2530. Maximal Score After Applying K Operations LeetCode Solution
In this guide, you will get 2530. Maximal Score After Applying K Operations LeetCode Solution with the best time and space complexity. The solution to Maximal Score After Applying K Operations problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Maximal Score After Applying K Operations solution in C++
- Maximal Score After Applying K Operations solution in Java
- Maximal Score After Applying K Operations solution in Python
- Additional Resources
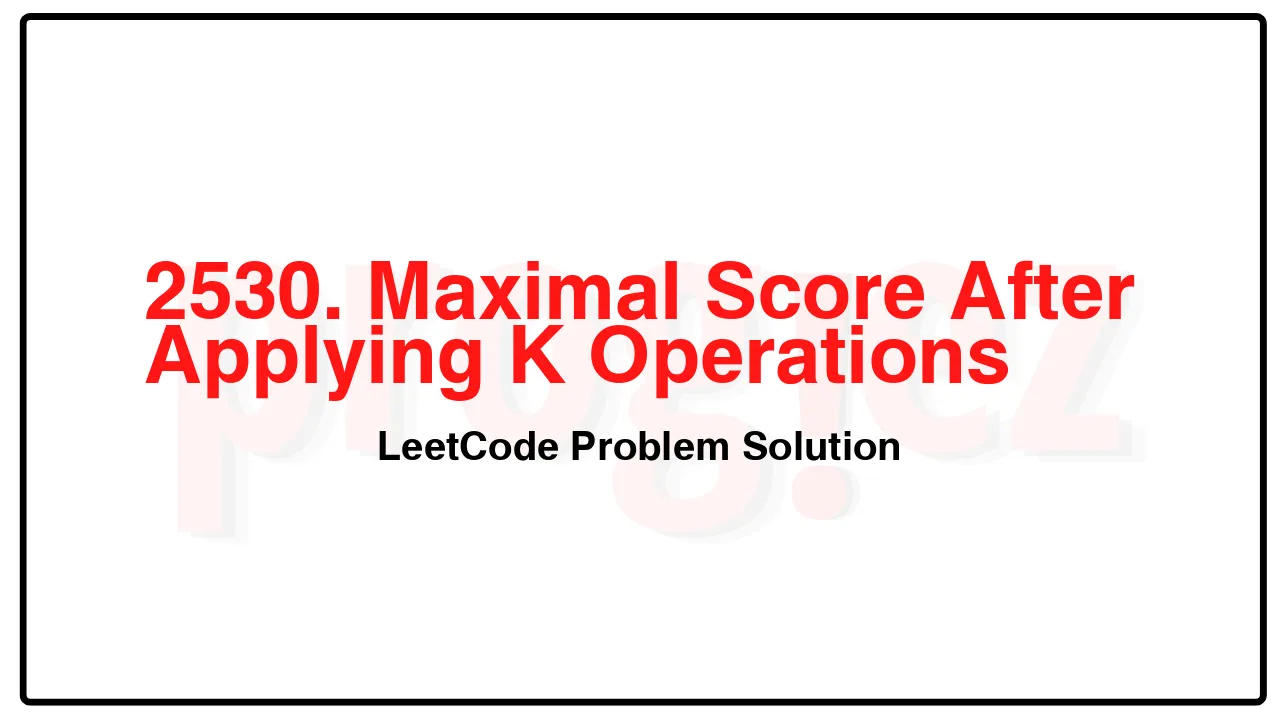
Problem Statement of Maximal Score After Applying K Operations
You are given a 0-indexed integer array nums and an integer k. You have a starting score of 0.
In one operation:
choose an index i such that 0 <= i < nums.length,
increase your score by nums[i], and
replace nums[i] with ceil(nums[i] / 3).
Return the maximum possible score you can attain after applying exactly k operations.
The ceiling function ceil(val) is the least integer greater than or equal to val.
Example 1:
Input: nums = [10,10,10,10,10], k = 5
Output: 50
Explanation: Apply the operation to each array element exactly once. The final score is 10 + 10 + 10 + 10 + 10 = 50.
Example 2:
Input: nums = [1,10,3,3,3], k = 3
Output: 17
Explanation: You can do the following operations:
Operation 1: Select i = 1, so nums becomes [1,4,3,3,3]. Your score increases by 10.
Operation 2: Select i = 1, so nums becomes [1,2,3,3,3]. Your score increases by 4.
Operation 3: Select i = 2, so nums becomes [1,2,1,3,3]. Your score increases by 3.
The final score is 10 + 4 + 3 = 17.
Constraints:
1 <= nums.length, k <= 105
1 <= nums[i] <= 109
Complexity Analysis
- Time Complexity: O(n\log k)
- Space Complexity: O(n)
2530. Maximal Score After Applying K Operations LeetCode Solution in C++
class Solution {
public:
long long maxKelements(vector<int>& nums, int k) {
long ans = 0;
priority_queue<int> maxHeap;
for (const int num : nums)
maxHeap.push(num);
for (int i = 0; i < k; ++i) {
const int num = maxHeap.top();
maxHeap.pop();
ans += num;
maxHeap.push((num + 2) / 3);
}
return ans;
}
};
/* code provided by PROGIEZ */
2530. Maximal Score After Applying K Operations LeetCode Solution in Java
class Solution {
public long maxKelements(int[] nums, int k) {
long ans = 0;
Queue<Integer> maxHeap = new PriorityQueue<>(Collections.reverseOrder());
for (final int num : nums)
maxHeap.offer(num);
for (int i = 0; i < k; ++i) {
final int num = maxHeap.poll();
ans += num;
maxHeap.offer((num + 2) / 3);
}
return ans;
}
}
// code provided by PROGIEZ
2530. Maximal Score After Applying K Operations LeetCode Solution in Python
class Solution:
def maxKelements(self, nums: list[int], k: int) -> int:
ans = 0
maxHeap = [-num for num in nums]
heapq.heapify(maxHeap)
for _ in range(k):
num = -heapq.heappop(maxHeap)
ans += num
heapq.heappush(maxHeap, -math.ceil(num / 3))
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.