542. 01 Matrix LeetCode Solution
In this guide, you will get 542. 01 Matrix LeetCode Solution with the best time and space complexity. The solution to Matrix problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Matrix solution in C++
- Matrix solution in Java
- Matrix solution in Python
- Additional Resources
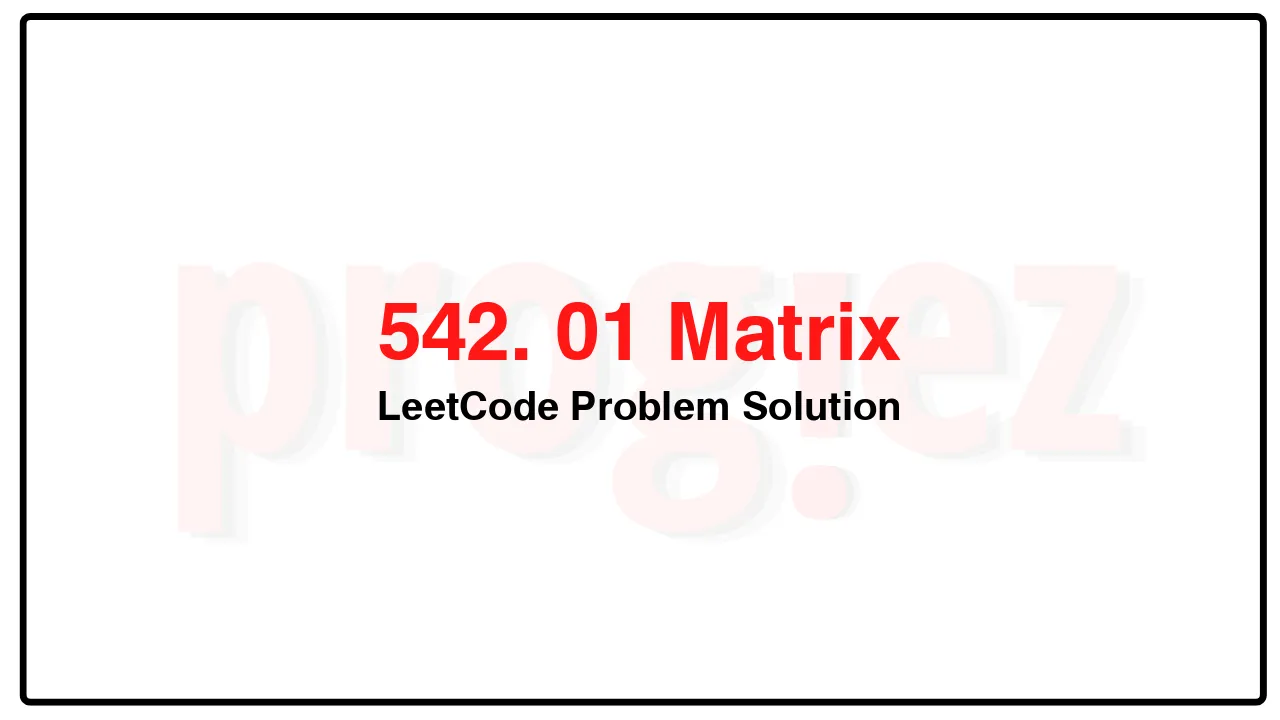
Problem Statement of Matrix
Given an m x n binary matrix mat, return the distance of the nearest 0 for each cell.
The distance between two cells sharing a common edge is 1.
Example 1:
Input: mat = [[0,0,0],[0,1,0],[0,0,0]]
Output: [[0,0,0],[0,1,0],[0,0,0]]
Example 2:
Input: mat = [[0,0,0],[0,1,0],[1,1,1]]
Output: [[0,0,0],[0,1,0],[1,2,1]]
Constraints:
m == mat.length
n == mat[i].length
1 <= m, n <= 104
1 <= m * n <= 104
mat[i][j] is either 0 or 1.
There is at least one 0 in mat.
Note: This question is the same as 1765: https://leetcode.com/problems/map-of-highest-peak/
Complexity Analysis
- Time Complexity: O(mn)
- Space Complexity: O(mn)
542. 01 Matrix LeetCode Solution in C++
class Solution {
public:
vector<vector<int>> updateMatrix(vector<vector<int>>& mat) {
constexpr int dirs[4][2] = {{0, 1}, {1, 0}, {0, -1}, {-1, 0}};
const int m = mat.size();
const int n = mat[0].size();
queue<pair<int, int>> q;
vector<vector<bool>> seen(m, vector<bool>(n));
for (int i = 0; i < m; ++i)
for (int j = 0; j < n; ++j)
if (mat[i][j] == 0) {
q.emplace(i, j);
seen[i][j] = true;
}
while (!q.empty()) {
const auto [i, j] = q.front();
q.pop();
for (const auto& [dx, dy] : dirs) {
const int x = i + dx;
const int y = j + dy;
if (x < 0 || x == m || y < 0 || y == n)
continue;
if (seen[x][y])
continue;
mat[x][y] = mat[i][j] + 1;
q.emplace(x, y);
seen[x][y] = true;
}
}
return mat;
}
};
/* code provided by PROGIEZ */
542. 01 Matrix LeetCode Solution in Java
class Solution {
public int[][] updateMatrix(int[][] mat) {
final int[][] dirs = {{0, 1}, {1, 0}, {0, -1}, {-1, 0}};
final int m = mat.length;
final int n = mat[0].length;
Queue<Pair<Integer, Integer>> q = new ArrayDeque<>();
boolean[][] seen = new boolean[m][n];
for (int i = 0; i < m; ++i)
for (int j = 0; j < n; ++j)
if (mat[i][j] == 0) {
q.offer(new Pair<>(i, j));
seen[i][j] = true;
}
while (!q.isEmpty()) {
final int i = q.peek().getKey();
final int j = q.poll().getValue();
for (int[] dir : dirs) {
final int x = i + dir[0];
final int y = j + dir[1];
if (x < 0 || x == m || y < 0 || y == n)
continue;
if (seen[x][y])
continue;
mat[x][y] = mat[i][j] + 1;
q.offer(new Pair<>(x, y));
seen[x][y] = true;
}
}
return mat;
}
}
// code provided by PROGIEZ
542. 01 Matrix LeetCode Solution in Python
class Solution:
def updateMatrix(self, mat: list[list[int]]) -> list[list[int]]:
dirs = ((0, 1), (1, 0), (0, -1), (-1, 0))
m = len(mat)
n = len(mat[0])
q = collections.deque()
seen = [[False] * n for _ in range(m)]
for i in range(m):
for j in range(n):
if mat[i][j] == 0:
q.append((i, j))
seen[i][j] = True
while q:
i, j = q.popleft()
for dx, dy in dirs:
x = i + dx
y = j + dy
if x < 0 or x == m or y < 0 or y == n:
continue
if seen[x][y]:
continue
mat[x][y] = mat[i][j] + 1
q.append((x, y))
seen[x][y] = True
return mat
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.