
for c in s:
if c == '(':
stack.append(')')
elif c == '{':
stack.append('}')
elif c == '[':
stack.append(']')
elif not stack or stack.pop() != c:
return False
return not stack
#code by PROGIEZ
Additional Resources
- Explore all Leetcode problems solutions at Progiez here
- Explore all problems on Leetcode website here
Feel free to give suggestions! Contact Us