2828. Check if a String Is an Acronym of Words LeetCode Solution
In this guide, you will get 2828. Check if a String Is an Acronym of Words LeetCode Solution with the best time and space complexity. The solution to Check if a String Is an Acronym of Words problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Check if a String Is an Acronym of Words solution in C++
- Check if a String Is an Acronym of Words solution in Java
- Check if a String Is an Acronym of Words solution in Python
- Additional Resources
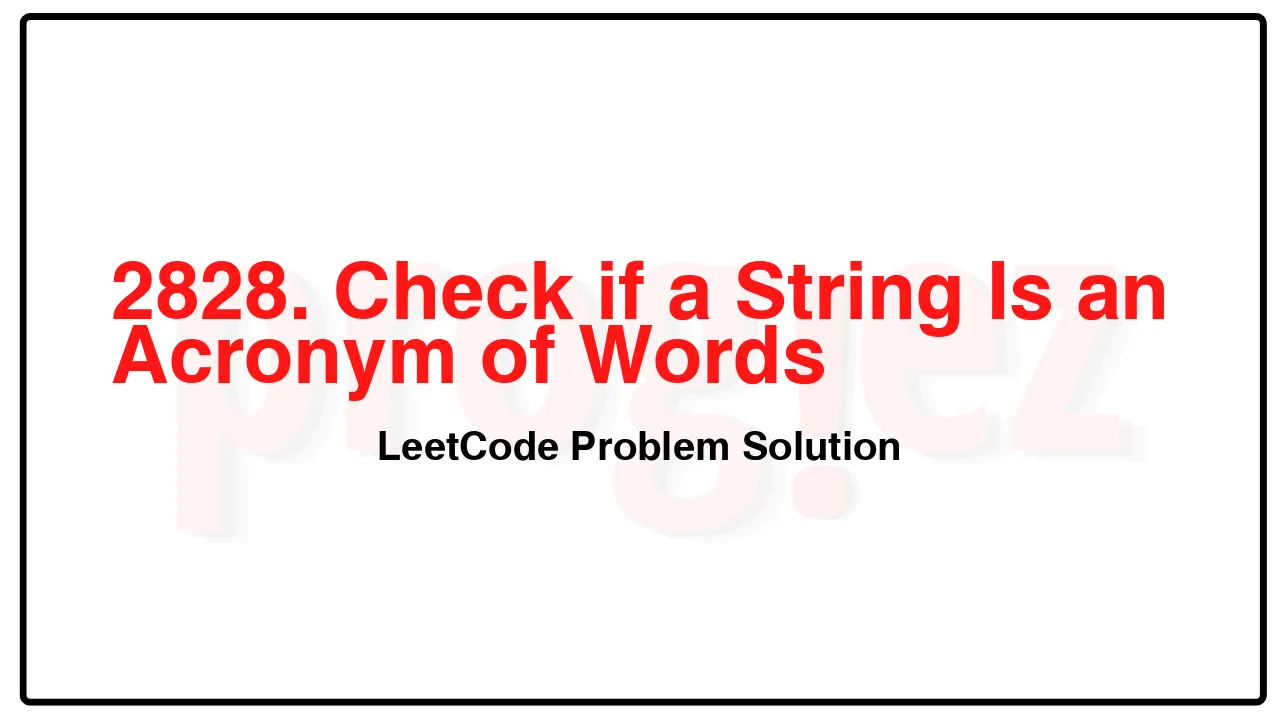
Problem Statement of Check if a String Is an Acronym of Words
Given an array of strings words and a string s, determine if s is an acronym of words.
The string s is considered an acronym of words if it can be formed by concatenating the first character of each string in words in order. For example, “ab” can be formed from [“apple”, “banana”], but it can’t be formed from [“bear”, “aardvark”].
Return true if s is an acronym of words, and false otherwise.
Example 1:
Input: words = [“alice”,”bob”,”charlie”], s = “abc”
Output: true
Explanation: The first character in the words “alice”, “bob”, and “charlie” are ‘a’, ‘b’, and ‘c’, respectively. Hence, s = “abc” is the acronym.
Example 2:
Input: words = [“an”,”apple”], s = “a”
Output: false
Explanation: The first character in the words “an” and “apple” are ‘a’ and ‘a’, respectively.
The acronym formed by concatenating these characters is “aa”.
Hence, s = “a” is not the acronym.
Example 3:
Input: words = [“never”,”gonna”,”give”,”up”,”on”,”you”], s = “ngguoy”
Output: true
Explanation: By concatenating the first character of the words in the array, we get the string “ngguoy”.
Hence, s = “ngguoy” is the acronym.
Constraints:
1 <= words.length <= 100
1 <= words[i].length <= 10
1 <= s.length <= 100
words[i] and s consist of lowercase English letters.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
2828. Check if a String Is an Acronym of Words LeetCode Solution in C++
class Solution {
public:
bool isAcronym(vector<string>& words, string s) {
if (words.size() != s.length())
return false;
for (int i = 0; i < words.size(); ++i)
if (words[i][0] != s[i])
return false;
return true;
}
};
/* code provided by PROGIEZ */
2828. Check if a String Is an Acronym of Words LeetCode Solution in Java
class Solution {
public boolean isAcronym(List<String> words, String s) {
if (words.size() != s.length())
return false;
for (int i = 0; i < words.size(); ++i)
if (words.get(i).charAt(0) != s.charAt(i))
return false;
return true;
}
}
// code provided by PROGIEZ
2828. Check if a String Is an Acronym of Words LeetCode Solution in Python
class Solution:
def isAcronym(self, words: list[str], s: str) -> bool:
return (len(words) == len(s) and
all(word[0] == c for word, c in zip(words, s)))
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.