3360. Stone Removal Game LeetCode Solution
In this guide, you will get 3360. Stone Removal Game LeetCode Solution with the best time and space complexity. The solution to Stone Removal Game problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Stone Removal Game solution in C++
- Stone Removal Game solution in Java
- Stone Removal Game solution in Python
- Additional Resources
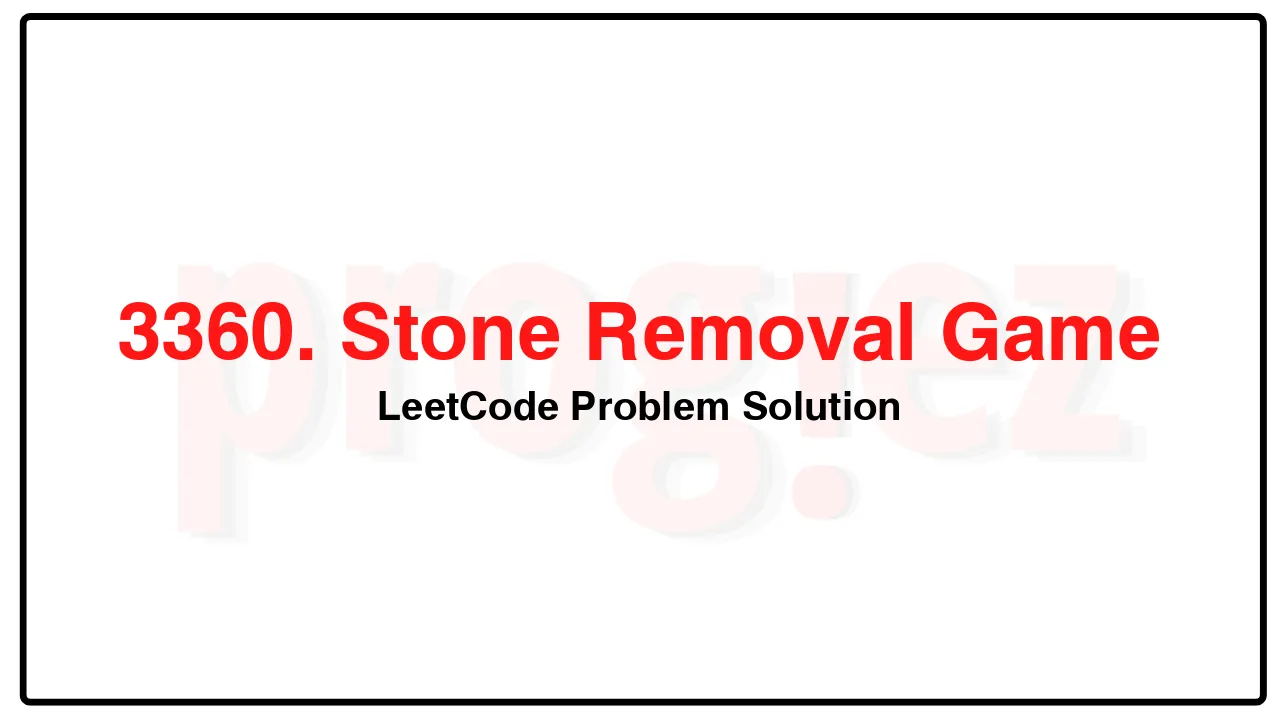
Problem Statement of Stone Removal Game
Alice and Bob are playing a game where they take turns removing stones from a pile, with Alice going first.
Alice starts by removing exactly 10 stones on her first turn.
For each subsequent turn, each player removes exactly 1 fewer stone than the previous opponent.
The player who cannot make a move loses the game.
Given a positive integer n, return true if Alice wins the game and false otherwise.
Example 1:
Input: n = 12
Output: true
Explanation:
Alice removes 10 stones on her first turn, leaving 2 stones for Bob.
Bob cannot remove 9 stones, so Alice wins.
Example 2:
Input: n = 1
Output: false
Explanation:
Alice cannot remove 10 stones, so Alice loses.
Constraints:
1 <= n <= 50
Complexity Analysis
- Time Complexity: O(10) = O(1)
- Space Complexity: O(1)
3360. Stone Removal Game LeetCode Solution in C++
class Solution {
public:
bool canAliceWin(int n) {
for (int stones = 10; stones >= 0; --stones) {
if (stones > n)
return stones % 2 == 1;
n -= stones;
}
throw;
}
};
/* code provided by PROGIEZ */
3360. Stone Removal Game LeetCode Solution in Java
class Solution {
public boolean canAliceWin(int n) {
for (int stones = 10; stones >= 0; --stones) {
if (stones > n)
return stones % 2 == 1;
n -= stones;
}
throw new IllegalArgumentException();
}
}
// code provided by PROGIEZ
3360. Stone Removal Game LeetCode Solution in Python
class Solution:
def canAliceWin(self, n: int) -> bool:
for stones in range(10, -1, -1):
if stones > n:
return stones % 2 == 1
n -= stones
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.