2404. Most Frequent Even Element LeetCode Solution
In this guide, you will get 2404. Most Frequent Even Element LeetCode Solution with the best time and space complexity. The solution to Most Frequent Even Element problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Most Frequent Even Element solution in C++
- Most Frequent Even Element solution in Java
- Most Frequent Even Element solution in Python
- Additional Resources
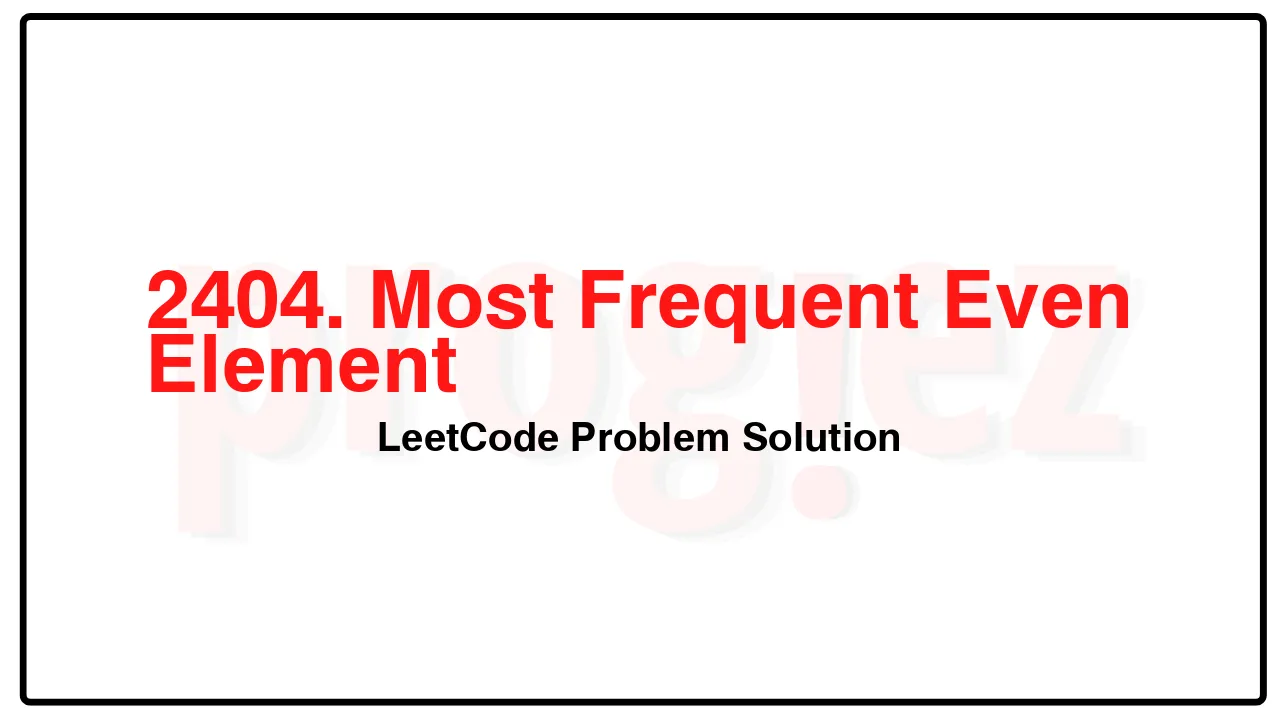
Problem Statement of Most Frequent Even Element
Given an integer array nums, return the most frequent even element.
If there is a tie, return the smallest one. If there is no such element, return -1.
Example 1:
Input: nums = [0,1,2,2,4,4,1]
Output: 2
Explanation:
The even elements are 0, 2, and 4. Of these, 2 and 4 appear the most.
We return the smallest one, which is 2.
Example 2:
Input: nums = [4,4,4,9,2,4]
Output: 4
Explanation: 4 is the even element appears the most.
Example 3:
Input: nums = [29,47,21,41,13,37,25,7]
Output: -1
Explanation: There is no even element.
Constraints:
1 <= nums.length <= 2000
0 <= nums[i] <= 105
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
2404. Most Frequent Even Element LeetCode Solution in C++
class Solution {
public:
int mostFrequentEven(vector<int>& nums) {
int ans = -1;
unordered_map<int, int> count;
for (const int num : nums) {
if (num % 2 == 1)
continue;
const int newCount = ++count[num];
const int maxCount = count[ans];
if (newCount > maxCount || newCount == maxCount && num < ans)
ans = num;
}
return ans;
}
};
/* code provided by PROGIEZ */
2404. Most Frequent Even Element LeetCode Solution in Java
class Solution {
public int mostFrequentEven(int[] nums) {
int ans = -1;
Map<Integer, Integer> count = new HashMap<>();
for (final int num : nums) {
if (num % 2 == 1)
continue;
final int newCount = count.merge(num, 1, Integer::sum);
final int maxCount = count.getOrDefault(ans, 0);
if (newCount > maxCount || newCount == maxCount && num < ans)
ans = num;
}
return ans;
}
}
// code provided by PROGIEZ
2404. Most Frequent Even Element LeetCode Solution in Python
class Solution:
def mostFrequentEven(self, nums: list[int]) -> int:
ans = -1
count = collections.Counter()
for num in nums:
if num % 2 == 1:
continue
count[num] += 1
newCount = count[num]
maxCount = count[ans]
if newCount > maxCount or newCount == maxCount and num < ans:
ans = num
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.