1004. Max Consecutive Ones III LeetCode Solution
In this guide, you will get 1004. Max Consecutive Ones III LeetCode Solution with the best time and space complexity. The solution to Max Consecutive Ones III problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Max Consecutive Ones III solution in C++
- Max Consecutive Ones III solution in Java
- Max Consecutive Ones III solution in Python
- Additional Resources
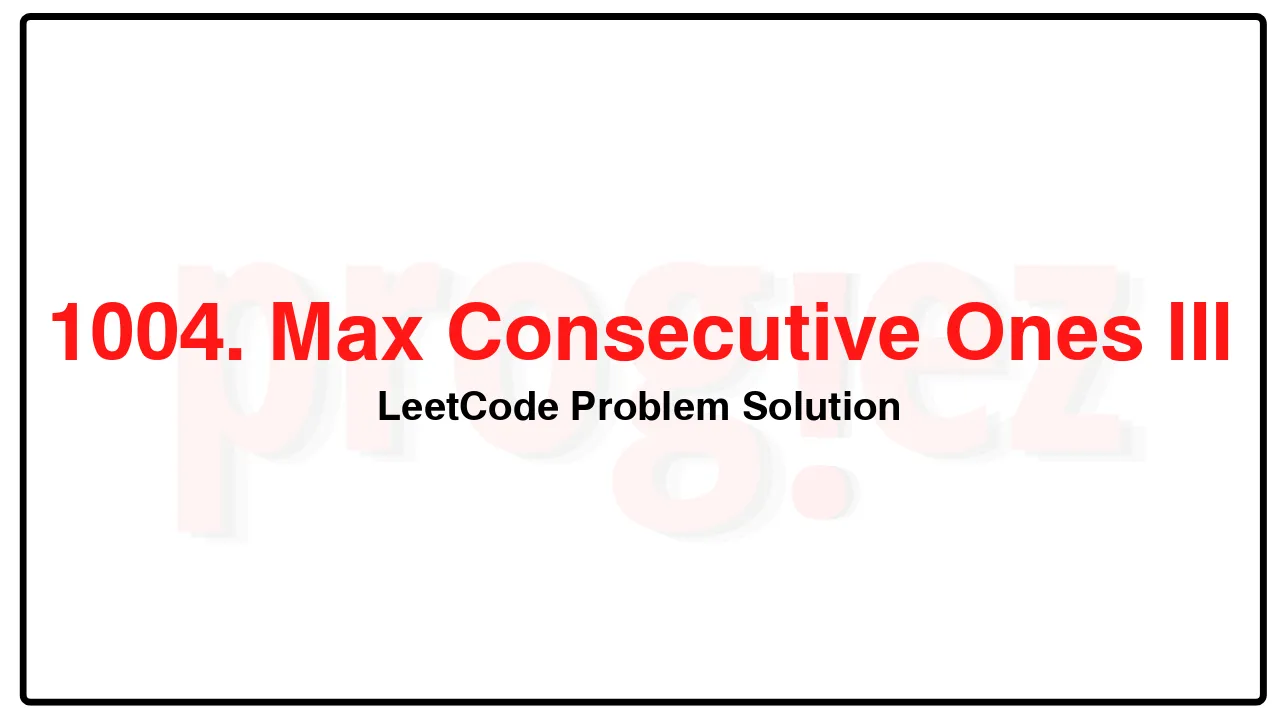
Problem Statement of Max Consecutive Ones III
Given a binary array nums and an integer k, return the maximum number of consecutive 1’s in the array if you can flip at most k 0’s.
Example 1:
Input: nums = [1,1,1,0,0,0,1,1,1,1,0], k = 2
Output: 6
Explanation: [1,1,1,0,0,1,1,1,1,1,1]
Bolded numbers were flipped from 0 to 1. The longest subarray is underlined.
Example 2:
Input: nums = [0,0,1,1,0,0,1,1,1,0,1,1,0,0,0,1,1,1,1], k = 3
Output: 10
Explanation: [0,0,1,1,1,1,1,1,1,1,1,1,0,0,0,1,1,1,1]
Bolded numbers were flipped from 0 to 1. The longest subarray is underlined.
Constraints:
1 <= nums.length <= 105
nums[i] is either 0 or 1.
0 <= k <= nums.length
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(1)
1004. Max Consecutive Ones III LeetCode Solution in C++
class Solution {
public:
int longestOnes(vector<int>& nums, int k) {
int ans = 0;
for (int l = 0, r = 0; r < nums.size(); ++r) {
if (nums[r] == 0)
--k;
while (k < 0)
if (nums[l++] == 0)
++k;
ans = max(ans, r - l + 1);
}
return ans;
}
};
/* code provided by PROGIEZ */
1004. Max Consecutive Ones III LeetCode Solution in Java
class Solution {
public int longestOnes(int[] nums, int k) {
int ans = 0;
for (int l = 0, r = 0; r < nums.length; ++r) {
if (nums[r] == 0)
--k;
while (k < 0)
if (nums[l++] == 0)
++k;
ans = Math.max(ans, r - l + 1);
}
return ans;
}
}
// code provided by PROGIEZ
1004. Max Consecutive Ones III LeetCode Solution in Python
class Solution:
def longestOnes(self, nums: list[int], k: int) -> int:
ans = 0
l = 0
for r, num in enumerate(nums):
if num == 0:
k -= 1
while k < 0:
if nums[l] == 0:
k += 1
l += 1
ans = max(ans, r - l + 1)
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.