557. Reverse Words in a String III LeetCode Solution
In this guide, you will get 557. Reverse Words in a String III LeetCode Solution with the best time and space complexity. The solution to Reverse Words in a String III problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Reverse Words in a String III solution in C++
- Reverse Words in a String III solution in Java
- Reverse Words in a String III solution in Python
- Additional Resources
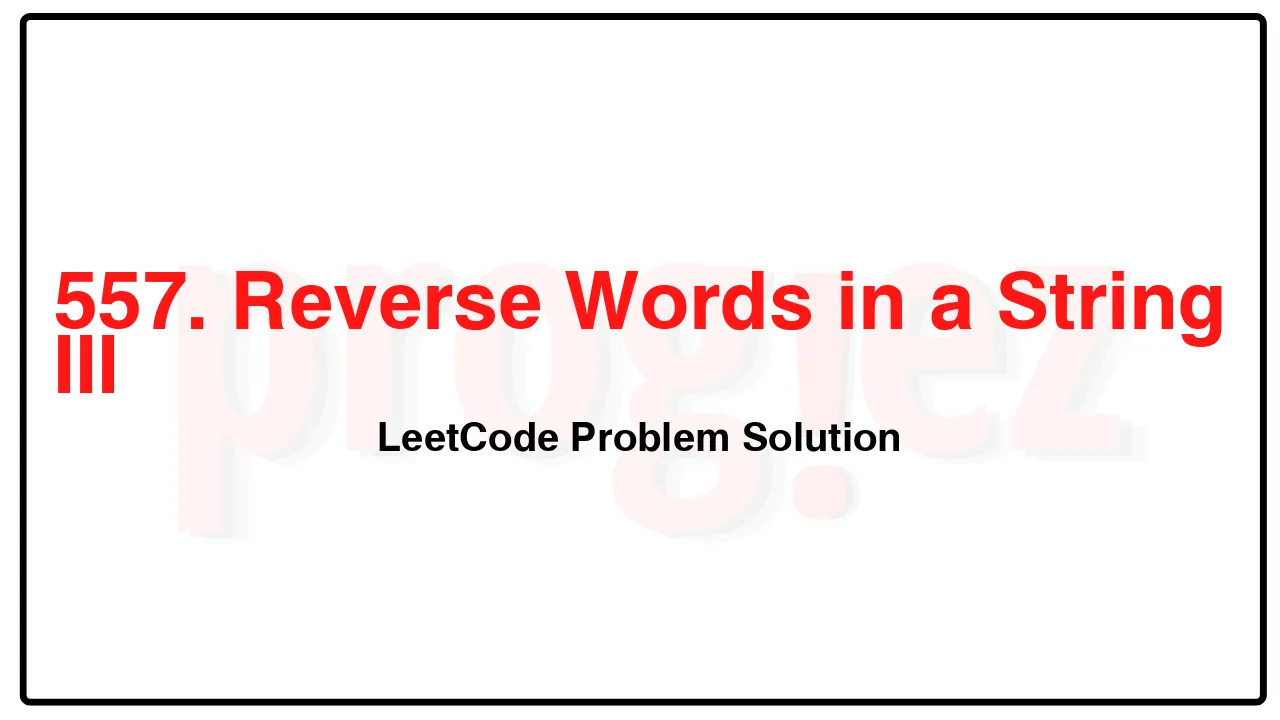
Problem Statement of Reverse Words in a String III
Given a string s, reverse the order of characters in each word within a sentence while still preserving whitespace and initial word order.
Example 1:
Input: s = “Let’s take LeetCode contest”
Output: “s’teL ekat edoCteeL tsetnoc”
Example 2:
Input: s = “Mr Ding”
Output: “rM gniD”
Constraints:
1 <= s.length <= 5 * 104
s contains printable ASCII characters.
s does not contain any leading or trailing spaces.
There is at least one word in s.
All the words in s are separated by a single space.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
557. Reverse Words in a String III LeetCode Solution in C++
class Solution {
public:
string reverseWords(string s) {
int i = 0;
int j = 0;
while (i < s.length()) {
while (i < j || i < s.length() && s[i] == ' ')
++i;
while (j < i || j < s.length() && s[j] != ' ')
++j;
reverse(s.begin() + i, s.begin() + j);
}
return s;
}
};
/* code provided by PROGIEZ */
557. Reverse Words in a String III LeetCode Solution in Java
class Solution {
public String reverseWords(String s) {
StringBuilder sb = new StringBuilder(s);
int i = 0;
int j = 0;
while (i < sb.length()) {
while (i < j || i < sb.length() && sb.charAt(i) == ' ')
++i;
while (j < i || j < sb.length() && sb.charAt(j) != ' ')
++j;
reverse(sb, i, j - 1);
}
return sb.toString();
}
private void reverse(StringBuilder sb, int l, int r) {
while (l < r) {
final char temp = sb.charAt(l);
sb.setCharAt(l++, sb.charAt(r));
sb.setCharAt(r--, temp);
}
}
}
// code provided by PROGIEZ
557. Reverse Words in a String III LeetCode Solution in Python
N/A
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.