1124. Longest Well-Performing Interval LeetCode Solution
In this guide, you will get 1124. Longest Well-Performing Interval LeetCode Solution with the best time and space complexity. The solution to Longest Well-Performing Interval problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Longest Well-Performing Interval solution in C++
- Longest Well-Performing Interval solution in Java
- Longest Well-Performing Interval solution in Python
- Additional Resources
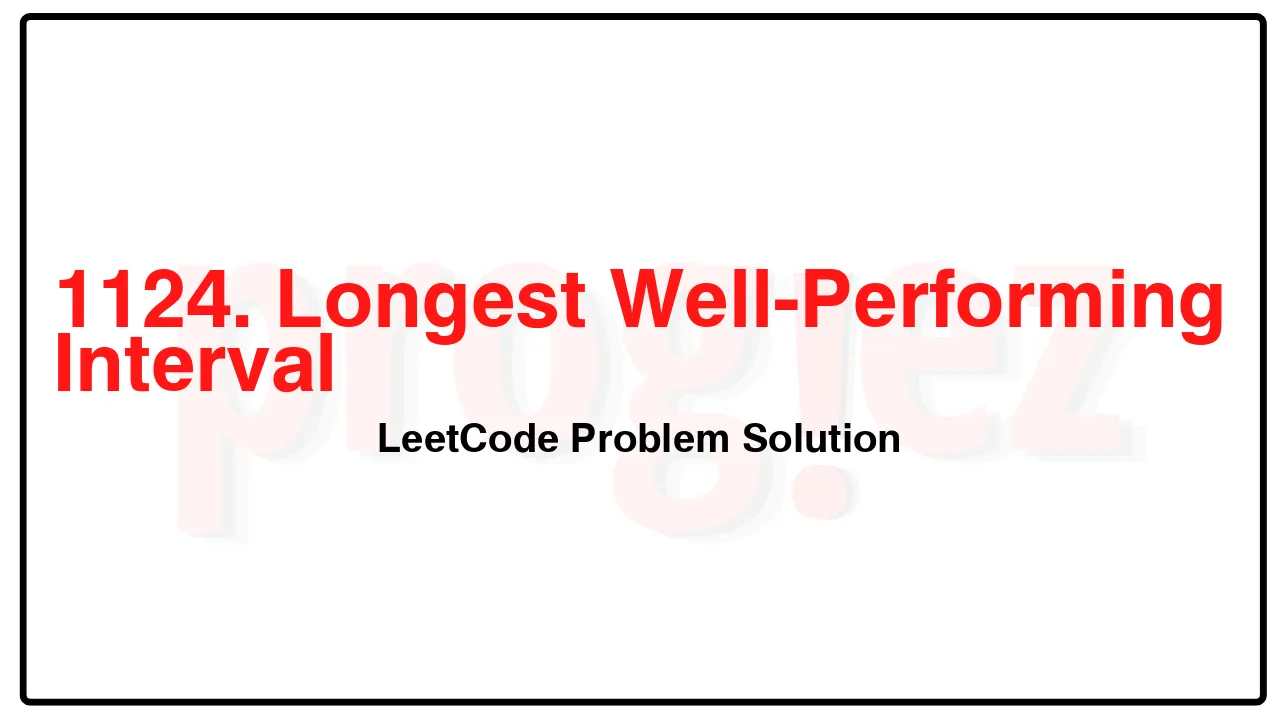
Problem Statement of Longest Well-Performing Interval
We are given hours, a list of the number of hours worked per day for a given employee.
A day is considered to be a tiring day if and only if the number of hours worked is (strictly) greater than 8.
A well-performing interval is an interval of days for which the number of tiring days is strictly larger than the number of non-tiring days.
Return the length of the longest well-performing interval.
Example 1:
Input: hours = [9,9,6,0,6,6,9]
Output: 3
Explanation: The longest well-performing interval is [9,9,6].
Example 2:
Input: hours = [6,6,6]
Output: 0
Constraints:
1 <= hours.length <= 104
0 <= hours[i] <= 16
Complexity Analysis
- Time Complexity:
- Space Complexity:
1124. Longest Well-Performing Interval LeetCode Solution in C++
class Solution {
public:
int longestWPI(vector<int>& hours) {
int ans = 0;
int prefix = 0;
unordered_map<int, int> map;
for (int i = 0; i < hours.size(); ++i) {
prefix += hours[i] > 8 ? 1 : -1;
if (prefix > 0) {
ans = i + 1;
} else {
if (!map.contains(prefix))
map[prefix] = i;
if (const auto it = map.find(prefix - 1); it != map.cend())
ans = max(ans, i - it->second);
}
}
return ans;
}
};
/* code provided by PROGIEZ */
1124. Longest Well-Performing Interval LeetCode Solution in Java
class Solution:
def longestWPI(self, hours: list[int]) -> int:
ans = 0
prefix = 0
dict = {}
for i in range(len(hours)):
prefix += 1 if hours[i] > 8 else -1
if prefix > 0:
ans = i + 1
else:
if prefix not in dict:
dict[prefix] = i
if prefix - 1 in dict:
ans = max(ans, i - dict[prefix - 1])
return ans
// code provided by PROGIEZ
1124. Longest Well-Performing Interval LeetCode Solution in Python
N/A
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.