2470. Number of Subarrays With LCM Equal to K LeetCode Solution
In this guide, you will get 2470. Number of Subarrays With LCM Equal to K LeetCode Solution with the best time and space complexity. The solution to Number of Subarrays With LCM Equal to K problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Number of Subarrays With LCM Equal to K solution in C++
- Number of Subarrays With LCM Equal to K solution in Java
- Number of Subarrays With LCM Equal to K solution in Python
- Additional Resources
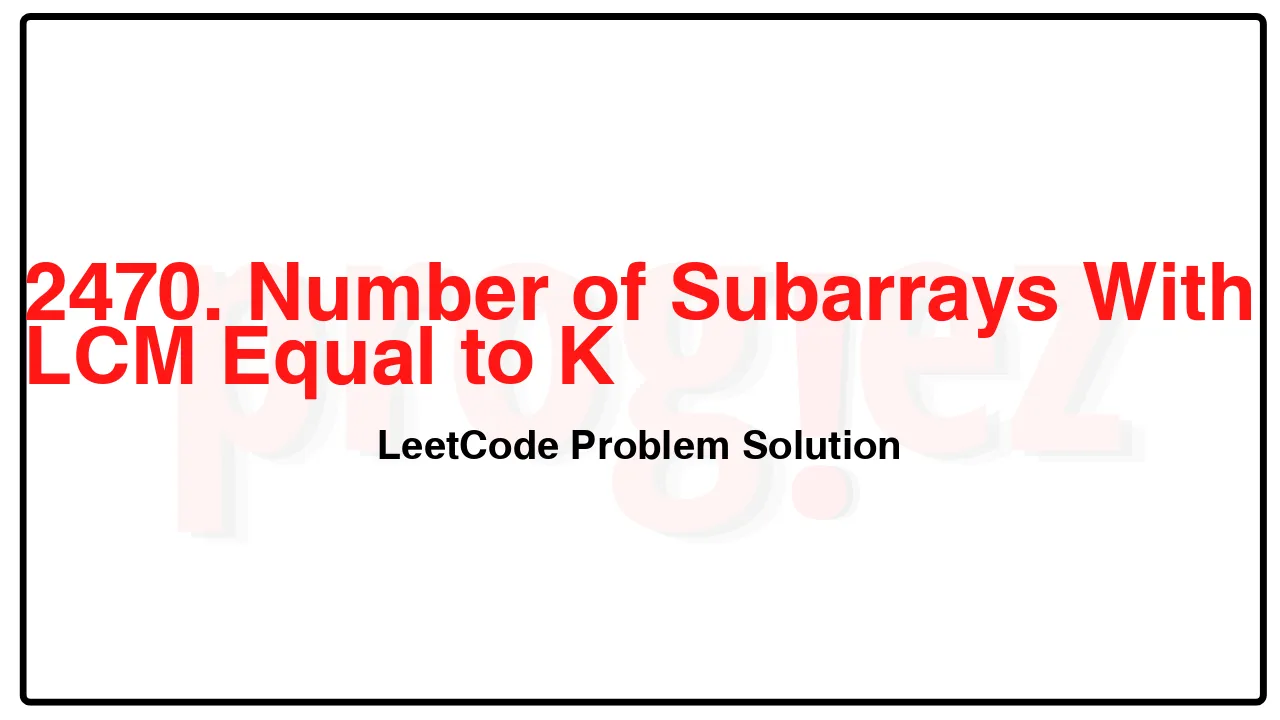
Problem Statement of Number of Subarrays With LCM Equal to K
Given an integer array nums and an integer k, return the number of subarrays of nums where the least common multiple of the subarray’s elements is k.
A subarray is a contiguous non-empty sequence of elements within an array.
The least common multiple of an array is the smallest positive integer that is divisible by all the array elements.
Example 1:
Input: nums = [3,6,2,7,1], k = 6
Output: 4
Explanation: The subarrays of nums where 6 is the least common multiple of all the subarray’s elements are:
– [3,6,2,7,1]
– [3,6,2,7,1]
– [3,6,2,7,1]
– [3,6,2,7,1]
Example 2:
Input: nums = [3], k = 2
Output: 0
Explanation: There are no subarrays of nums where 2 is the least common multiple of all the subarray’s elements.
Constraints:
1 <= nums.length <= 1000
1 <= nums[i], k <= 1000
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
2470. Number of Subarrays With LCM Equal to K LeetCode Solution in C++
class Solution {
public:
int subarrayLCM(vector<int>& nums, int k) {
int ans = 0;
for (int i = 0; i < nums.size(); ++i) {
int runningLcm = nums[i];
for (int j = i; j < nums.size(); ++j) {
runningLcm = std::lcm(runningLcm, nums[j]);
if (runningLcm > k)
break;
if (runningLcm == k)
++ans;
}
}
return ans;
}
};
/* code provided by PROGIEZ */
2470. Number of Subarrays With LCM Equal to K LeetCode Solution in Java
class Solution {
public int subarrayLCM(int[] nums, int k) {
int ans = 0;
for (int i = 0; i < nums.length; ++i) {
int runningLcm = nums[i];
for (int j = i; j < nums.length; ++j) {
runningLcm = lcm(runningLcm, nums[j]);
if (runningLcm > k)
break;
if (runningLcm == k)
++ans;
}
}
return ans;
}
private int gcd(int a, int b) {
return b == 0 ? a : gcd(b, a % b);
}
private int lcm(int a, int b) {
return a * (b / gcd(a, b));
}
}
// code provided by PROGIEZ
2470. Number of Subarrays With LCM Equal to K LeetCode Solution in Python
class Solution:
def subarrayLCM(self, nums: list[int], k: int) -> int:
ans = 0
for i, runningLcm in enumerate(nums):
for j in range(i, len(nums)):
runningLcm = math.lcm(runningLcm, nums[j])
if runningLcm > k:
break
if runningLcm == k:
ans += 1
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.