Programming in Modern C++ Week 7 Assignment Answers
Are you looking for the NPTEL Programming in Modern C++ Week 7 Assignment Answers? You’ve come to the right place! This resource provides comprehensive solutions to all the questions from the Week 7 assignment, helping you navigate through the complexities of modern C++ programming.
Course Link: Click Here
Table of Contents
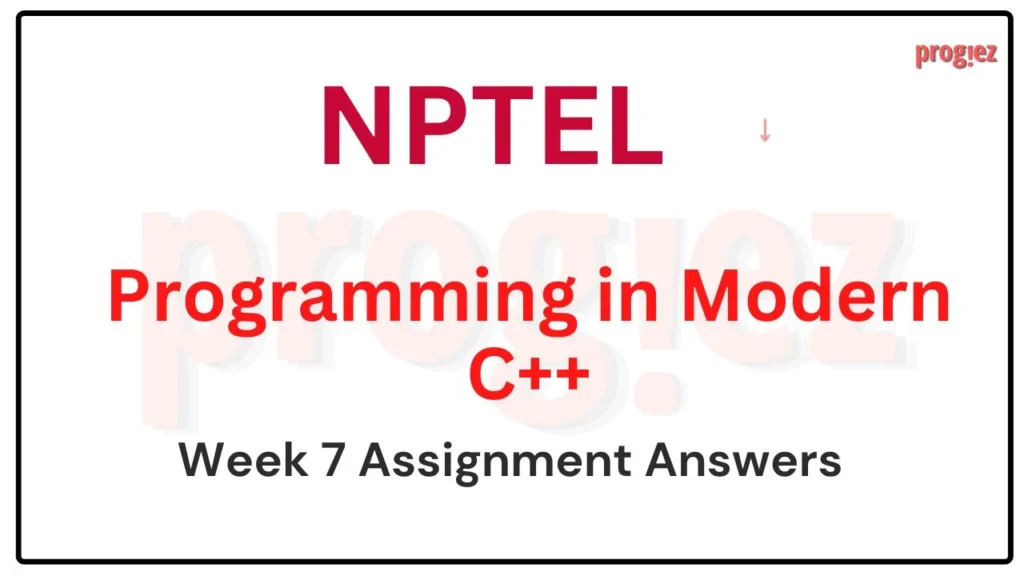
Programming in Modern C++ Week 7 Assignment Answers (July-Dec 2024)
Q1.Fill in the blank at LINE-1 and LINE-2 with the same statement such that the program will
print Ram : 30000.
a) const_cast (this)
b) static_cast (this)
c) dynamic_cast (this)
d) (employees) (this)
Answer: a) const_cast (this)
d) (employees) (this)
Q2 ‘What will be virtual function table (VFT) for the class A3?
a) A3::f(A3* const)
A2::g(A2* const)
A3::h(A3* const)
A2::i(A2* const)
b) A1::f(A1* const)
A2::g(A2 const)
A3::h(A3 const)
A2::i(A2 const)
c) A1::f(A1 const)
A2::g(A2 const)
A2::h(A2 const)
A3::i(A3 const)
d) A1::f(A1 const)
A2::g(A2 const)
A3::h(A3 const)
A3::i(A3* const)
Answer: a) A3::f(A3* const) A2::g(A2* const) A3::h(A3* const) A2::i(A2* const)
For answers or latest updates join our telegram channel: Click here to join
Q3.‘Which line/s will give compilation error?
a) LINE-1
b) LINE-2
c) LINE-3
d) LINE-4
Answer: c) LINE-3
d) LINE-4
Q4.Which of the following type-casting is permissible?
a) tst2 = static_cast(tsti);
b) tst2 = dynamic_cast(tsti);
c) tst2 = reinterpret_cast(tsti);
d) tst2 = const_cast(tstl);
Answer: c) tst2 = reinterpret_cast(tsti);
For answers or latest updates join our telegram channel: Click here to join
Q5.What will be the output?
a) 0101
b) 0111
c) 0110
d) 0010
Answer:b) 0111
Q6. ‘What will be the output?
a) 0101
b) 1010
c) 1100
d) 1011
Answer: c) 1100
For answers or latest updates join our telegram channel: Click here to join
Q7. Fill in the blank at LINE-1 so that the program will print 10.
a) const_cast<double*>
b) static_cast <double*>
c) dynamic_cast <doubles>
d) (const double*)
Answer: a) const_cast<double*>
Q8. Consider the following code segment.
Fill in the blank at LINE-1 so that the program will print “C++”.
a) reinterpret_cast
b) static_cast
c) dynamic_cast
d) (Bs)
Answer: a) reinterpret_cast
d) (Bs)
For answers or latest updates join our telegram channel: Click here to join
Q9.How many virtual function table (VFT) will be created?
a) 1
b) 2
c) 3
d) 4
Answer: c) 3
For answers or latest updates join our telegram channel: Click here to join
For answers to additional Nptel courses, please refer to this link: NPTEL Assignment
Programming in Modern C++ Week 7 Assignment Answers (JAN-APR 2024)
Course Name: Programming in Modern C++
Course Link: Click Here
For answers or latest updates join our telegram channel: Click here to join
Quiz
Q1. Consider the following code segment.
Fill in the blank at LINE-1 such that the program will print 3000 : 50.
a) const_cast > (this)
b) static_cast > (this)
c) dynamic_cast <student> (this)
d) (student) (this)
Answer: a), d)
Q2. Consider the following code segment.
Which line/s will give you error?
a) LINE-1
b) LINE-2
c) LINE-3
d) LINE-4
Answer: c), d)
Q3. Consider the following code segment.
Fill in the blank at LINE-1 such that the program will be compiled successfully.
a) static_cast<Test2*>
b) dynamic_cast<Test2*>
c) reinterpret_cast<Test2*>
d) const_cast<Test2*>
Answer: a), c)
For answers or latest updates join our telegram channel: Click here to join
Q4. Consider the following code segment.
Which of the following type-casting is permissible?
a) t2 = static_cast<Test2*>(t1);
b) t2 = dynamic_cast<Test2*>(t1);
c) t2 = reinterpret_cast<Test2*>(t1);
d) t2 = const_cast<Test2*>(t1);
Answer: c) t2 = reinterpret_cast<Test2*>(t1);
Q5. Consider the following code segment.
What will be the output?
a) 0101
b) 0111
c) 0110
d) 0011
Answer: d) 0011
Q6. Consider the following code segment.
What will be the output?
a) ABAB
b) BABA
c) BBAA
d) BABB
Answer: c) BBAA
For answers or latest updates join our telegram channel: Click here to join
Q7. Consider the following code segment.
What will be the VFT for the class C?
a) A::F(A* const)
C::G(C* const)
C::H(C* const)
B::I(B* const)
b) A::F(A* const)
B::G(B* const)
C::H(C* const)
B::I(B* const)
c) A::F(A* const)
B::G(B* const)
B::H(B* const)
C:: I(C* const)
d) A::F(A* const)
B::G(C* const)
C::H(C* const)
C::I(C* const)
Answer: a)
Q8. Consider the following code segment.
Fill in the blank at LINE-1 so that the program will print “200”.
a) const_cast<int*>
b) static_cast<int*>
c) dynamic_cast<int*>
d) (int*)
Answer: a), d)
Q9. Consider the following code segment.
How many virtual function table (VFT) will be created?
a) 1
b) 2
c) 3
d) 4
Answer: c) 3
For answers or latest updates join our telegram channel: Click here to join
These are Nptel Programming in Modern C++ Assignment 7 Answers
Programming
Question 1
Complete the program with the following instructions.
• Fill in the blank at LINE-1 to complete operator overloading for assignment operator.
• Fill in the blanks at LINE-2 and LINE-3 to complete the type casting statements.
The program must satisfy the given test cases.
Solution:
#include<iostream>
using namespace std;
class Test1{
int a = 10;
public:
void show(){
cout << a ;
}
void operator=(int x){ //LINE-1
a = a + x;
}
};
class Test2 : public Test1{
int b = 20;
public:
void show(){
cout << b << " ";
}
};
void fun(const Test2 &t, int x){
Test2 &u = const_cast<Test2&>(t); //LINE-2
u.show();
Test1 &v = reinterpret_cast<Test1&>(u); //LINE-3
v = x;
v.show();
}
For answers or latest updates join our telegram channel: Click here to join
Question 2
Consider the following program with the following instructions.
• Fill in the blank at LINE-1 to complete constructor definition.
• Fill in the blank at LINE-2 to complete assignment operator overload function signature.
• Fill in the blank at LINE-3 to complete integer cast operator overload function signature. The program must satisfy the sample input and output.
Solution:
#include<iostream>
using namespace std;
class StringClass{
int *arr;
int n;
public:
StringClass(int k) : n(k), arr(new int(n)){} //LINE-1
operator int(){ //LINE-2
return arr[--n] ;
}
StringClass operator=(int k){ //LINE-3
int t;
for(int j = 0; j < k; j++){
cin >> t;
this->arr[j] = t;
}
return *this;
}
};
For answers or latest updates join our telegram channel: Click here to join
Question 3
Consider the following program. Fill in the blanks as per the instructions given below :
• at LINE-1, LINE-2, LINE-3 and LINE-4 with appropriate inheritance statements
such that it will satisfy the given test cases.
Solution:
#include<iostream>
using namespace std;
class B {
public:
B(int i) { cout << 50 * i << " "; }
B() { cout << 1 << " "; }
};
class D1 : virtual public B { //LINE-1
public:
D1(int i);
};
class D2 : virtual public B { //LINE-2
public:
D2(int i);
};
class D3 : virtual public B { //LINE-3
public:
D3(int i);
};
class DD : public D2, public D1, public D3 { //LINE-4
public:
DD (int i) : D1(i), D2(i), D3(i) {
cout << 2 * i << " ";
}
};
For answers or latest updates join our telegram channel: Click here to join
More Weeks of Programming in Modern C++: Click here
More Nptel Courses: Click here
Programming in Modern C++ Week 7 Assignment Answers (July-Dec 2023)
Course Name: Programming in Modern C++
Course Link: Click Here
Programming
Question 1
Consider the following program. Fill in the blanks as per the instructions given below:
• at LINE-1, complete the constructor statement,
• at LINE-2 and LINE-3, complete the operator overloading function header,
such that it will satisfy the given test cases.
Solution:
#include<iostream>
#include<cctype>
using namespace std;
class Char{
char ch;
public:
Char(char _ch) : ch(tolower(_ch)){}
operator char(){ return ch; }
operator int(){ return ch - 'a' + 1; }
};
These are Nptel Programming in Modern C++ Assignment 7 Answers
Question 2
Consider the following program. Fill in the blanks as per the instructions given below.
• at LINE-1, complete the constructor definition,
• at LINE-2, complete the overload function header,
such that it will satisfy the given test cases.
Solution:
#include<iostream>
#include<cstring>
#include<malloc.h>
using namespace std;
class String{
char* _str;
public:
String(char* str) : _str(str){}
operator char*() {
char* t_str = (char*)malloc(sizeof(_str) + 7);
strcpy(t_str, "Coding is ");
strcat(t_str, _str);
return t_str;
}
};
These are Nptel Programming in Modern C++ Assignment 7 Answers
Question 3
Consider the following program. Fill in the blanks as per the instructions given below:
• at LINE-1, complete the overload function header,
• at LINE-2, complete the casting operator statement,
• at LINE-3, complete the casting operator statement,
such that it will satisfy the given test cases.
Solution:
class ClassB{
int b = 20;
public:
void display(){
cout << b;
}
ClassB operator=(int x){ //LINE-1
b = b + x;
}
};
void fun(const ClassA &t, int x){
ClassA &u = const_cast<ClassA&>(t); //LINE-2
u.display();
ClassB &v = reinterpret_cast<ClassB&>(u); //LINE-3
v = x;
v.display();
}
These are Nptel Programming in Modern C++ Assignment 7 Answers
More Weeks of Programming in Modern C++: Click here
More Nptel Courses: Click here
Course Name: Programming in Modern C++
Course Link: Click Here
These are Nptel Programming in Modern C++ Assignment 7 Answers
Quiz
Q1. Consider the following code segment.
Fill in the blank at LINE-1 and LINE-2 with the same statement such that the program will print Rajan : 5000.
a) const_cast (this)
b) static_cast (this)
c) dynamic_cast (this)
d) (employee*) (this)
Answer: a, d
Q2. Consider the following code segment.
What will be virtual function table (VFT) for the class C?
a) C::f(C* const)
B::g(B* const)
C::h(C* const)
B::i(B* const)
b) A::f(A* const)
B::g(B* const)
C::h(C* const)
B::i(B* const)
c) A::f(A* const)
B::g(B* const)
B::h(B* const)
C::i(C* const)
d) A::f(A* const)
B::g(B* const)
C::h(C* const)
C::i(C* const)
Answer: a) C::f(C* const)
B::g(B* const)
C::h(C* const)
B::i(B* const)
These are Nptel Programming in Modern C++ Assignment 7 Answers
Q3. Consider the following code segment.
Which line/s will give compilation error?
a) LINE-1
b) LINE-2
c) LINE-3
d) LINE-4
Answer: c, d
Q4. Consider the following code segment.
Which of the following type-casting is permissible?
a) t2 = static_cast< Test2* >(t1);
b) t2 = dynamic_cast< Test2* >(t1);
c) t2 = reinterpret_cast< Test2* >(t1);
d) t2 = const_cast< Test2* >(t1);
Answer: c) t2 = reinterpret_cast< Test2* >(t1);
These are Nptel Programming in Modern C++ Assignment 7 Answers
Q5. Consider the following code segment.
What will be the output?
a) 0101
b) 0111
c) 0110
d) 0010
Answer: b) 0111
Q6. Consider the following code segment.
What will be the output?
a) 0101
b) 0111
c) 0110
d) 0010
Answer: c) 0110
These are Nptel Programming in Modern C++ Assignment 7 Answers
Q7. Consider the following code segment.
Fill in the blank at LINE-1 so that the program will print 9.81.
a) const_cast< double* >
b) static_cast< double* >
c) dynamic_cast< double* >
d) (const doubles)
Answer: a) const_cast< double* >
Q8. Consider the following code segment.
Fill in the blank at LINE-1 so that the program will print “C++”.
a) reinterpret._cast< C2* >
b) static_cast< C2* >
c) dynamic_cast< C2* >
d) (C2%)
Answer: a, d
These are Nptel Programming in Modern C++ Assignment 7 Answers
Q9. Consider the following code segment below.
How many virtual function table (VFT) will be created?
a) 1
b) 2
c) 3
d) 4
Answer: c) 3
Programming Assignment of Programming in Modern C++
Question 1
Complete the program with the following instructions.
• Fill in the blank at LINE-1 to complete operator overloading for assignment operator.
• Fill in the blanks at LINE-2 and LINE-3 to complete the type casting statements.
The program must satisfy the given test cases.
Solution:
void operator=(int x)
{
b = b + x;
}
};
void fun(const Test1 &t, int x)
{
Test1 &u = const_cast<Test1&>(t);
u.show();
Test2 &v = reinterpret_cast<Test2&>(u);
These are Nptel Programming in Modern C++ Assignment 7 Answers
Question 2
Consider the following program with the following instructions.
• Fill in the blank at LINE-1 to complete constructor definition.
• Fill in the blank at LINE-2 to complete assignment operator overload function signature.
• Fill in the blank at LINE-3 to complete integer cast operator overload function signature.
The program must satisfy the sample input and output.
Solution:
#include<iostream>
using namespace std;
class intString{
int *arr;
int n;
mutable int index;
public:
intString(int k):n(k),arr(new int[k]),index(k-1){}
operator int()const
{
return arr[index--];
}
intString& operator=(int &k){
These are Nptel Programming in Modern C++ Assignment 7 Answers
Question 3
Consider the following program. Fill in the blanks as per the instructions given below:
• at LINE-1 to complete the constructor definition,
• at LINE-2 to complete the function header to overload operator for type-casting to char,
• at LINE-3 to complete the function header to overload operator for type-casting to int,
such that it will satisfy the given test cases.
Solution:
#include<iostream>
#include<cctype>
using namespace std;
class classChar
{
char ch;
public:
classChar(char _ch) : ch(tolower(_ch)){}
operator char() const
{
return ch;
}
operator int() const
{
return ch - 'a' + 1;
}
These are Nptel Programming in Modern C++ Assignment 7 Answers