144. Binary Tree Preorder Traversal LeetCode Solution
In this guide, you will get 144. Binary Tree Preorder Traversal LeetCode Solution with the best time and space complexity. The solution to Binary Tree Preorder Traversal problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Binary Tree Preorder Traversal solution in C++
- Binary Tree Preorder Traversal solution in Java
- Binary Tree Preorder Traversal solution in Python
- Additional Resources
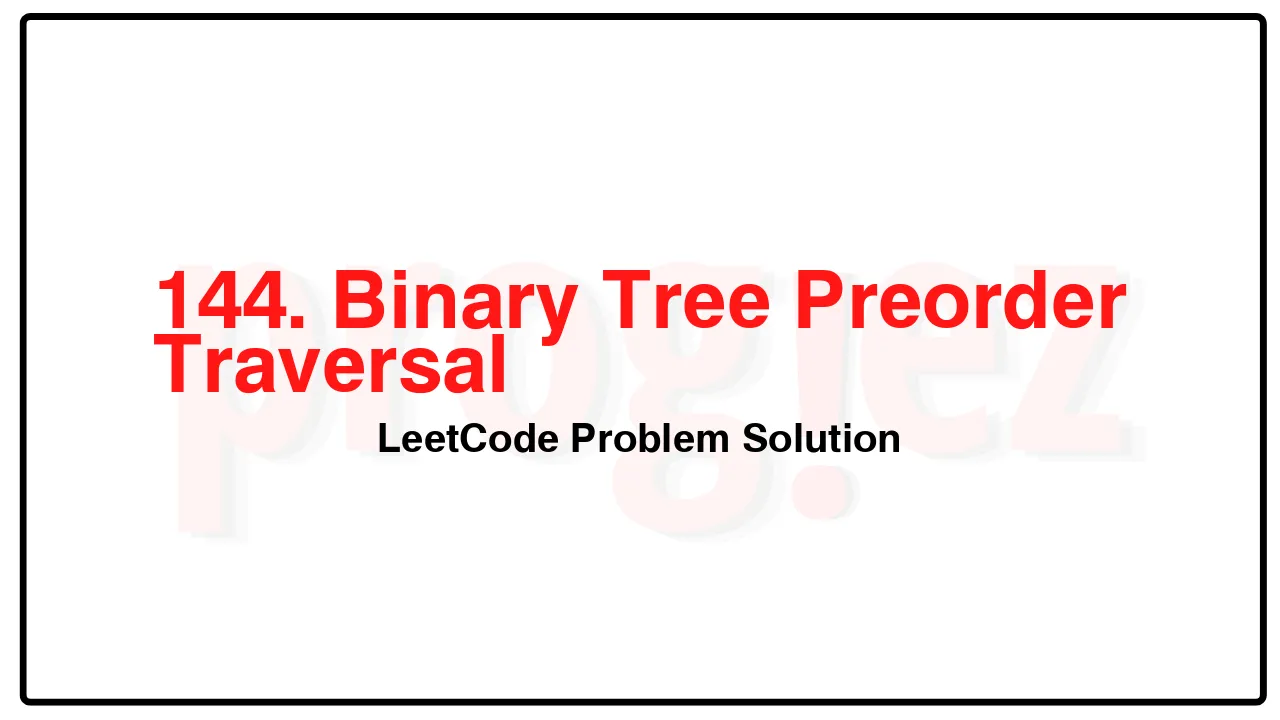
Problem Statement of Binary Tree Preorder Traversal
Given the root of a binary tree, return the preorder traversal of its nodes’ values.
Example 1:
Input: root = [1,null,2,3]
Output: [1,2,3]
Explanation:
Example 2:
Input: root = [1,2,3,4,5,null,8,null,null,6,7,9]
Output: [1,2,4,5,6,7,3,8,9]
Explanation:
Example 3:
Input: root = []
Output: []
Example 4:
Input: root = [1]
Output: [1]
Constraints:
The number of nodes in the tree is in the range [0, 100].
-100 <= Node.val <= 100
Follow up: Recursive solution is trivial, could you do it iteratively?
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(h)
144. Binary Tree Preorder Traversal LeetCode Solution in C++
class Solution {
public:
vector<int> preorderTraversal(TreeNode* root) {
vector<int> ans;
preorder(root, ans);
return ans;
}
private:
void preorder(TreeNode* root, vector<int>& ans) {
if (root == nullptr)
return;
ans.push_back(root->val);
preorder(root->left, ans);
preorder(root->right, ans);
}
};
/* code provided by PROGIEZ */
144. Binary Tree Preorder Traversal LeetCode Solution in Java
class Solution {
public List<Integer> preorderTraversal(TreeNode root) {
List<Integer> ans = new ArrayList<>();
preorder(root, ans);
return ans;
}
private void preorder(TreeNode root, List<Integer> ans) {
if (root == null)
return;
ans.add(root.val);
preorder(root.left, ans);
preorder(root.right, ans);
}
}
// code provided by PROGIEZ
144. Binary Tree Preorder Traversal LeetCode Solution in Python
class Solution:
def preorderTraversal(self, root: TreeNode | None) -> list[int]:
ans = []
def preorder(root: TreeNode | None) -> None:
if not root:
return
ans.append(root.val)
preorder(root.left)
preorder(root.right)
preorder(root)
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.