2811. Check if it is Possible to Split Array LeetCode Solution
In this guide, you will get 2811. Check if it is Possible to Split Array LeetCode Solution with the best time and space complexity. The solution to Check if it is Possible to Split Array problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Check if it is Possible to Split Array solution in C++
- Check if it is Possible to Split Array solution in Java
- Check if it is Possible to Split Array solution in Python
- Additional Resources
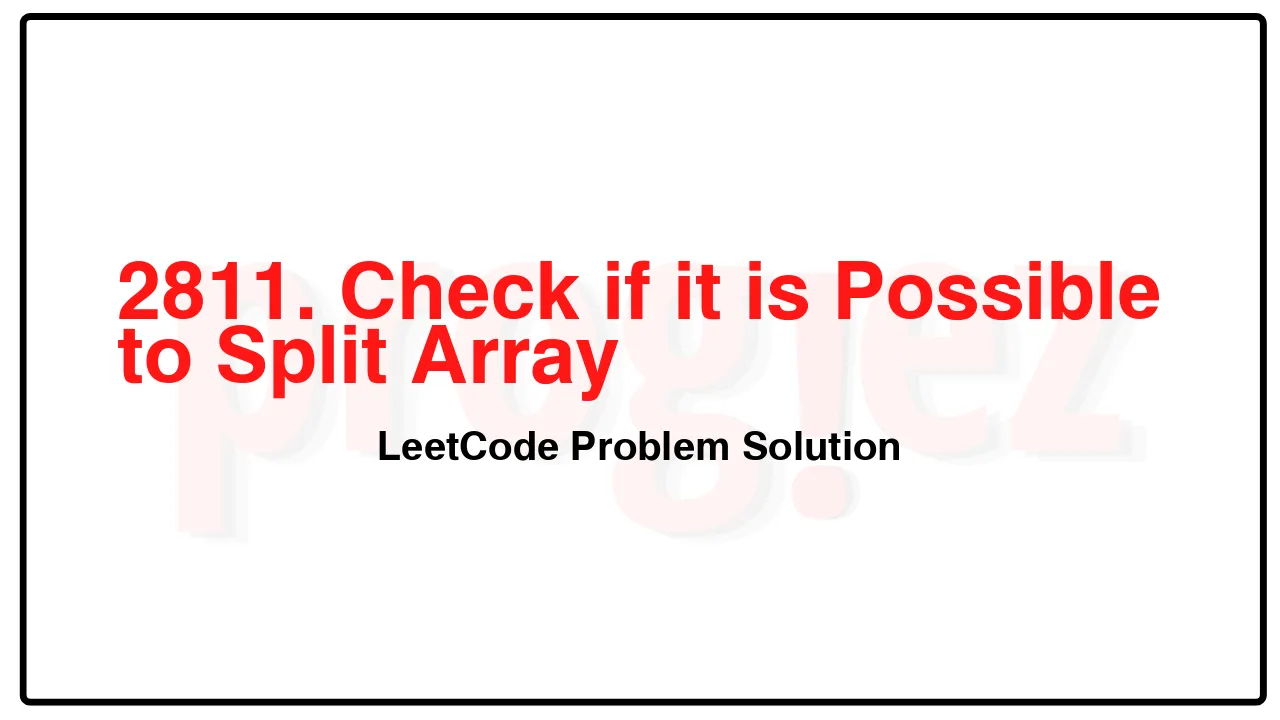
Problem Statement of Check if it is Possible to Split Array
You are given an array nums of length n and an integer m. You need to determine if it is possible to split the array into n arrays of size 1 by performing a series of steps.
An array is called good if:
The length of the array is one, or
The sum of the elements of the array is greater than or equal to m.
In each step, you can select an existing array (which may be the result of previous steps) with a length of at least two and split it into two arrays, if both resulting arrays are good.
Return true if you can split the given array into n arrays, otherwise return false.
Example 1:
Input: nums = [2, 2, 1], m = 4
Output: true
Explanation:
Split [2, 2, 1] to [2, 2] and [1]. The array [1] has a length of one, and the array [2, 2] has the sum of its elements equal to 4 >= m, so both are good arrays.
Split [2, 2] to [2] and [2]. both arrays have the length of one, so both are good arrays.
Example 2:
Input: nums = [2, 1, 3], m = 5
Output: false
Explanation:
The first move has to be either of the following:
Split [2, 1, 3] to [2, 1] and [3]. The array [2, 1] has neither length of one nor sum of elements greater than or equal to m.
Split [2, 1, 3] to [2] and [1, 3]. The array [1, 3] has neither length of one nor sum of elements greater than or equal to m.
So as both moves are invalid (they do not divide the array into two good arrays), we are unable to split nums into n arrays of size 1.
Example 3:
Input: nums = [2, 3, 3, 2, 3], m = 6
Output: true
Explanation:
Split [2, 3, 3, 2, 3] to [2] and [3, 3, 2, 3].
Split [3, 3, 2, 3] to [3, 3, 2] and [3].
Split [3, 3, 2] to [3, 3] and [2].
Split [3, 3] to [3] and [3].
Constraints:
1 <= n == nums.length <= 100
1 <= nums[i] <= 100
1 <= m <= 200
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
2811. Check if it is Possible to Split Array LeetCode Solution in C++
class Solution {
public:
bool canSplitArray(vector<int>& nums, int m) {
if (nums.size() < 3)
return true;
for (int i = 1; i < nums.size(); ++i)
if (nums[i] + nums[i - 1] >= m)
return true;
return false;
}
};
/* code provided by PROGIEZ */
2811. Check if it is Possible to Split Array LeetCode Solution in Java
class Solution {
public boolean canSplitArray(List<Integer> nums, int m) {
if (nums.size() < 3)
return true;
for (int i = 1; i < nums.size(); ++i)
if (nums.get(i) + nums.get(i - 1) >= m)
return true;
return false;
}
}
// code provided by PROGIEZ
2811. Check if it is Possible to Split Array LeetCode Solution in Python
class Solution:
def canSplitArray(self, nums: list[int], m: int) -> bool:
return len(nums) < 3 or any(a + b >= m for a, b in itertools.pairwise(nums))
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.