2448. Minimum Cost to Make Array Equal LeetCode Solution
In this guide, you will get 2448. Minimum Cost to Make Array Equal LeetCode Solution with the best time and space complexity. The solution to Minimum Cost to Make Array Equal problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Minimum Cost to Make Array Equal solution in C++
- Minimum Cost to Make Array Equal solution in Java
- Minimum Cost to Make Array Equal solution in Python
- Additional Resources
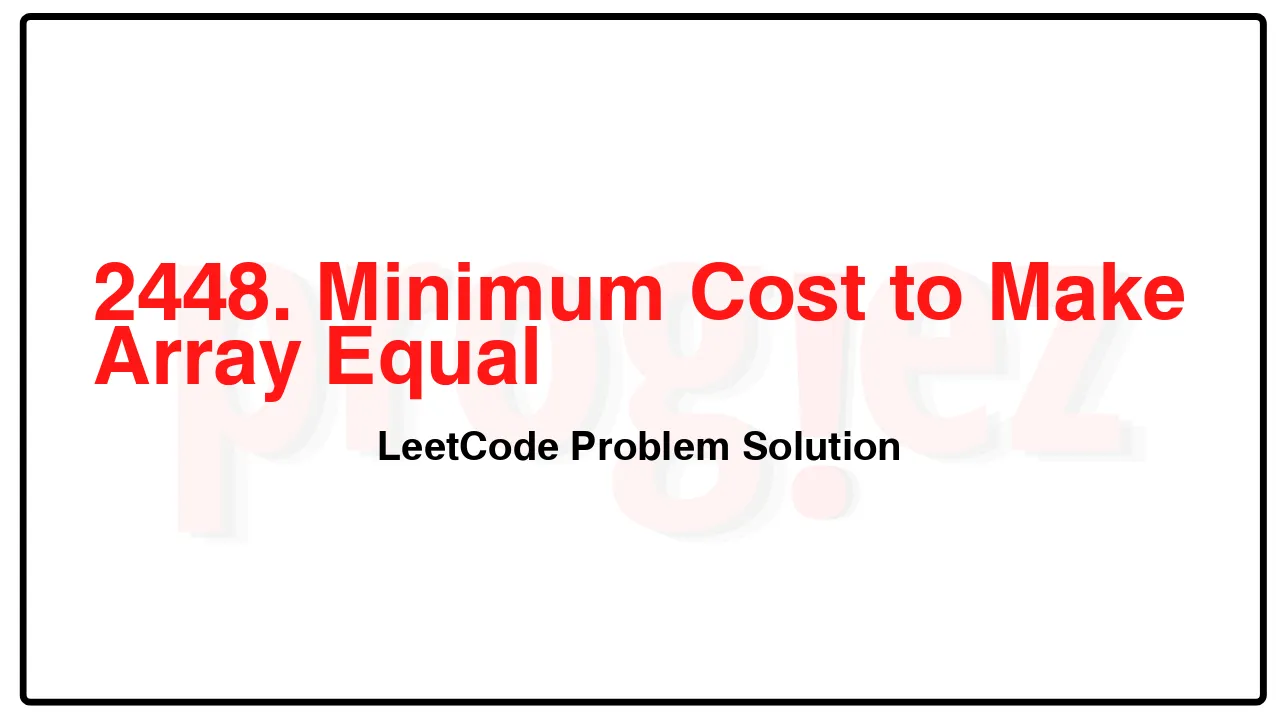
Problem Statement of Minimum Cost to Make Array Equal
You are given two 0-indexed arrays nums and cost consisting each of n positive integers.
You can do the following operation any number of times:
Increase or decrease any element of the array nums by 1.
The cost of doing one operation on the ith element is cost[i].
Return the minimum total cost such that all the elements of the array nums become equal.
Example 1:
Input: nums = [1,3,5,2], cost = [2,3,1,14]
Output: 8
Explanation: We can make all the elements equal to 2 in the following way:
– Increase the 0th element one time. The cost is 2.
– Decrease the 1st element one time. The cost is 3.
– Decrease the 2nd element three times. The cost is 1 + 1 + 1 = 3.
The total cost is 2 + 3 + 3 = 8.
It can be shown that we cannot make the array equal with a smaller cost.
Example 2:
Input: nums = [2,2,2,2,2], cost = [4,2,8,1,3]
Output: 0
Explanation: All the elements are already equal, so no operations are needed.
Constraints:
n == nums.length == cost.length
1 <= n <= 105
1 <= nums[i], cost[i] <= 106
Test cases are generated in a way that the output doesn't exceed 253-1
Complexity Analysis
- Time Complexity: O(n\log (\max(\texttt{nums}) – \min(\texttt{nums})))
- Space Complexity: O(1)
2448. Minimum Cost to Make Array Equal LeetCode Solution in C++
class Solution {
public:
long long minCost(vector<int>& nums, vector<int>& cost) {
long ans = 0;
int l = ranges::min(nums);
int r = ranges::max(nums);
while (l < r) {
const int m = (l + r) / 2;
const long cost1 = getCost(nums, cost, m);
const long cost2 = getCost(nums, cost, m + 1);
ans = min(cost1, cost2);
if (cost1 < cost2)
r = m;
else
l = m + 1;
}
return ans;
}
private:
long getCost(const vector<int>& nums, const vector<int>& cost, int target) {
long res = 0;
for (int i = 0; i < nums.size(); ++i)
res += static_cast<long>(abs(nums[i] - target)) * cost[i];
return res;
}
};
/* code provided by PROGIEZ */
2448. Minimum Cost to Make Array Equal LeetCode Solution in Java
class Solution {
public long minCost(int[] nums, int[] cost) {
long ans = 0;
int l = Arrays.stream(nums).min().getAsInt();
int r = Arrays.stream(nums).max().getAsInt();
while (l < r) {
final int m = (l + r) / 2;
final long cost1 = getCost(nums, cost, m);
final long cost2 = getCost(nums, cost, m + 1);
ans = Math.min(cost1, cost2);
if (cost1 < cost2)
r = m;
else
l = m + 1;
}
return ans;
}
private long getCost(int[] nums, int[] cost, int target) {
long res = 0;
for (int i = 0; i < nums.length; ++i)
res += Math.abs(nums[i] - target) * (long) cost[i];
return res;
}
}
// code provided by PROGIEZ
2448. Minimum Cost to Make Array Equal LeetCode Solution in Python
class Solution:
def minCost(self, nums: list[int], cost: list[int]) -> int:
ans = 0
l = min(nums)
r = max(nums)
def getCost(target: int) -> int:
return sum(abs(num - target) * c for num, c in zip(nums, cost))
while l < r:
m = (l + r) // 2
cost1 = getCost(m)
cost2 = getCost(m + 1)
ans = min(cost1, cost2)
if cost1 < cost2:
r = m
else:
l = m + 1
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.