2351. First Letter to Appear Twice LeetCode Solution
In this guide, you will get 2351. First Letter to Appear Twice LeetCode Solution with the best time and space complexity. The solution to First Letter to Appear Twice problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- First Letter to Appear Twice solution in C++
- First Letter to Appear Twice solution in Java
- First Letter to Appear Twice solution in Python
- Additional Resources
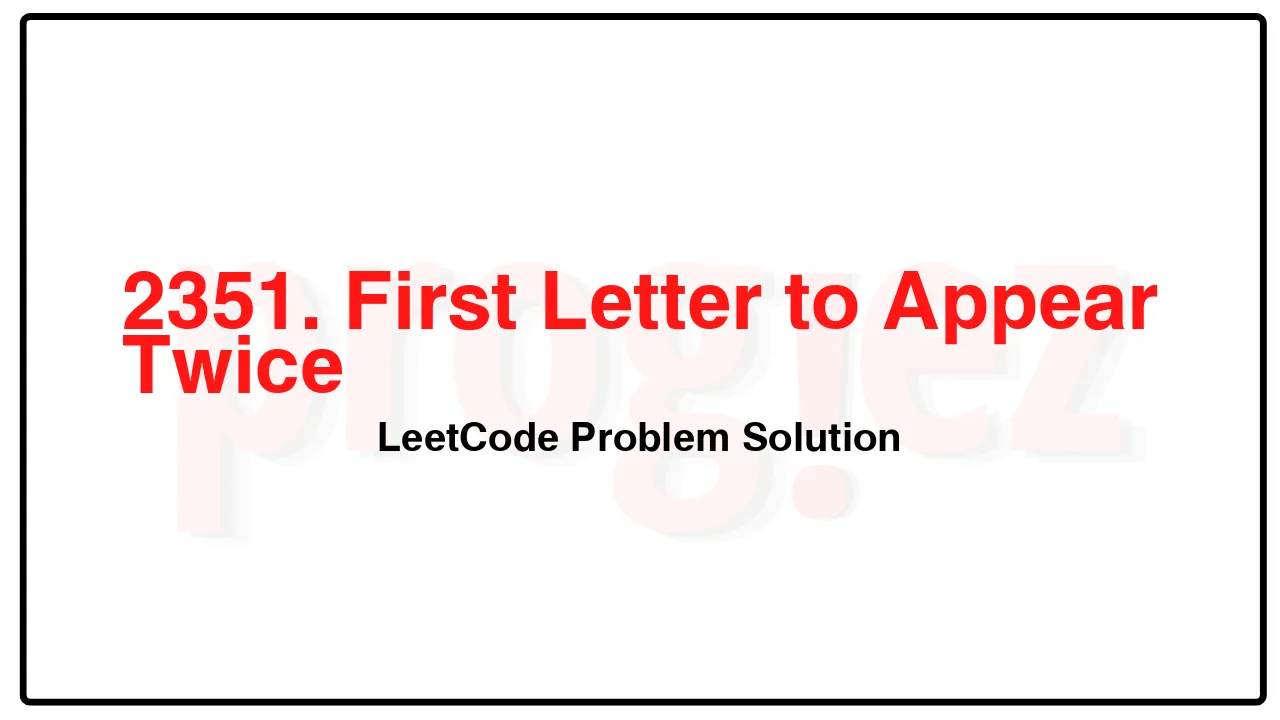
Problem Statement of First Letter to Appear Twice
Given a string s consisting of lowercase English letters, return the first letter to appear twice.
Note:
A letter a appears twice before another letter b if the second occurrence of a is before the second occurrence of b.
s will contain at least one letter that appears twice.
Example 1:
Input: s = “abccbaacz”
Output: “c”
Explanation:
The letter ‘a’ appears on the indexes 0, 5 and 6.
The letter ‘b’ appears on the indexes 1 and 4.
The letter ‘c’ appears on the indexes 2, 3 and 7.
The letter ‘z’ appears on the index 8.
The letter ‘c’ is the first letter to appear twice, because out of all the letters the index of its second occurrence is the smallest.
Example 2:
Input: s = “abcdd”
Output: “d”
Explanation:
The only letter that appears twice is ‘d’ so we return ‘d’.
Constraints:
2 <= s.length <= 100
s consists of lowercase English letters.
s has at least one repeated letter.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(26) = O(1)
2351. First Letter to Appear Twice LeetCode Solution in C++
class Solution {
public:
char repeatedCharacter(string s) {
vector<bool> seen(26);
for (const char c : s) {
if (seen[c - 'a'])
return c;
seen[c - 'a'] = true;
}
throw;
}
};
/* code provided by PROGIEZ */
2351. First Letter to Appear Twice LeetCode Solution in Java
class Solution {
public char repeatedCharacter(String s) {
boolean[] seen = new boolean[26];
for (final char c : s.toCharArray()) {
if (seen[c - 'a'])
return c;
seen[c - 'a'] = true;
}
throw new IllegalArgumentException();
}
}
// code provided by PROGIEZ
2351. First Letter to Appear Twice LeetCode Solution in Python
class Solution:
def repeatedCharacter(self, s: str) -> str:
seen = [False] * 26
for c in s:
if seen[ord(c) - ord('a')]:
return c
seen[ord(c) - ord('a')] = True
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.