1768. Merge Strings Alternately LeetCode Solution
In this guide, you will get 1768. Merge Strings Alternately LeetCode Solution with the best time and space complexity. The solution to Merge Strings Alternately problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Merge Strings Alternately solution in C++
- Merge Strings Alternately solution in Java
- Merge Strings Alternately solution in Python
- Additional Resources
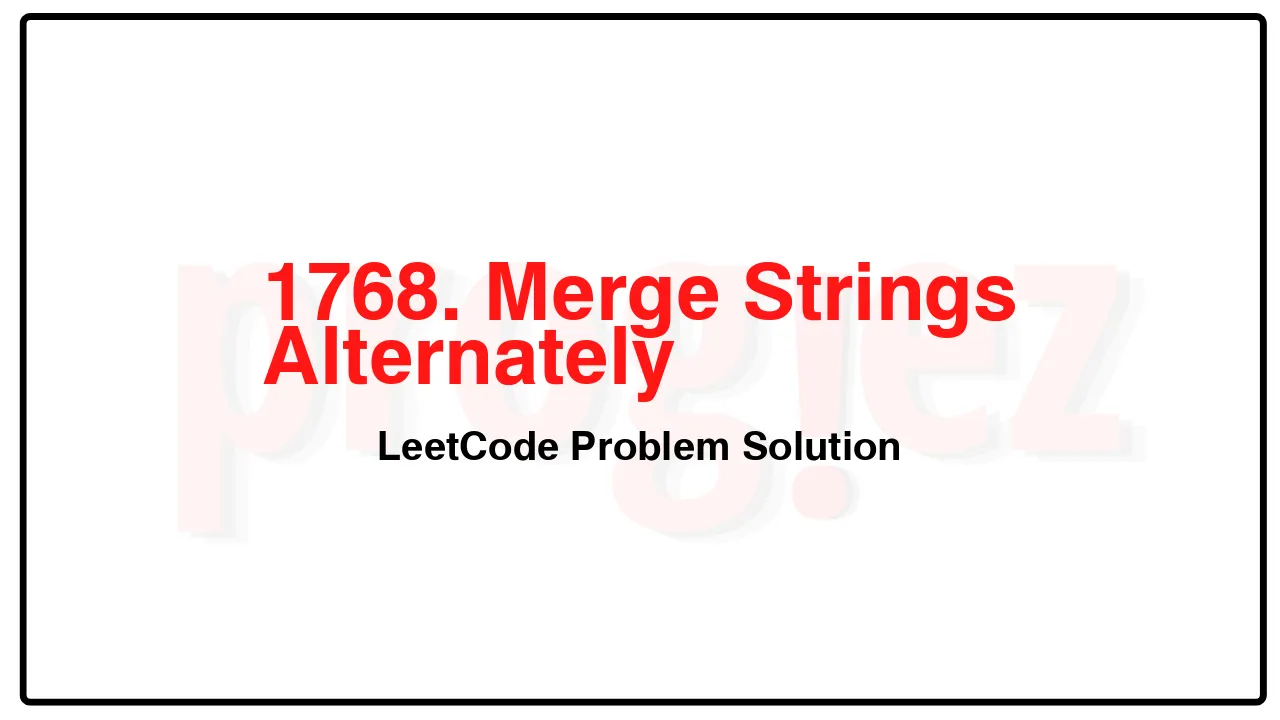
Problem Statement of Merge Strings Alternately
You are given two strings word1 and word2. Merge the strings by adding letters in alternating order, starting with word1. If a string is longer than the other, append the additional letters onto the end of the merged string.
Return the merged string.
Example 1:
Input: word1 = “abc”, word2 = “pqr”
Output: “apbqcr”
Explanation: The merged string will be merged as so:
word1: a b c
word2: p q r
merged: a p b q c r
Example 2:
Input: word1 = “ab”, word2 = “pqrs”
Output: “apbqrs”
Explanation: Notice that as word2 is longer, “rs” is appended to the end.
word1: a b
word2: p q r s
merged: a p b q r s
Example 3:
Input: word1 = “abcd”, word2 = “pq”
Output: “apbqcd”
Explanation: Notice that as word1 is longer, “cd” is appended to the end.
word1: a b c d
word2: p q
merged: a p b q c d
Constraints:
1 <= word1.length, word2.length <= 100
word1 and word2 consist of lowercase English letters.
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
1768. Merge Strings Alternately LeetCode Solution in C++
class Solution {
public:
string mergeAlternately(string word1, string word2) {
const int n = min(word1.length(), word2.length());
string prefix;
for (int i = 0; i < n; ++i) {
prefix += word1[i];
prefix += word2[i];
}
return prefix + word1.substr(n) + word2.substr(n);
}
};
/* code provided by PROGIEZ */
1768. Merge Strings Alternately LeetCode Solution in Java
class Solution {
public String mergeAlternately(String word1, String word2) {
final int n = Math.min(word1.length(), word2.length());
StringBuilder sb = new StringBuilder();
for (int i = 0; i < n; ++i) {
sb.append(word1.charAt(i));
sb.append(word2.charAt(i));
}
return sb.append(word1.substring(n)).append(word2.substring(n)).toString();
}
}
// code provided by PROGIEZ
1768. Merge Strings Alternately LeetCode Solution in Python
class Solution:
def mergeAlternately(self, word1: str, word2: str) -> str:
return ''.join(a + b for a, b in zip_longest(word1, word2, fillvalue=''))
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.