2857. Count Pairs of Points With Distance k LeetCode Solution
In this guide, you will get 2857. Count Pairs of Points With Distance k LeetCode Solution with the best time and space complexity. The solution to Count Pairs of Points With Distance k problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Count Pairs of Points With Distance k solution in C++
- Count Pairs of Points With Distance k solution in Java
- Count Pairs of Points With Distance k solution in Python
- Additional Resources
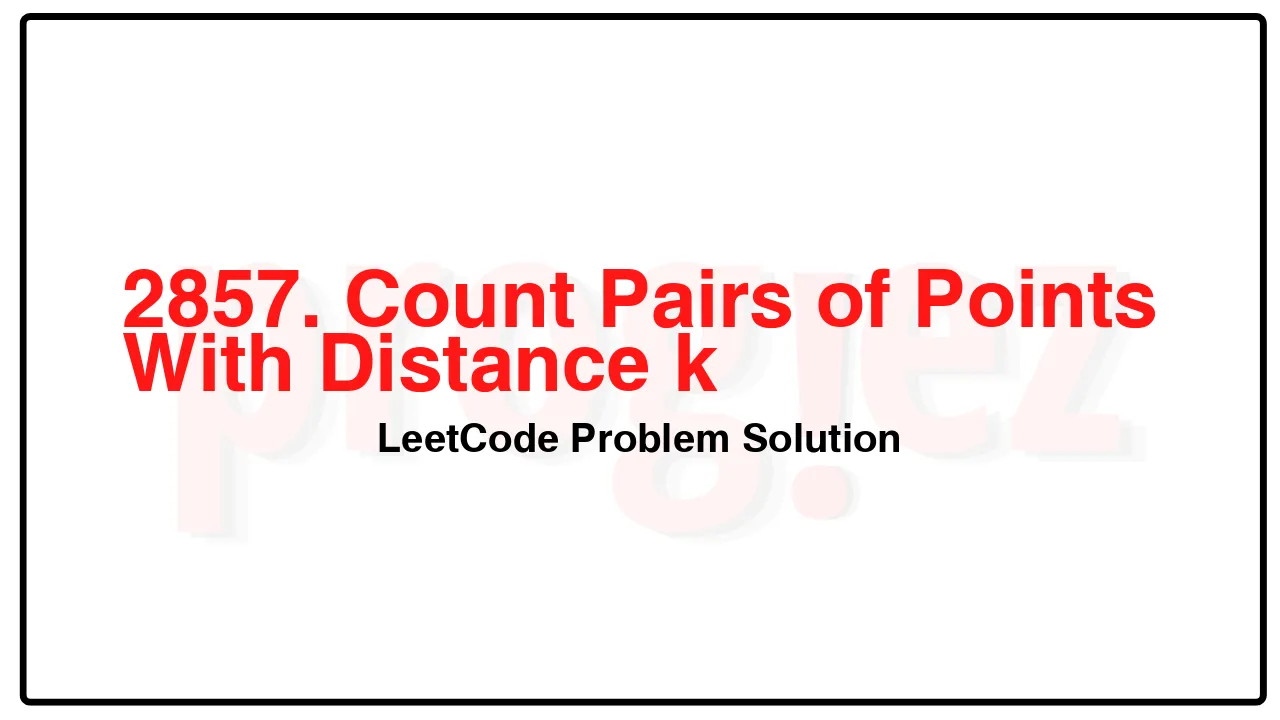
Problem Statement of Count Pairs of Points With Distance k
You are given a 2D integer array coordinates and an integer k, where coordinates[i] = [xi, yi] are the coordinates of the ith point in a 2D plane.
We define the distance between two points (x1, y1) and (x2, y2) as (x1 XOR x2) + (y1 XOR y2) where XOR is the bitwise XOR operation.
Return the number of pairs (i, j) such that i < j and the distance between points i and j is equal to k.
Example 1:
Input: coordinates = [[1,2],[4,2],[1,3],[5,2]], k = 5
Output: 2
Explanation: We can choose the following pairs:
– (0,1): Because we have (1 XOR 4) + (2 XOR 2) = 5.
– (2,3): Because we have (1 XOR 5) + (3 XOR 2) = 5.
Example 2:
Input: coordinates = [[1,3],[1,3],[1,3],[1,3],[1,3]], k = 0
Output: 10
Explanation: Any two chosen pairs will have a distance of 0. There are 10 ways to choose two pairs.
Constraints:
2 <= coordinates.length <= 50000
0 <= xi, yi <= 106
0 <= k <= 100
Complexity Analysis
- Time Complexity: O(kn)
- Space Complexity: O(n)
2857. Count Pairs of Points With Distance k LeetCode Solution in C++
class Solution {
public:
int countPairs(vector<vector<int>>& coordinates, int k) {
int ans = 0;
for (int x = 0; x <= k; ++x) {
const int y = k - x;
unordered_map<pair<int, int>, int, PairHash> count;
for (const vector<int>& point : coordinates) {
const int xi = point[0];
const int yi = point[1];
if (const auto it = count.find({xi ^ x, yi ^ y}); it != count.cend())
ans += it->second;
++count[{xi, yi}];
}
}
return ans;
}
private:
struct PairHash {
size_t operator()(const pair<int, int>& p) const {
return p.first ^ p.second;
}
};
};
/* code provided by PROGIEZ */
2857. Count Pairs of Points With Distance k LeetCode Solution in Java
class Solution {
public int countPairs(List<List<Integer>> coordinates, int k) {
int ans = 0;
for (int x = 0; x <= k; ++x) {
final int y = k - x;
Map<Pair<Integer, Integer>, Integer> count = new HashMap<>();
for (List<Integer> point : coordinates) {
final int xi = point.get(0);
final int yi = point.get(1);
ans += count.getOrDefault(new Pair<>(xi ^ x, yi ^ y), 0);
count.merge(new Pair<>(xi, yi), 1, Integer::sum);
}
}
return ans;
}
}
// code provided by PROGIEZ
2857. Count Pairs of Points With Distance k LeetCode Solution in Python
class Solution:
def countPairs(self, coordinates: list[list[int]], k: int) -> int:
ans = 0
for x in range(k + 1):
y = k - x
count = collections.Counter()
for xi, yi in coordinates:
ans += count[(xi ^ x, yi ^ y)]
count[(xi, yi)] += 1
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.