2270. Number of Ways to Split Array LeetCode Solution
In this guide, you will get 2270. Number of Ways to Split Array LeetCode Solution with the best time and space complexity. The solution to Number of Ways to Split Array problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Number of Ways to Split Array solution in C++
- Number of Ways to Split Array solution in Java
- Number of Ways to Split Array solution in Python
- Additional Resources
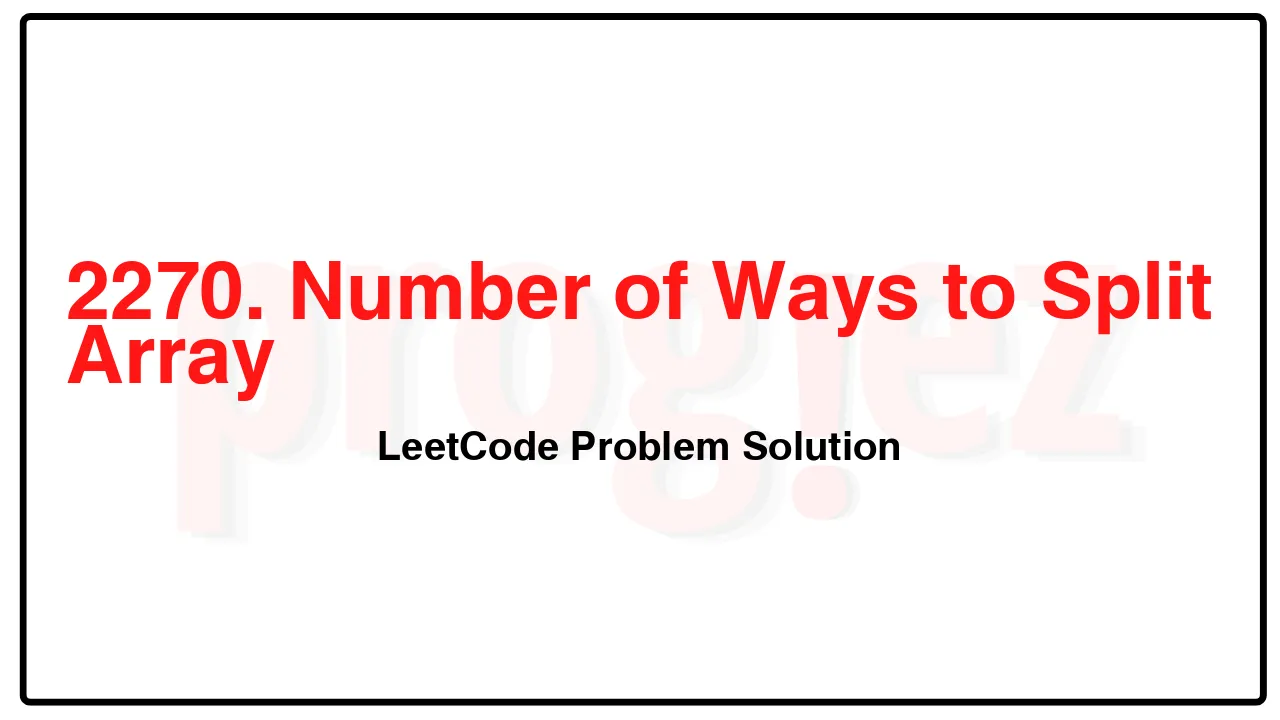
Problem Statement of Number of Ways to Split Array
You are given a 0-indexed integer array nums of length n.
nums contains a valid split at index i if the following are true:
The sum of the first i + 1 elements is greater than or equal to the sum of the last n – i – 1 elements.
There is at least one element to the right of i. That is, 0 <= i < n – 1.
Return the number of valid splits in nums.
Example 1:
Input: nums = [10,4,-8,7]
Output: 2
Explanation:
There are three ways of splitting nums into two non-empty parts:
– Split nums at index 0. Then, the first part is [10], and its sum is 10. The second part is [4,-8,7], and its sum is 3. Since 10 >= 3, i = 0 is a valid split.
– Split nums at index 1. Then, the first part is [10,4], and its sum is 14. The second part is [-8,7], and its sum is -1. Since 14 >= -1, i = 1 is a valid split.
– Split nums at index 2. Then, the first part is [10,4,-8], and its sum is 6. The second part is [7], and its sum is 7. Since 6 = 1, i = 1 is a valid split.
– Split nums at index 2. Then, the first part is [2,3,1], and its sum is 6. The second part is [0], and its sum is 0. Since 6 >= 0, i = 2 is a valid split.
Constraints:
2 <= nums.length <= 105
-105 <= nums[i] <= 105
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
2270. Number of Ways to Split Array LeetCode Solution in C++
class Solution {
public:
int waysToSplitArray(vector<int>& nums) {
int ans = 0;
long prefix = 0;
long suffix = accumulate(nums.begin(), nums.end(), 0L);
for (int i = 0; i < nums.size() - 1; ++i) {
prefix += nums[i];
suffix -= nums[i];
if (prefix >= suffix)
++ans;
}
return ans;
}
};
/* code provided by PROGIEZ */
2270. Number of Ways to Split Array LeetCode Solution in Java
class Solution {
public int waysToSplitArray(int[] nums) {
int ans = 0;
long prefix = 0;
long suffix = Arrays.stream(nums).asLongStream().sum();
for (int i = 0; i < nums.length - 1; ++i) {
prefix += nums[i];
suffix -= nums[i];
if (prefix >= suffix)
++ans;
}
return ans;
}
}
// code provided by PROGIEZ
2270. Number of Ways to Split Array LeetCode Solution in Python
class Solution:
def waysToSplitArray(self, nums: list[int]) -> int:
ans = 0
prefix = 0
suffix = sum(nums)
for i in range(len(nums) - 1):
prefix += nums[i]
suffix -= nums[i]
if prefix >= suffix:
ans += 1
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.