3168. Minimum Number of Chairs in a Waiting Room LeetCode Solution
In this guide, you will get 3168. Minimum Number of Chairs in a Waiting Room LeetCode Solution with the best time and space complexity. The solution to Minimum Number of Chairs in a Waiting Room problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Minimum Number of Chairs in a Waiting Room solution in C++
- Minimum Number of Chairs in a Waiting Room solution in Java
- Minimum Number of Chairs in a Waiting Room solution in Python
- Additional Resources
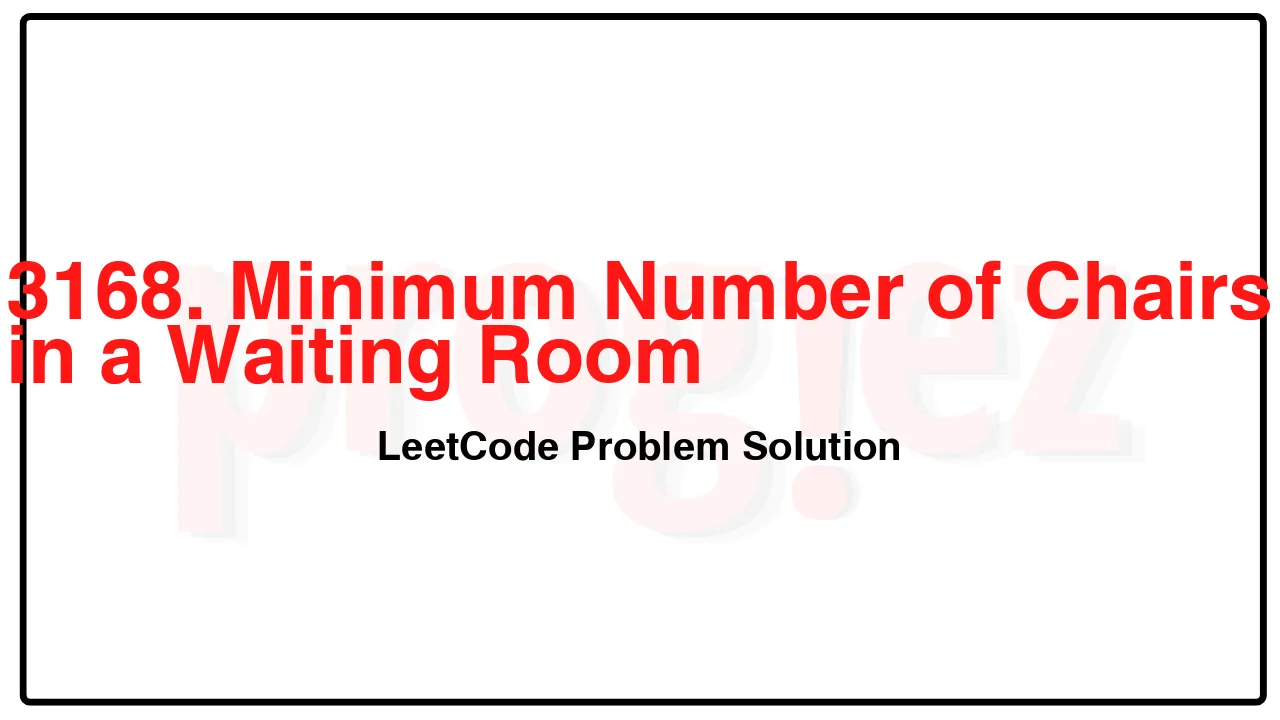
Problem Statement of Minimum Number of Chairs in a Waiting Room
You are given a string s. Simulate events at each second i:
If s[i] == ‘E’, a person enters the waiting room and takes one of the chairs in it.
If s[i] == ‘L’, a person leaves the waiting room, freeing up a chair.
Return the minimum number of chairs needed so that a chair is available for every person who enters the waiting room given that it is initially empty.
Example 1:
Input: s = “EEEEEEE”
Output: 7
Explanation:
After each second, a person enters the waiting room and no person leaves it. Therefore, a minimum of 7 chairs is needed.
Example 2:
Input: s = “ELELEEL”
Output: 2
Explanation:
Let’s consider that there are 2 chairs in the waiting room. The table below shows the state of the waiting room at each second.
Second
Event
People in the Waiting Room
Available Chairs
0
Enter
1
1
1
Leave
0
2
2
Enter
1
1
3
Leave
0
2
4
Enter
1
1
5
Enter
2
0
6
Leave
1
1
Example 3:
Input: s = “ELEELEELLL”
Output: 3
Explanation:
Let’s consider that there are 3 chairs in the waiting room. The table below shows the state of the waiting room at each second.
Second
Event
People in the Waiting Room
Available Chairs
0
Enter
1
2
1
Leave
0
3
2
Enter
1
2
3
Enter
2
1
4
Leave
1
2
5
Enter
2
1
6
Enter
3
0
7
Leave
2
1
8
Leave
1
2
9
Leave
0
3
Constraints:
1 <= s.length <= 50
s consists only of the letters 'E' and 'L'.
s represents a valid sequence of entries and exits.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
3168. Minimum Number of Chairs in a Waiting Room LeetCode Solution in C++
class Solution {
public:
int minimumChairs(string s) {
int ans = 0;
int chairs = 0;
for (const char c : s) {
chairs += c == 'E' ? 1 : -1;
ans = max(ans, chairs);
}
return ans;
}
};
/* code provided by PROGIEZ */
3168. Minimum Number of Chairs in a Waiting Room LeetCode Solution in Java
class Solution {
public int minimumChairs(String s) {
int ans = 0;
int chairs = 0;
for (final char c : s.toCharArray()) {
chairs += c == 'E' ? 1 : -1;
ans = Math.max(ans, chairs);
}
return ans;
}
}
// code provided by PROGIEZ
3168. Minimum Number of Chairs in a Waiting Room LeetCode Solution in Python
class Solution:
def minimumChairs(self, s: str) -> int:
ans = 0
chairs = 0
for c in s:
chairs += 1 if c == 'E' else -1
ans = max(ans, chairs)
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.