2186. Minimum Number of Steps to Make Two Strings Anagram II LeetCode Solution
In this guide, you will get 2186. Minimum Number of Steps to Make Two Strings Anagram II LeetCode Solution with the best time and space complexity. The solution to Minimum Number of Steps to Make Two Strings Anagram II problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Minimum Number of Steps to Make Two Strings Anagram II solution in C++
- Minimum Number of Steps to Make Two Strings Anagram II solution in Java
- Minimum Number of Steps to Make Two Strings Anagram II solution in Python
- Additional Resources
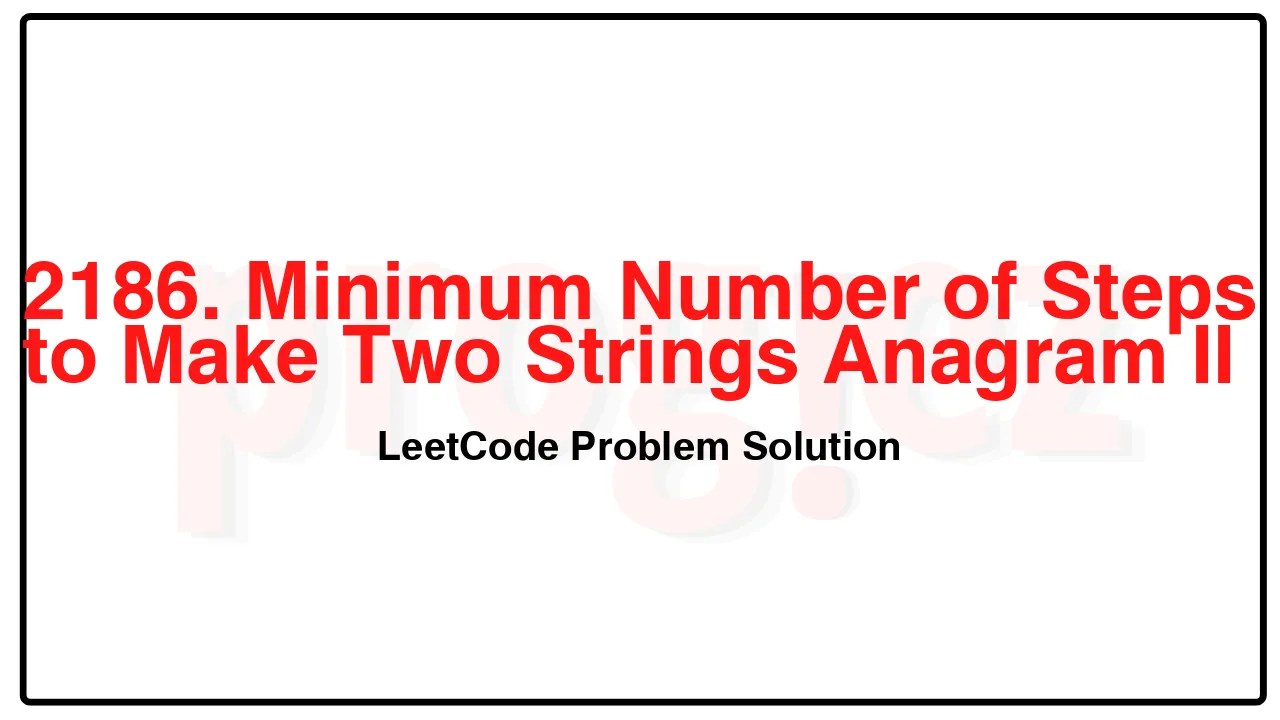
Problem Statement of Minimum Number of Steps to Make Two Strings Anagram II
You are given two strings s and t. In one step, you can append any character to either s or t.
Return the minimum number of steps to make s and t anagrams of each other.
An anagram of a string is a string that contains the same characters with a different (or the same) ordering.
Example 1:
Input: s = “leetcode”, t = “coats”
Output: 7
Explanation:
– In 2 steps, we can append the letters in “as” onto s = “leetcode”, forming s = “leetcodeas”.
– In 5 steps, we can append the letters in “leede” onto t = “coats”, forming t = “coatsleede”.
“leetcodeas” and “coatsleede” are now anagrams of each other.
We used a total of 2 + 5 = 7 steps.
It can be shown that there is no way to make them anagrams of each other with less than 7 steps.
Example 2:
Input: s = “night”, t = “thing”
Output: 0
Explanation: The given strings are already anagrams of each other. Thus, we do not need any further steps.
Constraints:
1 <= s.length, t.length <= 2 * 105
s and t consist of lowercase English letters.
Complexity Analysis
- Time Complexity: O(|\texttt{s}| + |\texttt{t}|)
- Space Complexity: O(26) = O(1)
2186. Minimum Number of Steps to Make Two Strings Anagram II LeetCode Solution in C++
class Solution {
public:
int minSteps(string s, string t) {
vector<int> count(26);
for (const char c : s)
++count[c - 'a'];
for (const char c : t)
--count[c - 'a'];
return accumulate(count.begin(), count.end(), 0,
[](int subtotal, int c) { return subtotal + abs(c); });
}
};
/* code provided by PROGIEZ */
2186. Minimum Number of Steps to Make Two Strings Anagram II LeetCode Solution in Java
class Solution {
public int minSteps(String s, String t) {
int[] count = new int[26];
s.chars().forEach(c -> ++count[c - 'a']);
t.chars().forEach(c -> --count[c - 'a']);
return Arrays.stream(count).map(Math::abs).sum();
}
}
// code provided by PROGIEZ
2186. Minimum Number of Steps to Make Two Strings Anagram II LeetCode Solution in Python
class Solution:
def minSteps(self, s: str, t: str) -> int:
count = collections.Counter(s)
count.subtract(collections.Counter(t))
return sum([abs(c) for c in count.values()])
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.