3447. Assign Elements to Groups with Constraints LeetCode Solution
In this guide, you will get 3447. Assign Elements to Groups with Constraints LeetCode Solution with the best time and space complexity. The solution to Assign Elements to Groups with Constraints problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Assign Elements to Groups with Constraints solution in C++
- Assign Elements to Groups with Constraints solution in Java
- Assign Elements to Groups with Constraints solution in Python
- Additional Resources
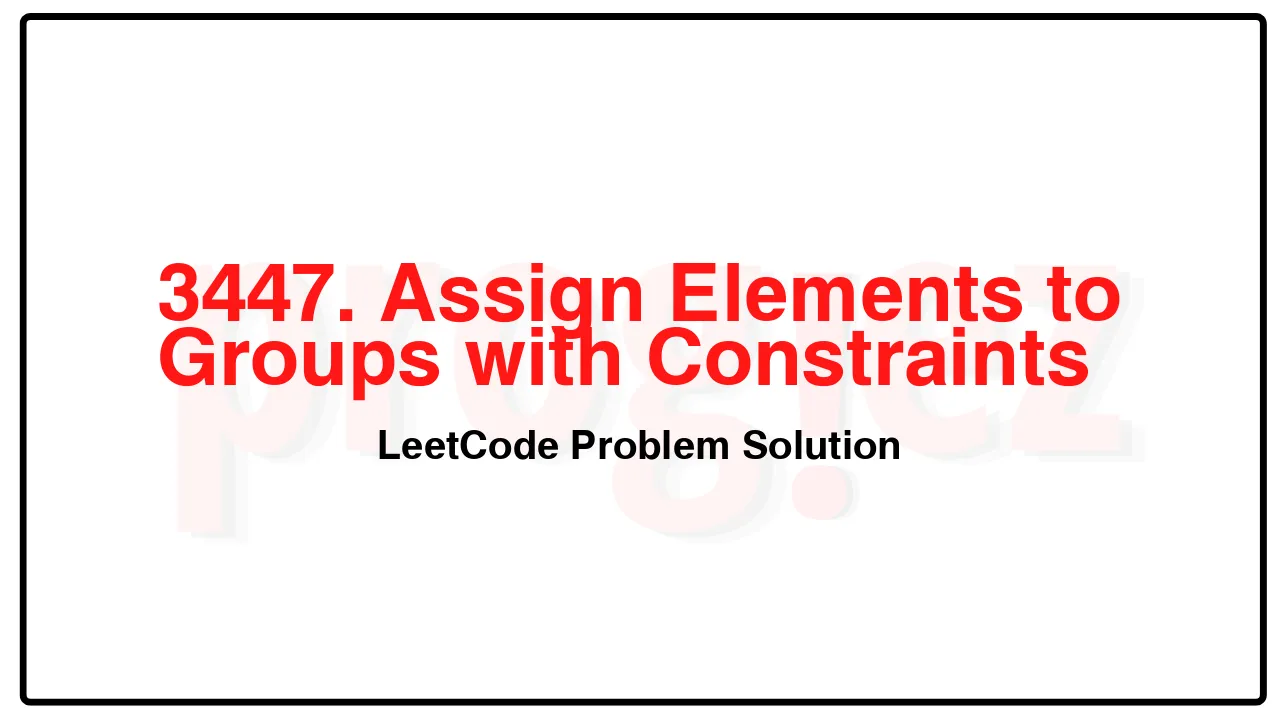
Problem Statement of Assign Elements to Groups with Constraints
You are given an integer array groups, where groups[i] represents the size of the ith group. You are also given an integer array elements.
Your task is to assign one element to each group based on the following rules:
An element at index j can be assigned to a group i if groups[i] is divisible by elements[j].
If there are multiple elements that can be assigned, assign the element with the smallest index j.
If no element satisfies the condition for a group, assign -1 to that group.
Return an integer array assigned, where assigned[i] is the index of the element chosen for group i, or -1 if no suitable element exists.
Note: An element may be assigned to more than one group.
Example 1:
Input: groups = [8,4,3,2,4], elements = [4,2]
Output: [0,0,-1,1,0]
Explanation:
elements[0] = 4 is assigned to groups 0, 1, and 4.
elements[1] = 2 is assigned to group 3.
Group 2 cannot be assigned any element.
Example 2:
Input: groups = [2,3,5,7], elements = [5,3,3]
Output: [-1,1,0,-1]
Explanation:
elements[1] = 3 is assigned to group 1.
elements[0] = 5 is assigned to group 2.
Groups 0 and 3 cannot be assigned any element.
Example 3:
Input: groups = [10,21,30,41], elements = [2,1]
Output: [0,1,0,1]
Explanation:
elements[0] = 2 is assigned to the groups with even values, and elements[1] = 1 is assigned to the groups with odd values.
Constraints:
1 <= groups.length <= 105
1 <= elements.length <= 105
1 <= groups[i] <= 105
1 <= elements[i] <= 105
Complexity Analysis
- Time Complexity: O(n\sqrt{n})
- Space Complexity: O(n)
3447. Assign Elements to Groups with Constraints LeetCode Solution in C++
class Solution {
public:
vector<int> assignElements(vector<int>& groups, vector<int>& elements) {
vector<int> ans;
unordered_map<int, int> elementToMinIndex;
for (int i = 0; i < elements.size(); ++i)
if (!elementToMinIndex.contains(elements[i]))
elementToMinIndex[elements[i]] = i;
for (const int num : groups)
ans.push_back(getMinIndex(num, elementToMinIndex));
return ans;
}
private:
int getMinIndex(int num, const unordered_map<int, int>& elementToMinIndex) {
int res = INT_MAX;
for (int i = 1; i * i <= num; ++i) {
if (num % i != 0)
continue;
if (elementToMinIndex.contains(i))
res = min(res, elementToMinIndex.at(i));
if (num / i != i && elementToMinIndex.contains(num / i))
res = min(res, elementToMinIndex.at(num / i));
}
return res == INT_MAX ? -1 : res;
}
};
/* code provided by PROGIEZ */
3447. Assign Elements to Groups with Constraints LeetCode Solution in Java
class Solution {
public int[] assignElements(int[] groups, int[] elements) {
int[] ans = new int[groups.length];
Map<Integer, Integer> elementToMinIndex = new HashMap<>();
for (int i = 0; i < elements.length; ++i)
elementToMinIndex.putIfAbsent(elements[i], i);
for (int i = 0; i < groups.length; ++i)
ans[i] = getMinIndex(groups[i], elementToMinIndex);
return ans;
}
private int getMinIndex(int num, Map<Integer, Integer> elementToMinIndex) {
int res = Integer.MAX_VALUE;
for (int i = 1; i * i <= num; ++i) {
if (num % i != 0)
continue;
if (elementToMinIndex.containsKey(i))
res = Math.min(res, elementToMinIndex.get(i));
if (num / i != i && elementToMinIndex.containsKey(num / i))
res = Math.min(res, elementToMinIndex.get(num / i));
}
return res == Integer.MAX_VALUE ? -1 : res;
}
}
// code provided by PROGIEZ
3447. Assign Elements to Groups with Constraints LeetCode Solution in Python
class Solution:
def assignElements(self, groups: list[int], elements: list[int]) -> list[int]:
ans = []
elementToMinIndex = {}
for i, element in enumerate(elements):
if element not in elementToMinIndex:
elementToMinIndex[element] = i
for num in groups:
ans.append(self._getMinIndex(num, elementToMinIndex))
return ans
def _getMinIndex(self, num: int, elementToMinIndex: dict[int, int]) -> int:
res = math.inf
i = 1
while i * i <= num:
if num % i != 0:
continue
if i in elementToMinIndex:
res = min(res, elementToMinIndex[i])
if num // i != i and (num // i) in elementToMinIndex:
res = min(res, elementToMinIndex[num // i])
i += 1
return -1 if res == math.inf else res
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.