1798. Maximum Number of Consecutive Values You Can Make LeetCode Solution
In this guide, you will get 1798. Maximum Number of Consecutive Values You Can Make LeetCode Solution with the best time and space complexity. The solution to Maximum Number of Consecutive Values You Can Make problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Maximum Number of Consecutive Values You Can Make solution in C++
- Maximum Number of Consecutive Values You Can Make solution in Java
- Maximum Number of Consecutive Values You Can Make solution in Python
- Additional Resources
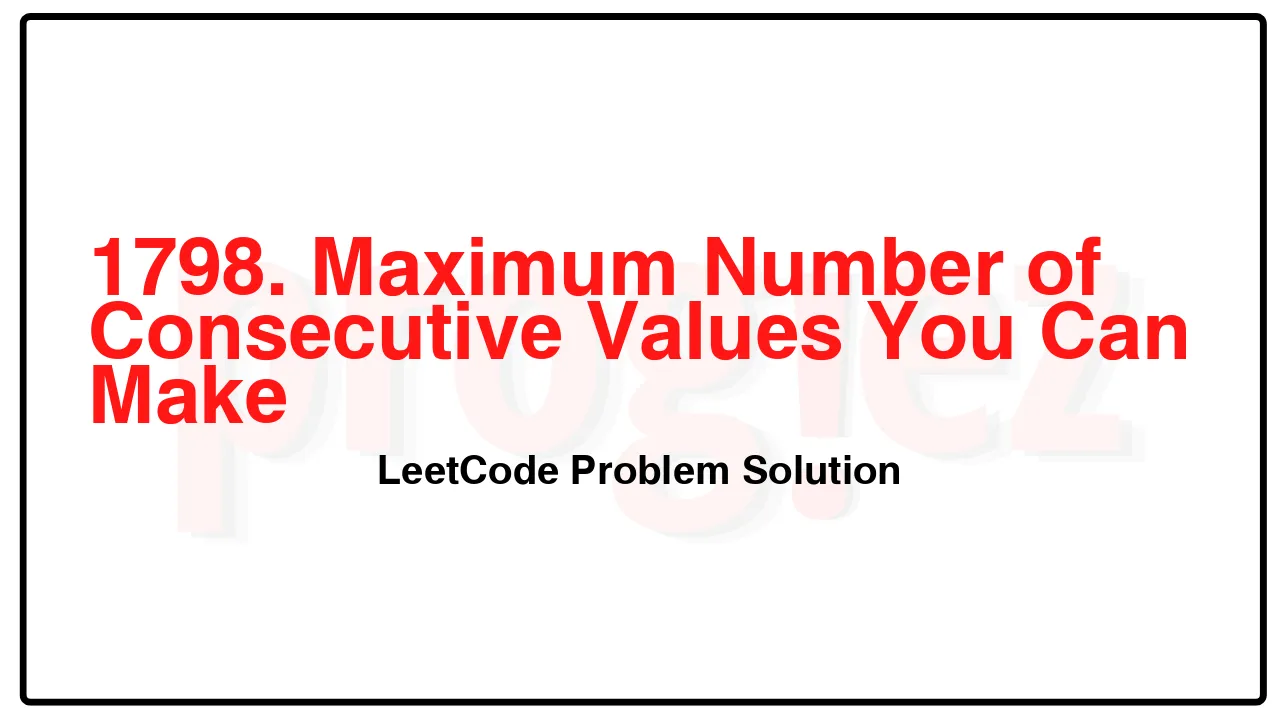
Problem Statement of Maximum Number of Consecutive Values You Can Make
You are given an integer array coins of length n which represents the n coins that you own. The value of the ith coin is coins[i]. You can make some value x if you can choose some of your n coins such that their values sum up to x.
Return the maximum number of consecutive integer values that you can make with your coins starting from and including 0.
Note that you may have multiple coins of the same value.
Example 1:
Input: coins = [1,3]
Output: 2
Explanation: You can make the following values:
– 0: take []
– 1: take [1]
You can make 2 consecutive integer values starting from 0.
Example 2:
Input: coins = [1,1,1,4]
Output: 8
Explanation: You can make the following values:
– 0: take []
– 1: take [1]
– 2: take [1,1]
– 3: take [1,1,1]
– 4: take [4]
– 5: take [4,1]
– 6: take [4,1,1]
– 7: take [4,1,1,1]
You can make 8 consecutive integer values starting from 0.
Example 3:
Input: coins = [1,4,10,3,1]
Output: 20
Constraints:
coins.length == n
1 <= n <= 4 * 104
1 <= coins[i] <= 4 * 104
Complexity Analysis
- Time Complexity: O(\texttt{sort})
- Space Complexity: O(\texttt{sort})
1798. Maximum Number of Consecutive Values You Can Make LeetCode Solution in C++
class Solution {
public:
int getMaximumConsecutive(vector<int>& coins) {
int ans = 1; // the next value we want to make
ranges::sort(coins);
for (const int coin : coins) {
if (coin > ans)
return ans;
ans += coin;
}
return ans;
}
};
/* code provided by PROGIEZ */
1798. Maximum Number of Consecutive Values You Can Make LeetCode Solution in Java
class Solution {
public int getMaximumConsecutive(int[] coins) {
int ans = 1; // the next value we want to make
Arrays.sort(coins);
for (final int coin : coins) {
if (coin > ans)
return ans;
ans += coin;
}
return ans;
}
}
// code provided by PROGIEZ
1798. Maximum Number of Consecutive Values You Can Make LeetCode Solution in Python
class Solution:
def getMaximumConsecutive(self, coins: list[int]) -> int:
ans = 1 # the next value we want to make
for coin in sorted(coins):
if coin > ans:
return ans
ans += coin
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.