949. Largest Time for Given Digits LeetCode Solution
In this guide, you will get 949. Largest Time for Given Digits LeetCode Solution with the best time and space complexity. The solution to Largest Time for Given Digits problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Largest Time for Given Digits solution in C++
- Largest Time for Given Digits solution in Java
- Largest Time for Given Digits solution in Python
- Additional Resources
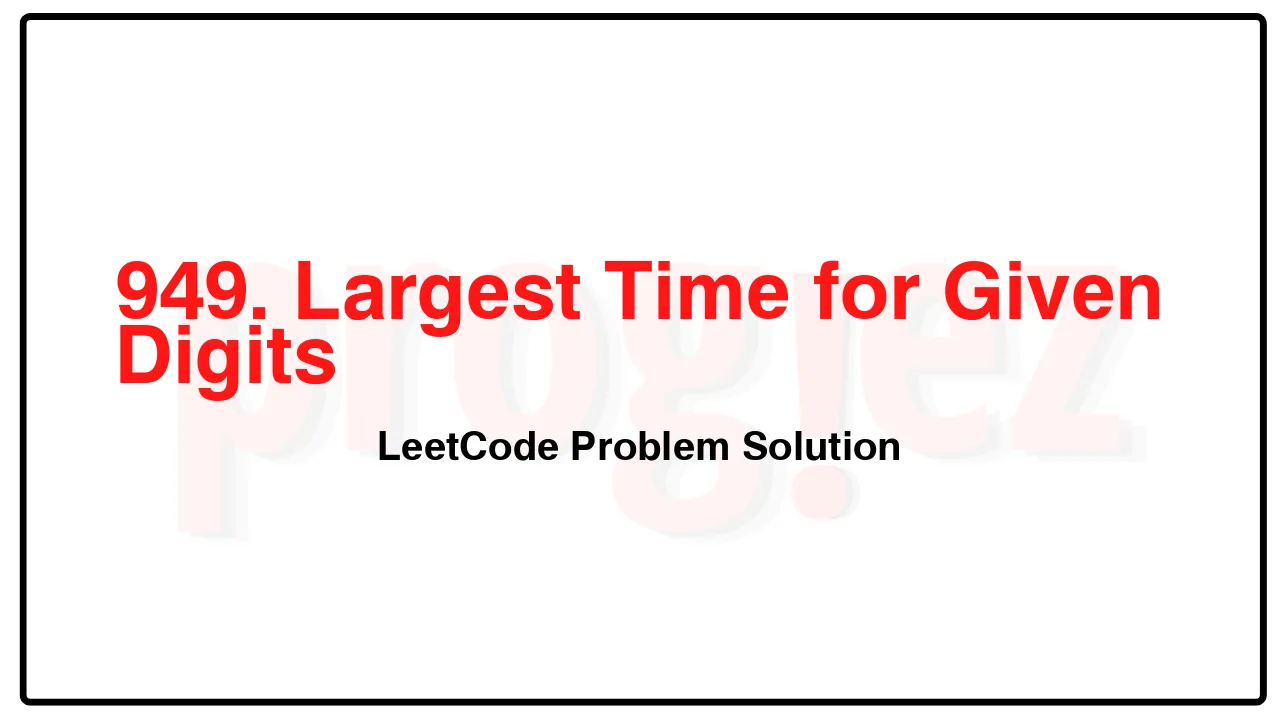
Problem Statement of Largest Time for Given Digits
Given an array arr of 4 digits, find the latest 24-hour time that can be made using each digit exactly once.
24-hour times are formatted as “HH:MM”, where HH is between 00 and 23, and MM is between 00 and 59. The earliest 24-hour time is 00:00, and the latest is 23:59.
Return the latest 24-hour time in “HH:MM” format. If no valid time can be made, return an empty string.
Example 1:
Input: arr = [1,2,3,4]
Output: “23:41”
Explanation: The valid 24-hour times are “12:34”, “12:43”, “13:24”, “13:42”, “14:23”, “14:32”, “21:34”, “21:43”, “23:14”, and “23:41”. Of these times, “23:41” is the latest.
Example 2:
Input: arr = [5,5,5,5]
Output: “”
Explanation: There are no valid 24-hour times as “55:55” is not valid.
Constraints:
arr.length == 4
0 <= arr[i] <= 9
Complexity Analysis
- Time Complexity:
- Space Complexity:
949. Largest Time for Given Digits LeetCode Solution in C++
class Solution {
public:
string largestTimeFromDigits(vector<int>& arr) {
string ans;
for (int i = 0; i < 4; ++i)
for (int j = 0; j < 4; ++j)
for (int k = 0; k < 4; ++k) {
if (i == j || i == k || j == k)
continue;
const string hours = to_string(arr[i]) + to_string(arr[j]);
const string minutes =
to_string(arr[k]) + to_string(arr[6 - i - j - k]);
if (hours < "24" && minutes < "60")
ans = max(ans, hours + ':' + minutes);
}
return ans;
}
};
/* code provided by PROGIEZ */
949. Largest Time for Given Digits LeetCode Solution in Java
class Solution {
public String largestTimeFromDigits(int[] arr) {
String ans = "";
for (int i = 0; i < 4; ++i)
for (int j = 0; j < 4; ++j)
for (int k = 0; k < 4; ++k) {
if (i == j || i == k || j == k)
continue;
final String hours = "" + arr[i] + arr[j];
final String minutes = "" + arr[k] + arr[6 - i - j - k];
final String time = hours + ':' + minutes;
if (hours.compareTo("24") < 0 && minutes.compareTo("60") < 0 && ans.compareTo(time) < 0)
ans = time;
}
return ans;
}
}
// code provided by PROGIEZ
949. Largest Time for Given Digits LeetCode Solution in Python
class Solution:
def largestTimeFromDigits(self, arr: list[int]) -> str:
for time in itertools.permutations(sorted(arr, reverse=True)):
if time[:2] < (2, 4) and time[2] < 6:
return '%d%d:%d%d' % time
return ''
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.