2425. Bitwise XOR of All Pairings LeetCode Solution
In this guide, you will get 2425. Bitwise XOR of All Pairings LeetCode Solution with the best time and space complexity. The solution to Bitwise XOR of All Pairings problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Bitwise XOR of All Pairings solution in C++
- Bitwise XOR of All Pairings solution in Java
- Bitwise XOR of All Pairings solution in Python
- Additional Resources
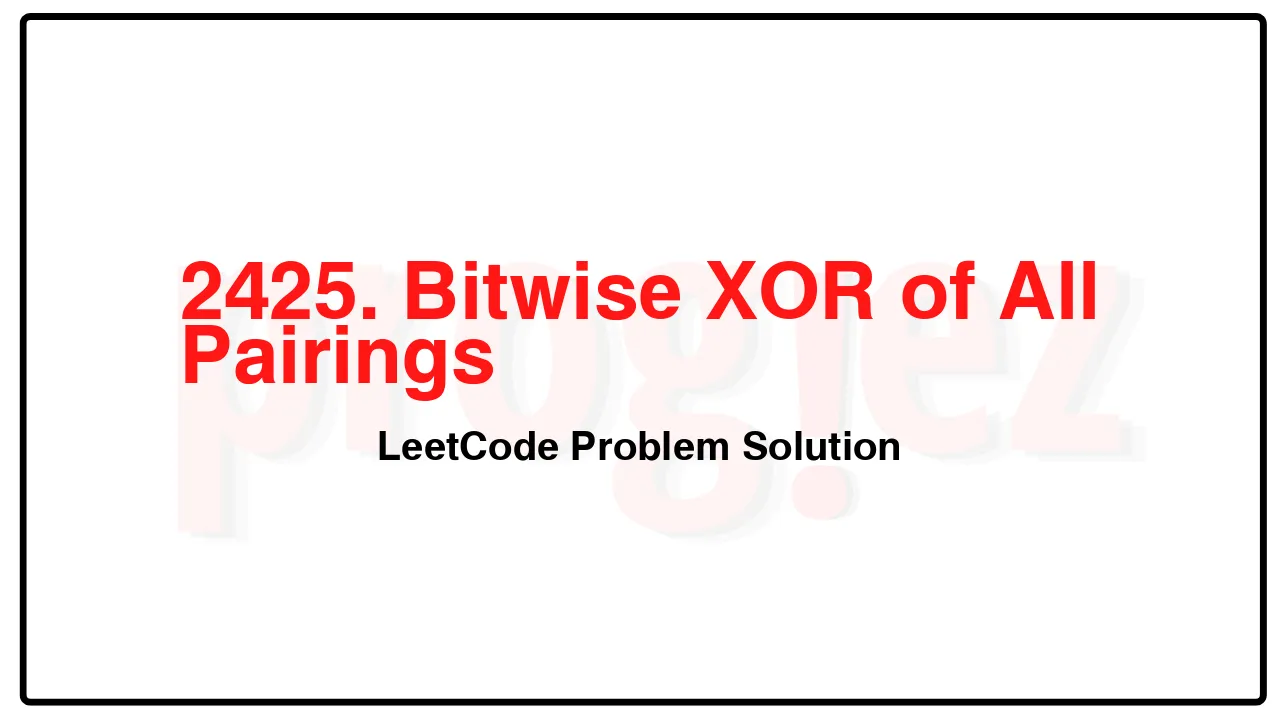
Problem Statement of Bitwise XOR of All Pairings
You are given two 0-indexed arrays, nums1 and nums2, consisting of non-negative integers. Let there be another array, nums3, which contains the bitwise XOR of all pairings of integers between nums1 and nums2 (every integer in nums1 is paired with every integer in nums2 exactly once).
Return the bitwise XOR of all integers in nums3.
Example 1:
Input: nums1 = [2,1,3], nums2 = [10,2,5,0]
Output: 13
Explanation:
A possible nums3 array is [8,0,7,2,11,3,4,1,9,1,6,3].
The bitwise XOR of all these numbers is 13, so we return 13.
Example 2:
Input: nums1 = [1,2], nums2 = [3,4]
Output: 0
Explanation:
All possible pairs of bitwise XORs are nums1[0] ^ nums2[0], nums1[0] ^ nums2[1], nums1[1] ^ nums2[0],
and nums1[1] ^ nums2[1].
Thus, one possible nums3 array is [2,5,1,6].
2 ^ 5 ^ 1 ^ 6 = 0, so we return 0.
Constraints:
1 <= nums1.length, nums2.length <= 105
0 <= nums1[i], nums2[j] <= 109
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
2425. Bitwise XOR of All Pairings LeetCode Solution in C++
class Solution {
public:
int xorAllNums(vector<int>& nums1, vector<int>& nums2) {
// If the size of nums1 is m and the size of nums2 is n, then each number in
// nums1 is repeated n times and each number in nums2 is repeated m times.
const int xors1 = accumulate(nums1.begin(), nums1.end(), 0, bit_xor<>());
const int xors2 = accumulate(nums2.begin(), nums2.end(), 0, bit_xor<>());
return (nums1.size() % 2 * xors2) ^ (nums2.size() % 2 * xors1);
}
};
/* code provided by PROGIEZ */
2425. Bitwise XOR of All Pairings LeetCode Solution in Java
class Solution {
public int xorAllNums(int[] nums1, int[] nums2) {
// If the size of nums1 is m and the size of nums2 is n, then each number in
// nums1 is repeated n times and each number in nums2 is repeated m times.
final int xors1 = Arrays.stream(nums1).reduce((a, b) -> a ^ b).getAsInt();
final int xors2 = Arrays.stream(nums2).reduce((a, b) -> a ^ b).getAsInt();
return (nums1.length % 2 * xors2) ^ (nums2.length % 2 * xors1);
}
}
// code provided by PROGIEZ
2425. Bitwise XOR of All Pairings LeetCode Solution in Python
class Solution:
def xorAllNums(self, nums1: list[int], nums2: list[int]) -> int:
xors1 = functools.reduce(operator.xor, nums1)
xors2 = functools.reduce(operator.xor, nums2)
# If the size of nums1 is m and the size of nums2 is n, then each number in
# nums1 is repeated n times and each number in nums2 is repeated m times.
return (len(nums1) % 2 * xors2) ^ (len(nums2) % 2 * xors1)
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.