3005. Count Elements With Maximum Frequency LeetCode Solution
In this guide, you will get 3005. Count Elements With Maximum Frequency LeetCode Solution with the best time and space complexity. The solution to Count Elements With Maximum Frequency problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Count Elements With Maximum Frequency solution in C++
- Count Elements With Maximum Frequency solution in Java
- Count Elements With Maximum Frequency solution in Python
- Additional Resources
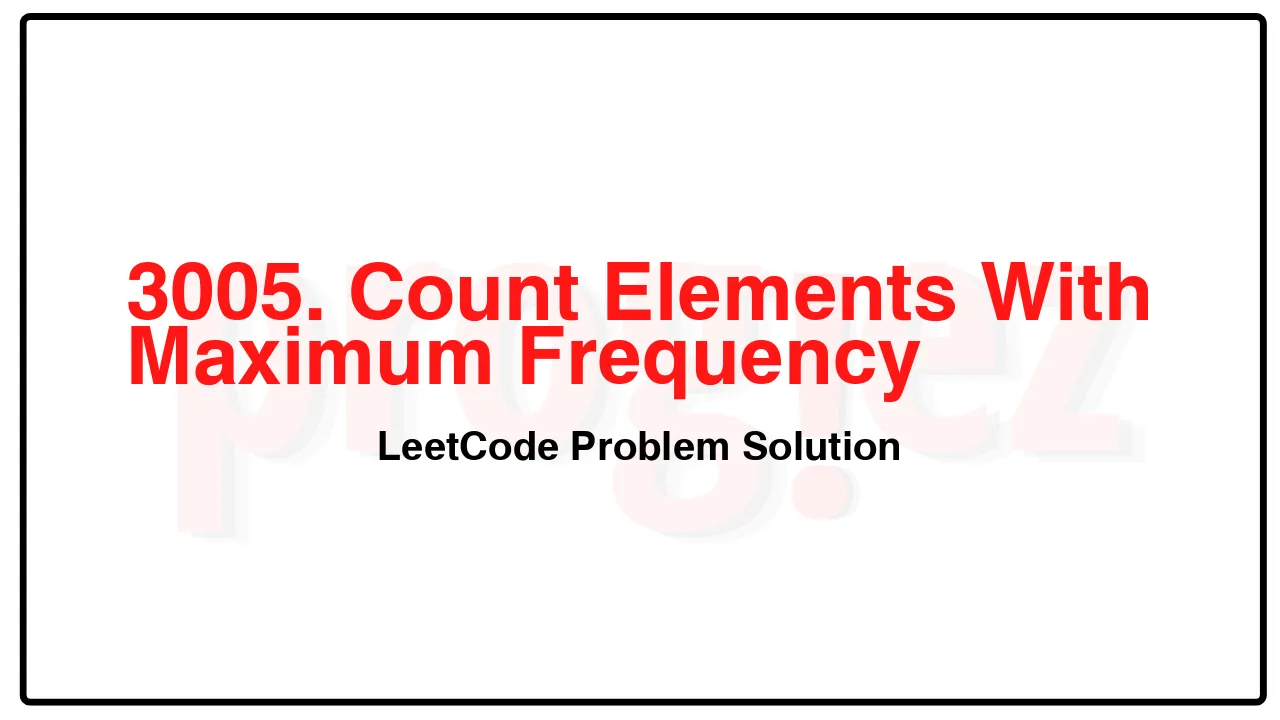
Problem Statement of Count Elements With Maximum Frequency
You are given an array nums consisting of positive integers.
Return the total frequencies of elements in nums such that those elements all have the maximum frequency.
The frequency of an element is the number of occurrences of that element in the array.
Example 1:
Input: nums = [1,2,2,3,1,4]
Output: 4
Explanation: The elements 1 and 2 have a frequency of 2 which is the maximum frequency in the array.
So the number of elements in the array with maximum frequency is 4.
Example 2:
Input: nums = [1,2,3,4,5]
Output: 5
Explanation: All elements of the array have a frequency of 1 which is the maximum.
So the number of elements in the array with maximum frequency is 5.
Constraints:
1 <= nums.length <= 100
1 <= nums[i] <= 100
Complexity Analysis
- Time Complexity:
- Space Complexity:
3005. Count Elements With Maximum Frequency LeetCode Solution in C++
class Solution {
public:
int maxFrequencyElements(vector<int>& nums) {
constexpr int kMax = 100;
vector<int> count(kMax + 1);
for (const int num : nums)
++count[num];
const int maxFreq = ranges::max(count);
return ranges::count(count, maxFreq) * maxFreq;
}
};
/* code provided by PROGIEZ */
3005. Count Elements With Maximum Frequency LeetCode Solution in Java
class Solution {
public int maxFrequencyElements(int[] nums) {
final int kMax = 100;
int ans = 0;
int[] count = new int[kMax + 1];
for (final int num : nums)
++count[num];
final int maxFreq = Arrays.stream(count).max().getAsInt();
for (final int freq : count)
if (freq == maxFreq)
ans += maxFreq;
return ans;
}
}
// code provided by PROGIEZ
3005. Count Elements With Maximum Frequency LeetCode Solution in Python
class Solution:
def maxFrequencyElements(self, nums: list[int]) -> int:
count = collections.Counter(nums)
maxFreq = max(count.values())
return sum(freq == maxFreq for freq in count.values()) * maxFreq
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.