2384. Largest Palindromic Number LeetCode Solution
In this guide, you will get 2384. Largest Palindromic Number LeetCode Solution with the best time and space complexity. The solution to Largest Palindromic Number problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Largest Palindromic Number solution in C++
- Largest Palindromic Number solution in Java
- Largest Palindromic Number solution in Python
- Additional Resources
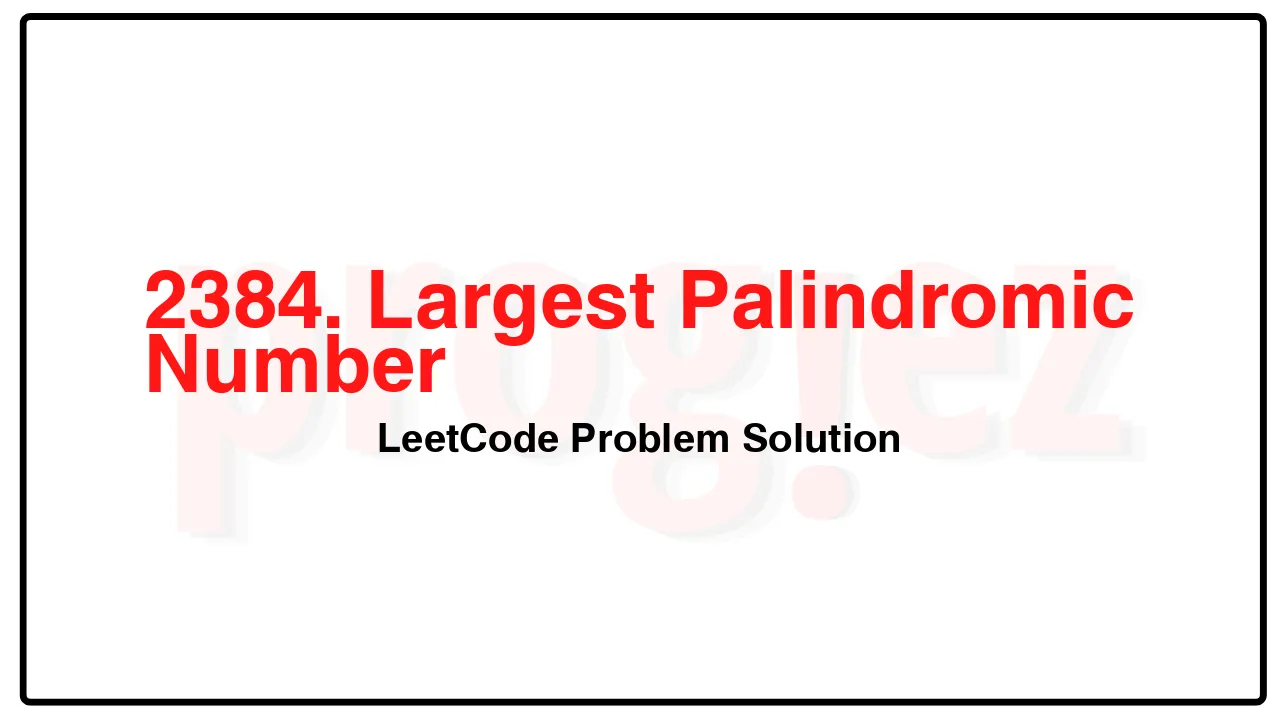
Problem Statement of Largest Palindromic Number
You are given a string num consisting of digits only.
Return the largest palindromic integer (in the form of a string) that can be formed using digits taken from num. It should not contain leading zeroes.
Notes:
You do not need to use all the digits of num, but you must use at least one digit.
The digits can be reordered.
Example 1:
Input: num = “444947137”
Output: “7449447”
Explanation:
Use the digits “4449477” from “444947137” to form the palindromic integer “7449447”.
It can be shown that “7449447” is the largest palindromic integer that can be formed.
Example 2:
Input: num = “00009”
Output: “9”
Explanation:
It can be shown that “9” is the largest palindromic integer that can be formed.
Note that the integer returned should not contain leading zeroes.
Constraints:
1 <= num.length <= 105
num consists of digits.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
2384. Largest Palindromic Number LeetCode Solution in C++
class Solution {
public:
string largestPalindromic(string num) {
unordered_map<char, int> count;
for (const char c : num)
++count[c];
const string firstHalf = getFirstHalf(count);
const string mid = getMid(count);
const string ans = firstHalf + mid + reversed(firstHalf);
return ans.empty() ? "0" : ans;
}
private:
string getFirstHalf(const unordered_map<char, int>& count) {
string firstHalf;
for (char c = '9'; c >= '0'; --c) {
const auto it = count.find(c);
if (it == count.cend())
continue;
const int freq = it->second;
firstHalf += string(freq / 2, c);
}
const int index = firstHalf.find_first_not_of('0');
return index == string::npos ? "" : firstHalf.substr(index);
}
string getMid(const unordered_map<char, int>& count) {
for (char c = '9'; c >= '0'; --c) {
const auto it = count.find(c);
if (it == count.cend())
continue;
const int freq = it->second;
if (freq % 2 == 1)
return string(1, c);
}
return "";
}
string reversed(const string& s) {
return {s.rbegin(), s.rend()};
}
};
/* code provided by PROGIEZ */
2384. Largest Palindromic Number LeetCode Solution in Java
class Solution {
public String largestPalindromic(String num) {
Map<Character, Integer> count = new HashMap<>();
for (final char c : num.toCharArray())
count.merge(c, 1, Integer::sum);
final String firstHalf = getFirstHalf(count);
final String mid = getMid(count);
final String ans = firstHalf + mid + reversed(firstHalf);
return ans.isEmpty() ? "0" : ans;
}
private String getFirstHalf(Map<Character, Integer> count) {
StringBuilder sb = new StringBuilder();
for (char c = '9'; c >= '0'; --c) {
final int freq = count.getOrDefault(c, 0);
sb.append(String.valueOf(c).repeat(freq / 2));
}
final int index = firstNotZeroIndex(sb);
return index == -1 ? "" : sb.substring(index);
}
private int firstNotZeroIndex(StringBuilder sb) {
for (int i = 0; i < sb.length(); ++i)
if (sb.charAt(i) != '0')
return i;
return -1;
}
private String getMid(Map<Character, Integer> count) {
StringBuilder sb = new StringBuilder();
for (char c = '9'; c >= '0'; --c) {
final int freq = count.getOrDefault(c, 0);
if (freq % 2 == 1)
return String.valueOf(c);
}
return "";
}
private String reversed(final String s) {
return new StringBuilder(s).reverse().toString();
}
}
// code provided by PROGIEZ
2384. Largest Palindromic Number LeetCode Solution in Python
class Solution:
def largestPalindromic(self, num: str) -> str:
count = collections.Counter(num)
firstHalf = ''.join(count[i] // 2 * i for i in '9876543210').lstrip('0')
mid = self._getMid(count)
return (firstHalf + mid + firstHalf[::-1]) or '0'
def _getMid(self, count: dict[str, int]) -> str:
for c in '9876543210':
if count[c] & 1:
return c
return ''
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.