3259. Maximum Energy Boost From Two Drinks LeetCode Solution
In this guide, you will get 3259. Maximum Energy Boost From Two Drinks LeetCode Solution with the best time and space complexity. The solution to Maximum Energy Boost From Two Drinks problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Maximum Energy Boost From Two Drinks solution in C++
- Maximum Energy Boost From Two Drinks solution in Java
- Maximum Energy Boost From Two Drinks solution in Python
- Additional Resources
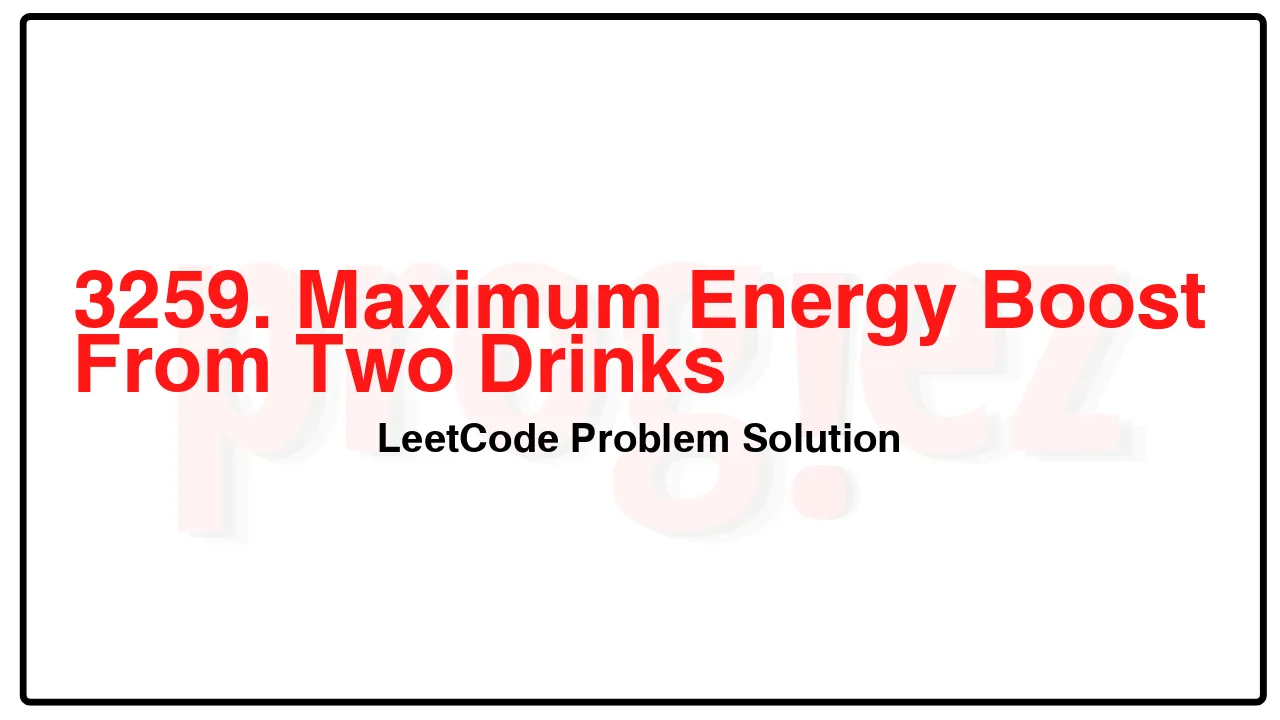
Problem Statement of Maximum Energy Boost From Two Drinks
You are given two integer arrays energyDrinkA and energyDrinkB of the same length n by a futuristic sports scientist. These arrays represent the energy boosts per hour provided by two different energy drinks, A and B, respectively.
You want to maximize your total energy boost by drinking one energy drink per hour. However, if you want to switch from consuming one energy drink to the other, you need to wait for one hour to cleanse your system (meaning you won’t get any energy boost in that hour).
Return the maximum total energy boost you can gain in the next n hours.
Note that you can start consuming either of the two energy drinks.
Example 1:
Input: energyDrinkA = [1,3,1], energyDrinkB = [3,1,1]
Output: 5
Explanation:
To gain an energy boost of 5, drink only the energy drink A (or only B).
Example 2:
Input: energyDrinkA = [4,1,1], energyDrinkB = [1,1,3]
Output: 7
Explanation:
To gain an energy boost of 7:
Drink the energy drink A for the first hour.
Switch to the energy drink B and we lose the energy boost of the second hour.
Gain the energy boost of the drink B in the third hour.
Constraints:
n == energyDrinkA.length == energyDrinkB.length
3 <= n <= 105
1 <= energyDrinkA[i], energyDrinkB[i] <= 105
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
3259. Maximum Energy Boost From Two Drinks LeetCode Solution in C++
class Solution {
public:
long long maxEnergyBoost(vector<int>& energyDrinkA,
vector<int>& energyDrinkB) {
long dpA = 0; // the maximum energy boost if the last drink is A
long dpB = 0; // the maximum energy boost if the last drink is B
for (int i = 0; i < energyDrinkA.size(); ++i) {
const long newDpA = max(dpB, dpA + energyDrinkA[i]);
const long newDpB = max(dpA, dpB + energyDrinkB[i]);
dpA = newDpA;
dpB = newDpB;
}
return max(dpA, dpB);
}
};
/* code provided by PROGIEZ */
3259. Maximum Energy Boost From Two Drinks LeetCode Solution in Java
class Solution {
public long maxEnergyBoost(int[] energyDrinkA, int[] energyDrinkB) {
long dpA = 0; // the maximum energy boost if the last drink is A
long dpB = 0; // the maximum energy boost if the last drink is B
for (int i = 0; i < energyDrinkA.length; ++i) {
final long newDpA = Math.max(dpB, dpA + energyDrinkA[i]);
final long newDpB = Math.max(dpA, dpB + energyDrinkB[i]);
dpA = newDpA;
dpB = newDpB;
}
return Math.max(dpA, dpB);
}
}
// code provided by PROGIEZ
3259. Maximum Energy Boost From Two Drinks LeetCode Solution in Python
class Solution:
def maxEnergyBoost(
self,
energyDrinkA: list[int],
energyDrinkB: list[int]
) -> int:
dpA = 0 # the maximum energy boost if the last drink is A
dpB = 0 # the maximum energy boost if the last drink is B
for a, b in zip(energyDrinkA, energyDrinkB):
dpA, dpB = max(dpB, dpA + a), max(dpA, dpB + b)
return max(dpA, dpB)
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.