3239. Minimum Number of Flips to Make Binary Grid Palindromic I LeetCode Solution
In this guide, you will get 3239. Minimum Number of Flips to Make Binary Grid Palindromic I LeetCode Solution with the best time and space complexity. The solution to Minimum Number of Flips to Make Binary Grid Palindromic I problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Minimum Number of Flips to Make Binary Grid Palindromic I solution in C++
- Minimum Number of Flips to Make Binary Grid Palindromic I solution in Java
- Minimum Number of Flips to Make Binary Grid Palindromic I solution in Python
- Additional Resources
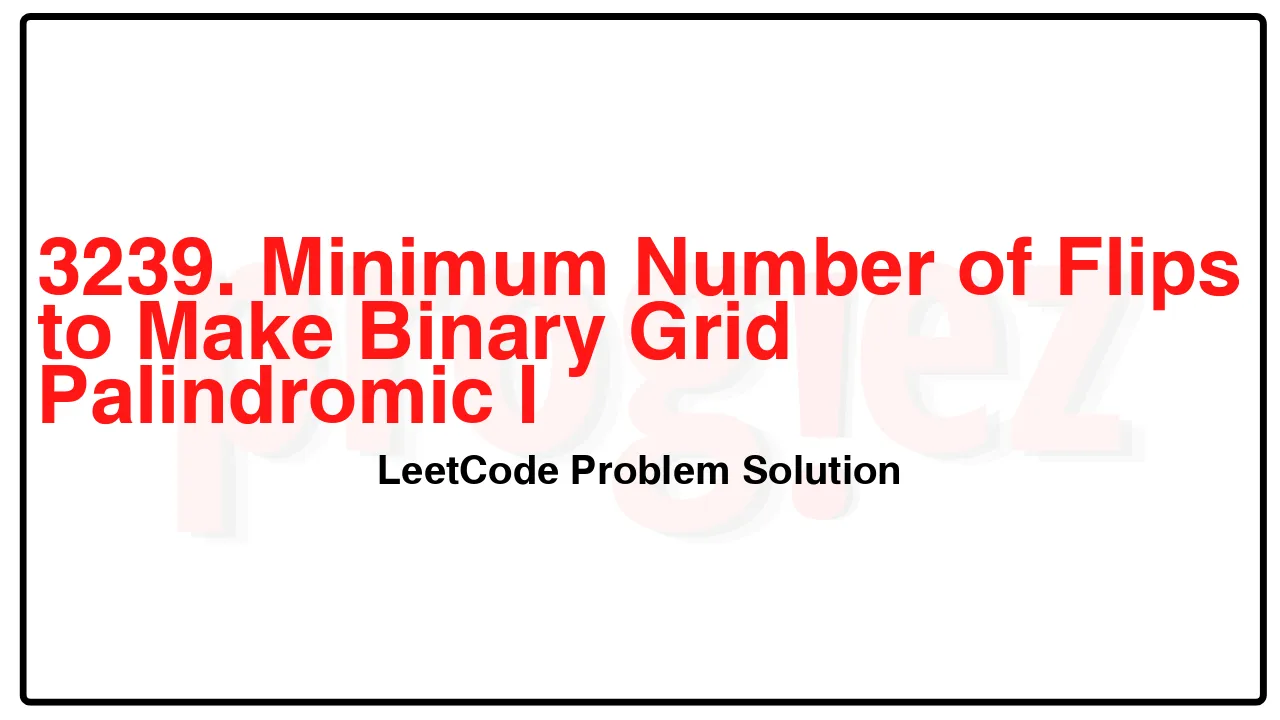
Problem Statement of Minimum Number of Flips to Make Binary Grid Palindromic I
You are given an m x n binary matrix grid.
A row or column is considered palindromic if its values read the same forward and backward.
You can flip any number of cells in grid from 0 to 1, or from 1 to 0.
Return the minimum number of cells that need to be flipped to make either all rows palindromic or all columns palindromic.
Example 1:
Input: grid = [[1,0,0],[0,0,0],[0,0,1]]
Output: 2
Explanation:
Flipping the highlighted cells makes all the rows palindromic.
Example 2:
Input: grid = [[0,1],[0,1],[0,0]]
Output: 1
Explanation:
Flipping the highlighted cell makes all the columns palindromic.
Example 3:
Input: grid = [[1],[0]]
Output: 0
Explanation:
All rows are already palindromic.
Constraints:
m == grid.length
n == grid[i].length
1 <= m * n <= 2 * 105
0 <= grid[i][j] <= 1
Complexity Analysis
- Time Complexity: O(mn)
- Space Complexity: O(1)
3239. Minimum Number of Flips to Make Binary Grid Palindromic I LeetCode Solution in C++
class Solution {
public:
int minFlips(vector<vector<int>>& grid) {
const int m = grid.size();
const int n = grid[0].size();
int rowFlips = 0;
int colFlips = 0;
for (const vector<int>& row : grid)
for (int i = 0; i < n / 2; ++i)
if (row[i] != row[n - 1 - i])
++rowFlips;
for (int j = 0; j < n; ++j)
for (int i = 0; i < m / 2; ++i)
if (grid[i][j] != grid[m - 1 - i][j])
++colFlips;
return min(rowFlips, colFlips);
}
};
/* code provided by PROGIEZ */
3239. Minimum Number of Flips to Make Binary Grid Palindromic I LeetCode Solution in Java
class Solution {
public int minFlips(int[][] grid) {
final int m = grid.length;
final int n = grid[0].length;
int rowFlips = 0;
int colFlips = 0;
for (int[] row : grid)
for (int i = 0; i < n / 2; ++i)
if (row[i] != row[n - 1 - i])
++rowFlips;
for (int j = 0; j < n; ++j)
for (int i = 0; i < m / 2; ++i)
if (grid[i][j] != grid[m - 1 - i][j])
++colFlips;
return Math.min(rowFlips, colFlips);
}
}
// code provided by PROGIEZ
3239. Minimum Number of Flips to Make Binary Grid Palindromic I LeetCode Solution in Python
class Solution:
def minFlips(self, grid: list[list[int]]) -> int:
rowFlips = sum(row[i] != row[-1 - i]
for row in grid for i in range(len(row) // 2))
colFlips = sum(col[i] != col[-1 - i] for col in zip(*grid)
for i in range(len(col) // 2))
return min(rowFlips, colFlips)
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.