2321. Maximum Score Of Spliced Array LeetCode Solution
In this guide, you will get 2321. Maximum Score Of Spliced Array LeetCode Solution with the best time and space complexity. The solution to Maximum Score Of Spliced Array problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Maximum Score Of Spliced Array solution in C++
- Maximum Score Of Spliced Array solution in Java
- Maximum Score Of Spliced Array solution in Python
- Additional Resources
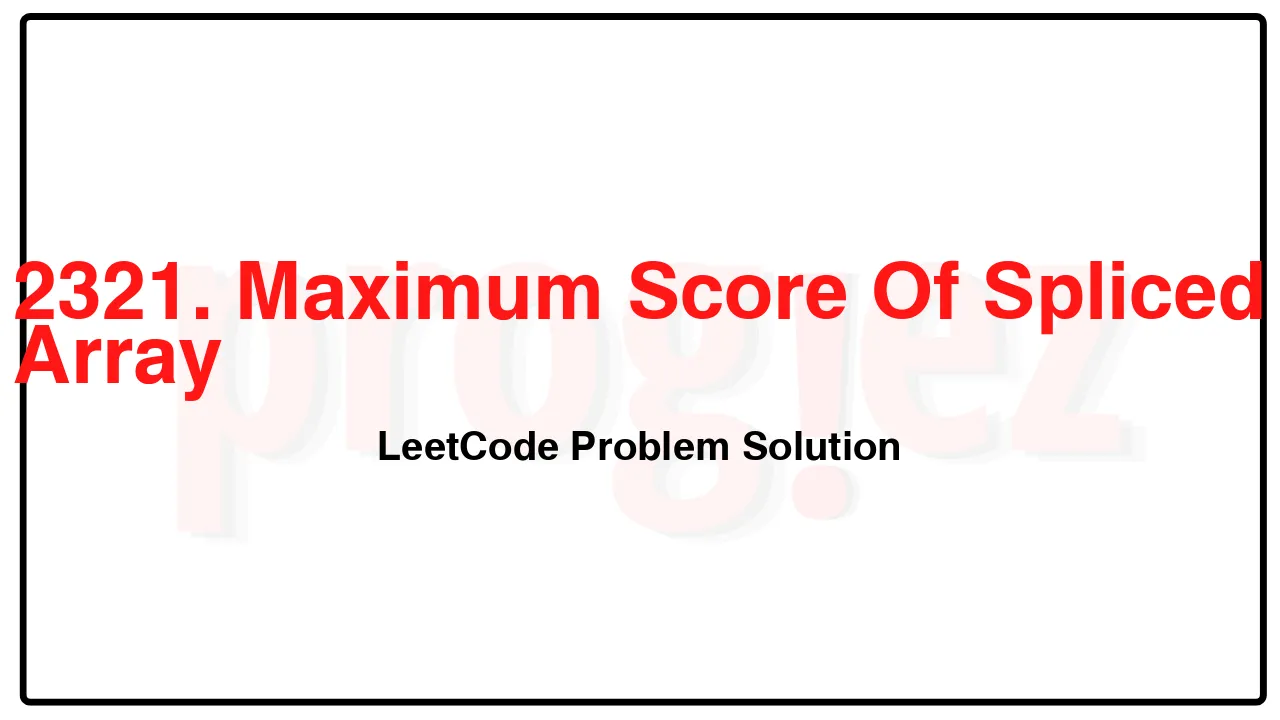
Problem Statement of Maximum Score Of Spliced Array
You are given two 0-indexed integer arrays nums1 and nums2, both of length n.
You can choose two integers left and right where 0 <= left <= right < n and swap the subarray nums1[left…right] with the subarray nums2[left…right].
For example, if nums1 = [1,2,3,4,5] and nums2 = [11,12,13,14,15] and you choose left = 1 and right = 2, nums1 becomes [1,12,13,4,5] and nums2 becomes [11,2,3,14,15].
You may choose to apply the mentioned operation once or not do anything.
The score of the arrays is the maximum of sum(nums1) and sum(nums2), where sum(arr) is the sum of all the elements in the array arr.
Return the maximum possible score.
A subarray is a contiguous sequence of elements within an array. arr[left…right] denotes the subarray that contains the elements of nums between indices left and right (inclusive).
Example 1:
Input: nums1 = [60,60,60], nums2 = [10,90,10]
Output: 210
Explanation: Choosing left = 1 and right = 1, we have nums1 = [60,90,60] and nums2 = [10,60,10].
The score is max(sum(nums1), sum(nums2)) = max(210, 80) = 210.
Example 2:
Input: nums1 = [20,40,20,70,30], nums2 = [50,20,50,40,20]
Output: 220
Explanation: Choosing left = 3, right = 4, we have nums1 = [20,40,20,40,20] and nums2 = [50,20,50,70,30].
The score is max(sum(nums1), sum(nums2)) = max(140, 220) = 220.
Example 3:
Input: nums1 = [7,11,13], nums2 = [1,1,1]
Output: 31
Explanation: We choose not to swap any subarray.
The score is max(sum(nums1), sum(nums2)) = max(31, 3) = 31.
Constraints:
n == nums1.length == nums2.length
1 <= n <= 105
1 <= nums1[i], nums2[i] <= 104
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
2321. Maximum Score Of Spliced Array LeetCode Solution in C++
class Solution {
public:
int maximumsSplicedArray(vector<int>& nums1, vector<int>& nums2) {
return max(kadane(nums1, nums2), kadane(nums2, nums1));
}
private:
// Returns the maximum gain of swapping some numbers in `nums1` with some
// numbers in `nums2`.
int kadane(const vector<int>& nums1, const vector<int>& nums2) {
int gain = 0;
int maxGain = 0;
for (int i = 0; i < nums1.size(); ++i) {
gain = max(0, gain + nums2[i] - nums1[i]);
maxGain = max(maxGain, gain);
}
return maxGain + accumulate(nums1.begin(), nums1.end(), 0);
}
};
/* code provided by PROGIEZ */
2321. Maximum Score Of Spliced Array LeetCode Solution in Java
class Solution {
public int maximumsSplicedArray(int[] nums1, int[] nums2) {
return Math.max(kadane(nums1, nums2), kadane(nums2, nums1));
}
// Returns the maximum gain of swapping some numbers in `nums1` with some numbers in `nums2`.
private int kadane(int[] nums1, int[] nums2) {
int gain = 0;
int maxGain = 0;
for (int i = 0; i < nums1.length; ++i) {
gain = Math.max(0, gain + nums2[i] - nums1[i]);
maxGain = Math.max(maxGain, gain);
}
return maxGain + Arrays.stream(nums1).sum();
}
}
// code provided by PROGIEZ
2321. Maximum Score Of Spliced Array LeetCode Solution in Python
class Solution:
def maximumsSplicedArray(self, nums1: list[int], nums2: list[int]) -> int:
def kadane(nums1: list[int], nums2: list[int]) -> int:
"""
Returns the maximum gain of swapping some numbers in `nums1` with some
numbers in `nums2`.
"""
gain = 0
maxGain = 0
for num1, num2 in zip(nums1, nums2):
gain = max(0, gain + num2 - num1)
maxGain = max(maxGain, gain)
return maxGain + sum(nums1)
return max(kadane(nums1, nums2), kadane(nums2, nums1))
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.