1657. Determine if Two Strings Are Close LeetCode Solution
In this guide, you will get 1657. Determine if Two Strings Are Close LeetCode Solution with the best time and space complexity. The solution to Determine if Two Strings Are Close problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Determine if Two Strings Are Close solution in C++
- Determine if Two Strings Are Close solution in Java
- Determine if Two Strings Are Close solution in Python
- Additional Resources
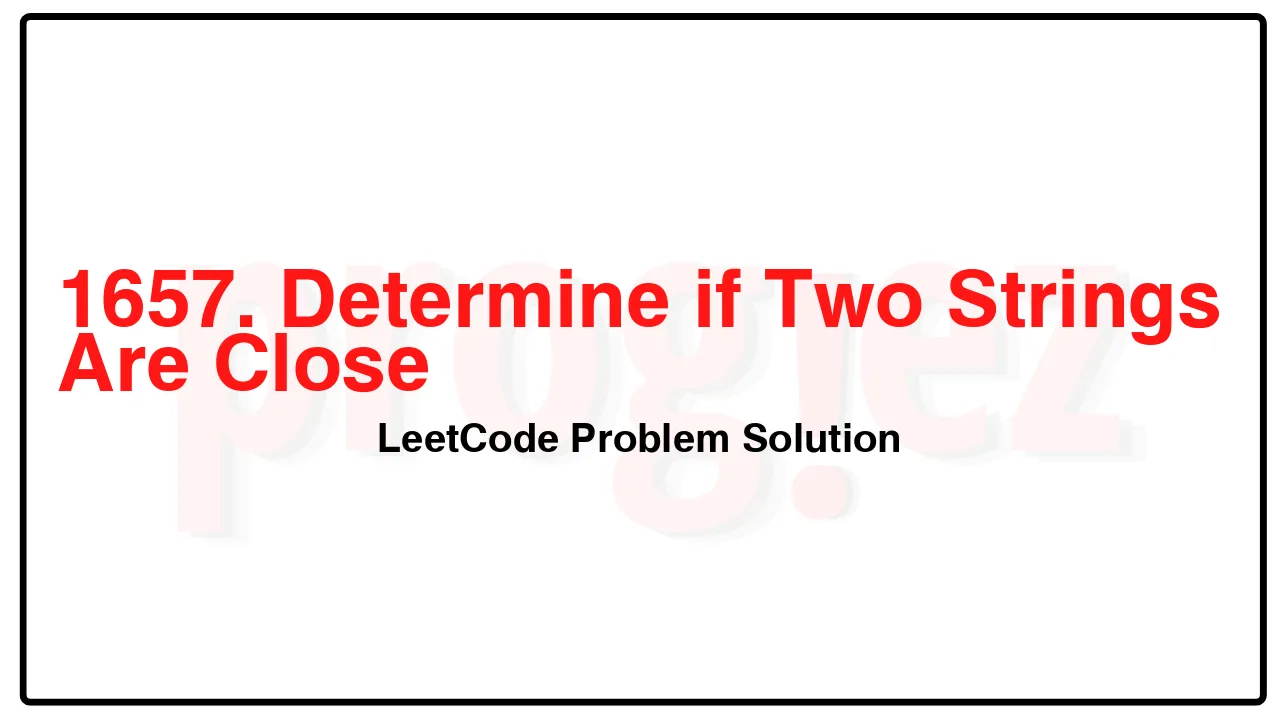
Problem Statement of Determine if Two Strings Are Close
Two strings are considered close if you can attain one from the other using the following operations:
Operation 1: Swap any two existing characters.
For example, abcde -> aecdb
Operation 2: Transform every occurrence of one existing character into another existing character, and do the same with the other character.
For example, aacabb -> bbcbaa (all a’s turn into b’s, and all b’s turn into a’s)
You can use the operations on either string as many times as necessary.
Given two strings, word1 and word2, return true if word1 and word2 are close, and false otherwise.
Example 1:
Input: word1 = “abc”, word2 = “bca”
Output: true
Explanation: You can attain word2 from word1 in 2 operations.
Apply Operation 1: “abc” -> “acb”
Apply Operation 1: “acb” -> “bca”
Example 2:
Input: word1 = “a”, word2 = “aa”
Output: false
Explanation: It is impossible to attain word2 from word1, or vice versa, in any number of operations.
Example 3:
Input: word1 = “cabbba”, word2 = “abbccc”
Output: true
Explanation: You can attain word2 from word1 in 3 operations.
Apply Operation 1: “cabbba” -> “caabbb”
Apply Operation 2: “caabbb” -> “baaccc”
Apply Operation 2: “baaccc” -> “abbccc”
Constraints:
1 <= word1.length, word2.length <= 105
word1 and word2 contain only lowercase English letters.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
1657. Determine if Two Strings Are Close LeetCode Solution in C++
class Solution {
public:
bool closeStrings(string word1, string word2) {
if (word1.length() != word2.length())
return false;
unordered_map<char, int> count1;
unordered_map<char, int> count2;
string s1; // Unique chars in word1
string s2; // Unique chars in word2
vector<int> freqs1; // Freqs of unique chars in word1
vector<int> freqs2; // Freqs of unique chars in word2
for (const char c : word1)
++count1[c];
for (const char c : word2)
++count2[c];
for (const auto& [c, freq] : count1) {
s1 += c;
freqs1.push_back(freq);
}
for (const auto& [c, freq] : count2) {
s2 += c;
freqs2.push_back(freq);
}
ranges::sort(s1);
ranges::sort(s2);
if (s1 != s2)
return false;
ranges::sort(freqs1);
ranges::sort(freqs2);
return freqs1 == freqs2;
}
};
/* code provided by PROGIEZ */
1657. Determine if Two Strings Are Close LeetCode Solution in Java
class Solution {
public boolean closeStrings(String word1, String word2) {
if (word1.length() != word2.length())
return false;
Map<Character, Integer> count1 = new HashMap<>();
Map<Character, Integer> count2 = new HashMap<>();
for (final char c : word1.toCharArray())
count1.merge(c, 1, Integer::sum);
for (final char c : word2.toCharArray())
count2.merge(c, 1, Integer::sum);
if (!count1.keySet().equals(count2.keySet()))
return false;
List<Integer> freqs1 = new ArrayList<>(count1.values());
List<Integer> freqs2 = new ArrayList<>(count2.values());
Collections.sort(freqs1);
Collections.sort(freqs2);
return freqs1.equals(freqs2);
}
}
// code provided by PROGIEZ
1657. Determine if Two Strings Are Close LeetCode Solution in Python
class Solution:
def closeStrings(self, word1: str, word2: str) -> bool:
if len(word1) != len(word2):
return False
count1 = collections.Counter(word1)
count2 = collections.Counter(word2)
if count1.keys() != count2.keys():
return False
return sorted(count1.values()) == sorted(count2.values())
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.