2206. Divide Array Into Equal Pairs LeetCode Solution
In this guide, you will get 2206. Divide Array Into Equal Pairs LeetCode Solution with the best time and space complexity. The solution to Divide Array Into Equal Pairs problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Divide Array Into Equal Pairs solution in C++
- Divide Array Into Equal Pairs solution in Java
- Divide Array Into Equal Pairs solution in Python
- Additional Resources
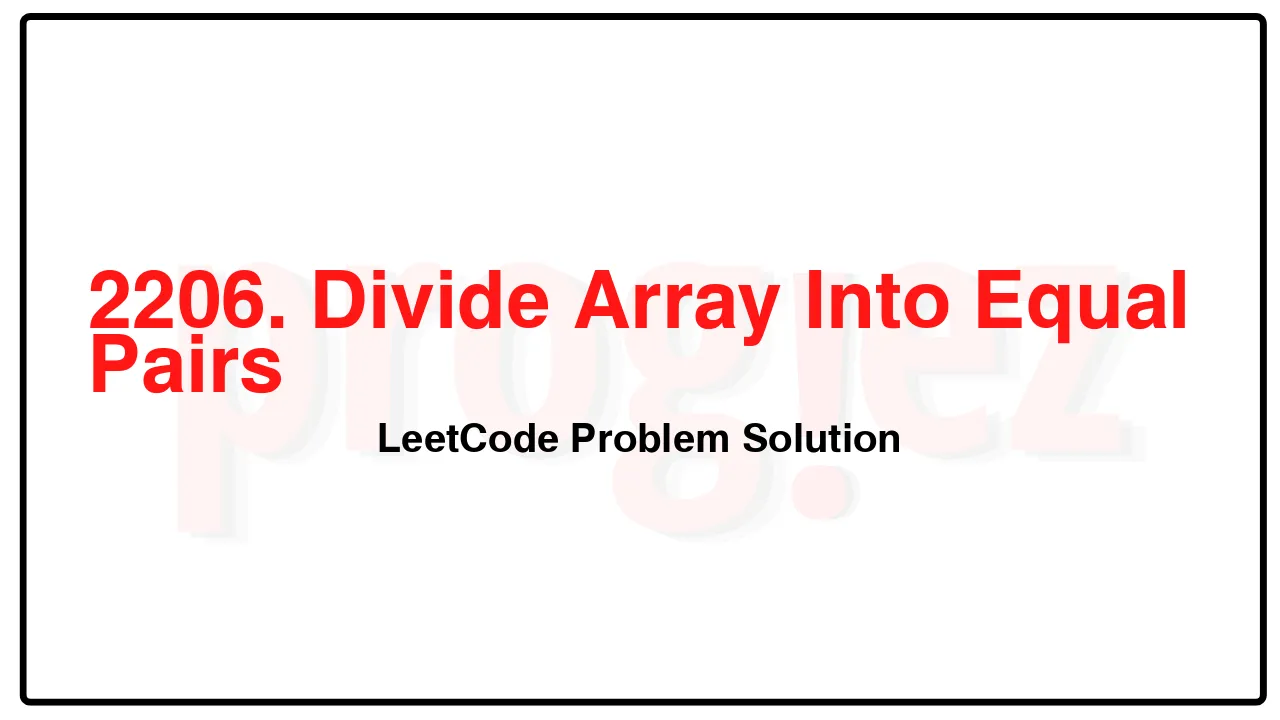
Problem Statement of Divide Array Into Equal Pairs
You are given an integer array nums consisting of 2 * n integers.
You need to divide nums into n pairs such that:
Each element belongs to exactly one pair.
The elements present in a pair are equal.
Return true if nums can be divided into n pairs, otherwise return false.
Example 1:
Input: nums = [3,2,3,2,2,2]
Output: true
Explanation:
There are 6 elements in nums, so they should be divided into 6 / 2 = 3 pairs.
If nums is divided into the pairs (2, 2), (3, 3), and (2, 2), it will satisfy all the conditions.
Example 2:
Input: nums = [1,2,3,4]
Output: false
Explanation:
There is no way to divide nums into 4 / 2 = 2 pairs such that the pairs satisfy every condition.
Constraints:
nums.length == 2 * n
1 <= n <= 500
1 <= nums[i] <= 500
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(500) = O(1)
2206. Divide Array Into Equal Pairs LeetCode Solution in C++
class Solution {
public:
bool divideArray(vector<int>& nums) {
vector<int> count(501);
for (const int num : nums)
++count[num];
return ranges::all_of(count, [](int c) { return c % 2 == 0; });
}
};
/* code provided by PROGIEZ */
2206. Divide Array Into Equal Pairs LeetCode Solution in Java
class Solution {
public boolean divideArray(int[] nums) {
int[] count = new int[501];
for (final int num : nums)
++count[num];
return Arrays.stream(count).allMatch(c -> c % 2 == 0);
}
}
// code provided by PROGIEZ
2206. Divide Array Into Equal Pairs LeetCode Solution in Python
class Solution:
def divideArray(self, nums: list[int]) -> bool:
return all(value % 2 == 0 for value in collections.Counter(nums).values())
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.