1894. Find the Student that Will Replace the Chalk LeetCode Solution
In this guide, you will get 1894. Find the Student that Will Replace the Chalk LeetCode Solution with the best time and space complexity. The solution to Find the Student that Will Replace the Chalk problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Find the Student that Will Replace the Chalk solution in C++
- Find the Student that Will Replace the Chalk solution in Java
- Find the Student that Will Replace the Chalk solution in Python
- Additional Resources
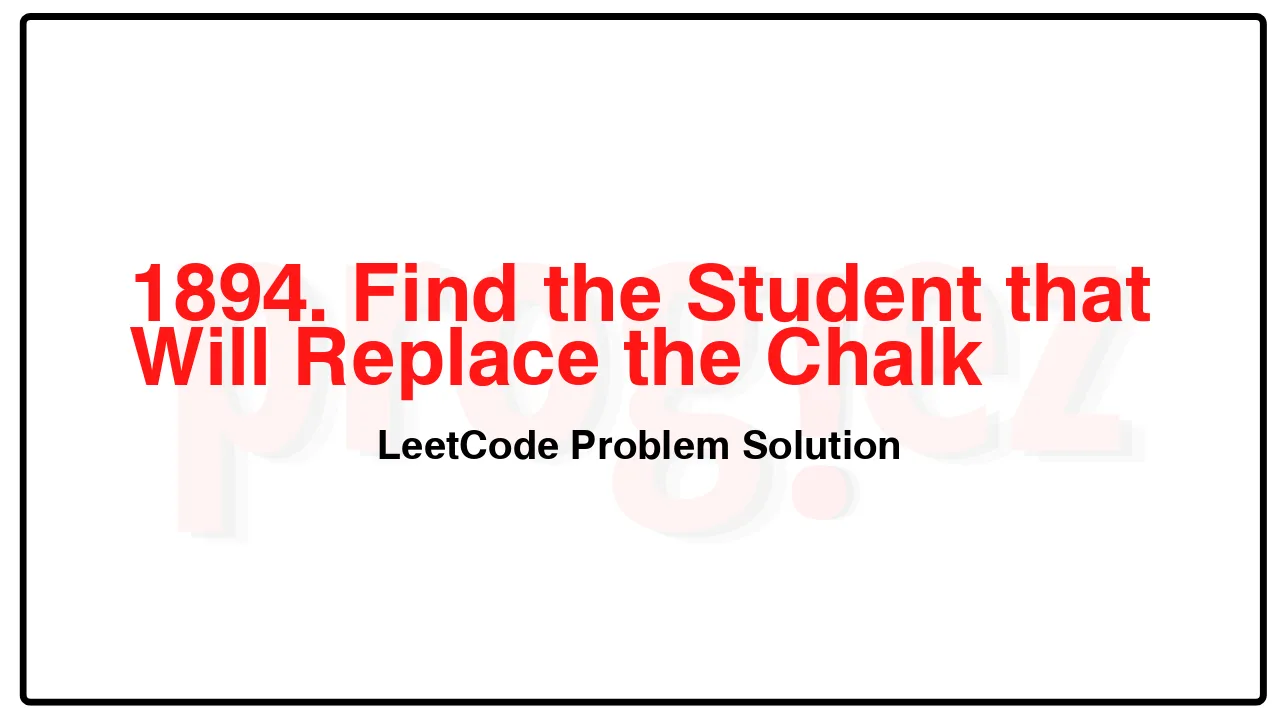
Problem Statement of Find the Student that Will Replace the Chalk
There are n students in a class numbered from 0 to n – 1. The teacher will give each student a problem starting with the student number 0, then the student number 1, and so on until the teacher reaches the student number n – 1. After that, the teacher will restart the process, starting with the student number 0 again.
You are given a 0-indexed integer array chalk and an integer k. There are initially k pieces of chalk. When the student number i is given a problem to solve, they will use chalk[i] pieces of chalk to solve that problem. However, if the current number of chalk pieces is strictly less than chalk[i], then the student number i will be asked to replace the chalk.
Return the index of the student that will replace the chalk pieces.
Example 1:
Input: chalk = [5,1,5], k = 22
Output: 0
Explanation: The students go in turns as follows:
– Student number 0 uses 5 chalk, so k = 17.
– Student number 1 uses 1 chalk, so k = 16.
– Student number 2 uses 5 chalk, so k = 11.
– Student number 0 uses 5 chalk, so k = 6.
– Student number 1 uses 1 chalk, so k = 5.
– Student number 2 uses 5 chalk, so k = 0.
Student number 0 does not have enough chalk, so they will have to replace it.
Example 2:
Input: chalk = [3,4,1,2], k = 25
Output: 1
Explanation: The students go in turns as follows:
– Student number 0 uses 3 chalk so k = 22.
– Student number 1 uses 4 chalk so k = 18.
– Student number 2 uses 1 chalk so k = 17.
– Student number 3 uses 2 chalk so k = 15.
– Student number 0 uses 3 chalk so k = 12.
– Student number 1 uses 4 chalk so k = 8.
– Student number 2 uses 1 chalk so k = 7.
– Student number 3 uses 2 chalk so k = 5.
– Student number 0 uses 3 chalk so k = 2.
Student number 1 does not have enough chalk, so they will have to replace it.
Constraints:
chalk.length == n
1 <= n <= 105
1 <= chalk[i] <= 105
1 <= k <= 109
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1);
1894. Find the Student that Will Replace the Chalk LeetCode Solution in C++
class Solution {
public:
int chalkReplacer(vector<int>& chalk, int k) {
k %= accumulate(chalk.begin(), chalk.end(), 0L);
if (k == 0)
return 0;
for (int i = 0; i < chalk.size(); ++i) {
k -= chalk[i];
if (k < 0)
return i;
}
throw;
}
};
/* code provided by PROGIEZ */
1894. Find the Student that Will Replace the Chalk LeetCode Solution in Java
class Solution {
public int chalkReplacer(int[] chalk, int k) {
k %= Arrays.stream(chalk).asLongStream().sum();
if (k == 0)
return 0;
for (int i = 0; i < chalk.length; ++i) {
k -= chalk[i];
if (k < 0)
return i;
}
throw new IllegalArgumentException();
}
}
// code provided by PROGIEZ
1894. Find the Student that Will Replace the Chalk LeetCode Solution in Python
class Solution:
def chalkReplacer(self, chalk: list[int], k: int) -> int:
k %= sum(chalk)
if k == 0:
return 0
for i, c in enumerate(chalk):
k -= c
if k < 0:
return i
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.