147. Insertion Sort List LeetCode Solution
In this guide, you will get 147. Insertion Sort List LeetCode Solution with the best time and space complexity. The solution to Insertion Sort List problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Insertion Sort List solution in C++
- Insertion Sort List solution in Java
- Insertion Sort List solution in Python
- Additional Resources
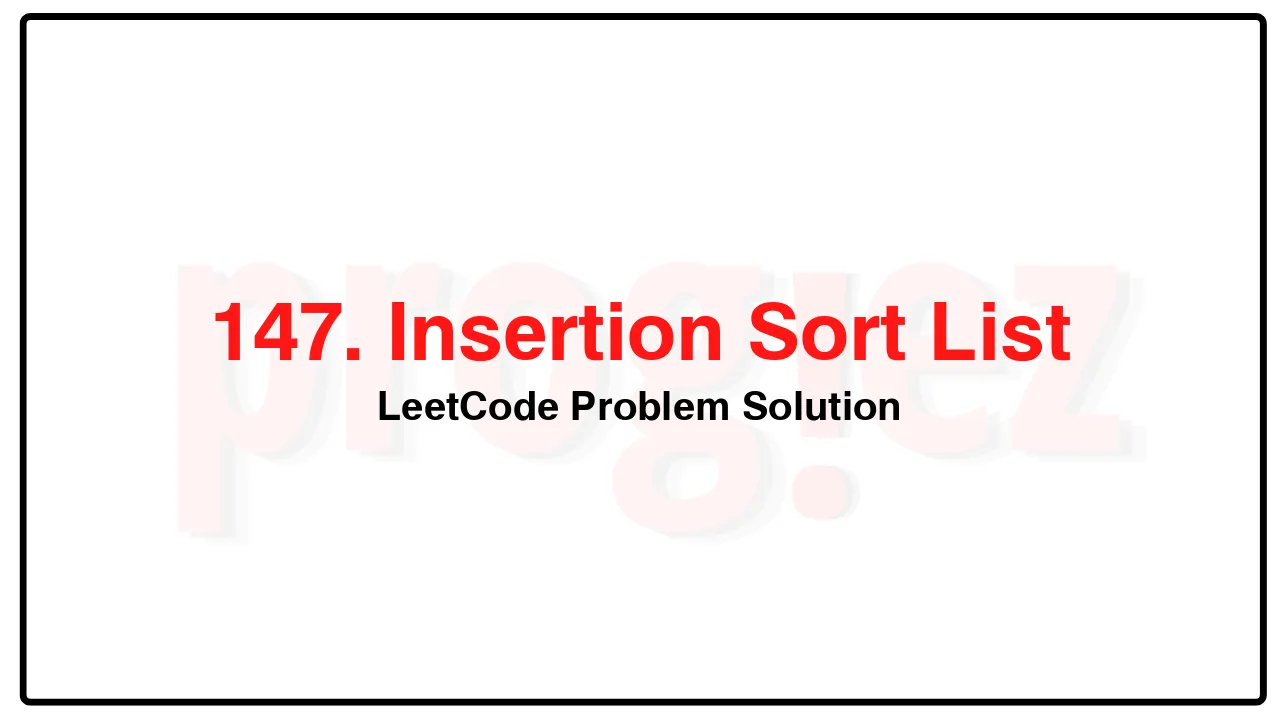
Problem Statement of Insertion Sort List
Given the head of a singly linked list, sort the list using insertion sort, and return the sorted list’s head.
The steps of the insertion sort algorithm:
Insertion sort iterates, consuming one input element each repetition and growing a sorted output list.
At each iteration, insertion sort removes one element from the input data, finds the location it belongs within the sorted list and inserts it there.
It repeats until no input elements remain.
The following is a graphical example of the insertion sort algorithm. The partially sorted list (black) initially contains only the first element in the list. One element (red) is removed from the input data and inserted in-place into the sorted list with each iteration.
Example 1:
Input: head = [4,2,1,3]
Output: [1,2,3,4]
Example 2:
Input: head = [-1,5,3,4,0]
Output: [-1,0,3,4,5]
Constraints:
The number of nodes in the list is in the range [1, 5000].
-5000 <= Node.val <= 5000
Complexity Analysis
- Time Complexity: O(n^2)
- Space Complexity: O(1)
147. Insertion Sort List LeetCode Solution in C++
class Solution {
public:
ListNode* insertionSortList(ListNode* head) {
ListNode dummy(0);
ListNode* prev = &dummy; // the last and thus largest of the sorted list
while (head != nullptr) { // the current inserting node
ListNode* next = head->next; // Cache the next inserting node.
if (prev->val >= head->val)
prev = &dummy; // Move `prev` to the front.
while (prev->next && prev->next->val < head->val)
prev = prev->next;
head->next = prev->next;
prev->next = head;
head = next; // Update the current inserting node.
}
return dummy.next;
}
};
/* code provided by PROGIEZ */
147. Insertion Sort List LeetCode Solution in Java
class Solution {
public ListNode insertionSortList(ListNode head) {
ListNode dummy = new ListNode(0);
ListNode prev = dummy; // the last and thus largest of the sorted list
while (head != null) { // the current inserting node
ListNode next = head.next; // Cache the next inserting node.
if (prev.val >= head.val)
prev = dummy; // Move `prev` to the front.
while (prev.next != null && prev.next.val < head.val)
prev = prev.next;
head.next = prev.next;
prev.next = head;
head = next; // Update the current inserting node.
}
return dummy.next;
}
}
// code provided by PROGIEZ
147. Insertion Sort List LeetCode Solution in Python
class Solution:
def insertionSortList(self, head: ListNode | None) -> ListNode | None:
dummy = ListNode(0)
prev = dummy # the last and thus largest of the sorted list
while head: # the current inserting node
next = head.next # Cache the next inserting node.
if prev.val >= head.val:
prev = dummy # Move `prev` to the front.
while prev.next and prev.next.val < head.val:
prev = prev.next
head.next = prev.next
prev.next = head
head = next # Update the current inserting node.
return dummy.next
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.