6. Zigzag Conversion LeetCode Solution
In this guide we will provide 6. Zigzag Conversion LeetCode Solution with best time and space complexity. The solution to Zigzag Conversion problem is provided in various programming languages like C++, Java and python. This will be helpful for you if you are preparing for placements, hackathon, interviews or practice purposes. The solutions provided here are very easy to follow and with detailed explanations.
Table of Contents
- Problem Statement
- Zigzag Conversion solution in C++
- Zigzag Conversion soution in Java
- Zigzag Conversion solution Python
- Additional Resources
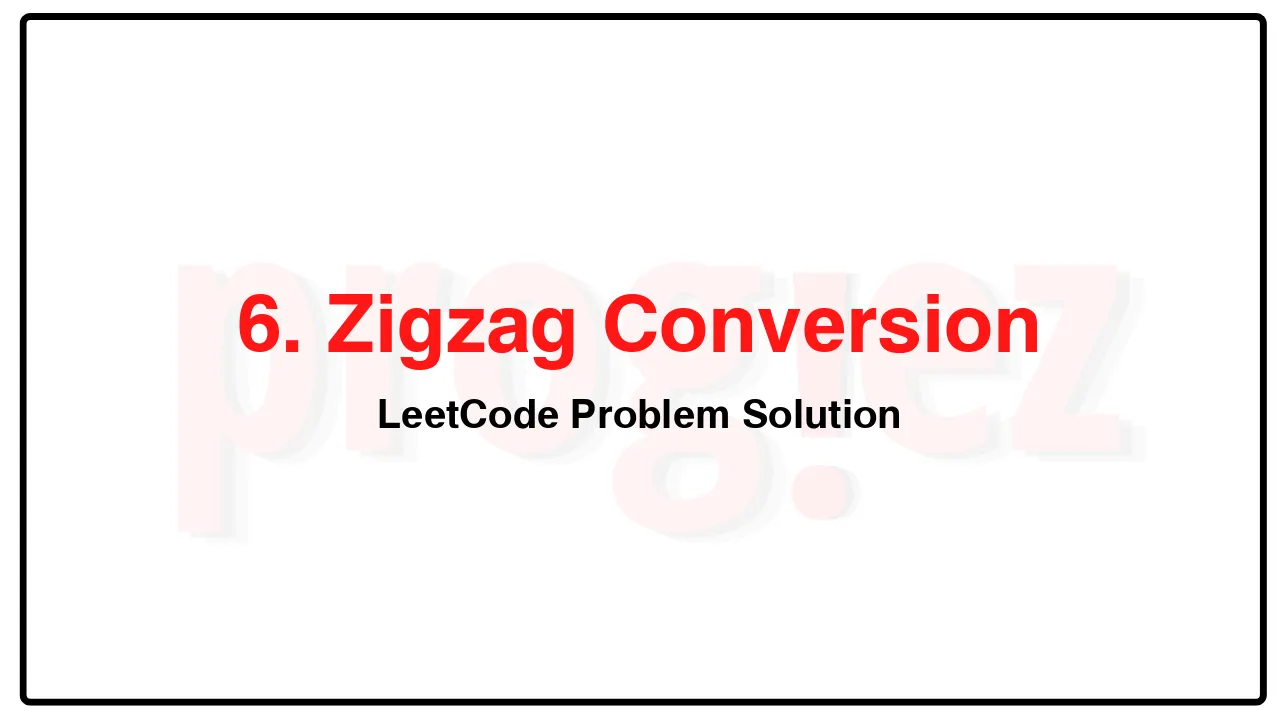
Problem Statement of Zigzag Conversion
The string “PAYPALISHIRING” is written in a zigzag pattern on a given number of rows like this: (you may want to display this pattern in a fixed font for better legibility)
P A H N
A P L S I I G
Y I R
And then read line by line: “PAHNAPLSIIGYIR”
Write the code that will take a string and make this conversion given a number of rows:
string convert(string s, int numRows);
Example 1:
Input: s = “PAYPALISHIRING”, numRows = 3
Output: “PAHNAPLSIIGYIR”
Example 2:
Input: s = “PAYPALISHIRING”, numRows = 4
Output: “PINALSIGYAHRPI”
Explanation:
P I N
A L S I G
Y A H R
P I
Example 3:
Input: s = “A”, numRows = 1
Output: “A”
Constraints:
1 <= s.length <= 1000
s consists of English letters (lower-case and upper-case), ',' and '.'.
1 <= numRows <= 1000
Complexity Analysis
- Time Complexity: O(|\texttt{s}|)
- Space Complexity: O(|\texttt{s}|)
6. Zigzag Conversion LeetCode Solution in C++
class Solution {
public:
string convert(string s, int numRows) {
string ans;
vector<vector<char>> rows(numRows);
int k = 0;
int direction = (numRows == 1) - 1;
for (const char c : s) {
rows[k].push_back(c);
if (k == 0 || k == numRows - 1)
direction *= -1;
k += direction;
}
for (const vector<char>& row : rows)
for (const char c : row)
ans += c;
return ans;
}
};
/* code provided by PROGIEZ */
6. Zigzag Conversion LeetCode Solution in Java
class Solution {
public String convert(String s, int numRows) {
StringBuilder sb = new StringBuilder();
List<Character>[] rows = new List[numRows];
int k = 0;
int direction = numRows == 1 ? 0 : -1;
for (int i = 0; i < numRows; ++i)
rows[i] = new ArrayList<>();
for (final char c : s.toCharArray()) {
rows[k].add(c);
if (k == 0 || k == numRows - 1)
direction *= -1;
k += direction;
}
for (List<Character> row : rows)
for (final char c : row)
sb.append(c);
return sb.toString();
}
}
// code provided by PROGIEZ
6. Zigzag Conversion LeetCode Solution in Python
class Solution:
def convert(self, s: str, numRows: int) -> str:
rows = [''] * numRows
k = 0
direction = (numRows == 1) - 1
for c in s:
rows[k] += c
if k == 0 or k == numRows - 1:
direction *= -1
k += direction
return ''.join(rows)
#code by PROGIEZ
Additional Resources
- Explore all Leetcode problems solutions at Progiez here
- Explore all problems on Leetcode website here
Feel free to give suggestions! Contact Us