718. Maximum Length of Repeated Subarray LeetCode Solution
In this guide, you will get 718. Maximum Length of Repeated Subarray LeetCode Solution with the best time and space complexity. The solution to Maximum Length of Repeated Subarray problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Maximum Length of Repeated Subarray solution in C++
- Maximum Length of Repeated Subarray solution in Java
- Maximum Length of Repeated Subarray solution in Python
- Additional Resources
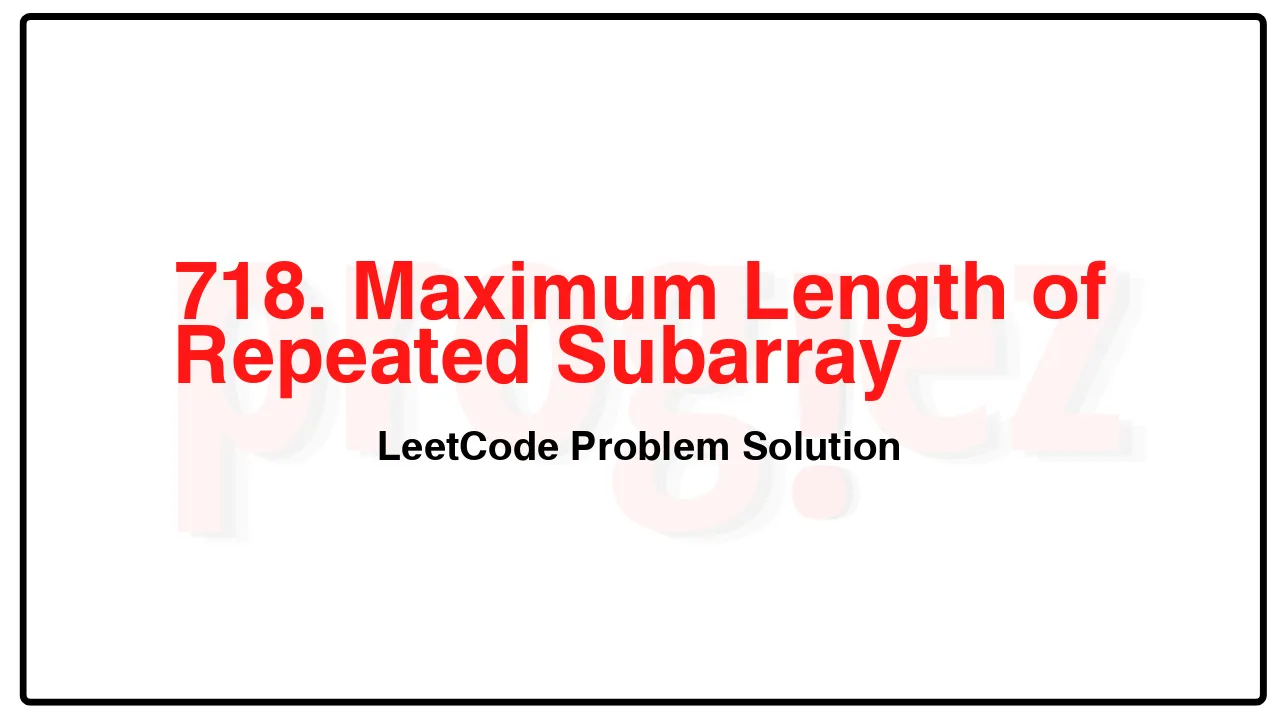
Problem Statement of Maximum Length of Repeated Subarray
Given two integer arrays nums1 and nums2, return the maximum length of a subarray that appears in both arrays.
Example 1:
Input: nums1 = [1,2,3,2,1], nums2 = [3,2,1,4,7]
Output: 3
Explanation: The repeated subarray with maximum length is [3,2,1].
Example 2:
Input: nums1 = [0,0,0,0,0], nums2 = [0,0,0,0,0]
Output: 5
Explanation: The repeated subarray with maximum length is [0,0,0,0,0].
Constraints:
1 <= nums1.length, nums2.length <= 1000
0 <= nums1[i], nums2[i] <= 100
Complexity Analysis
- Time Complexity: O(mn)
- Space Complexity: O(mn)
718. Maximum Length of Repeated Subarray LeetCode Solution in C++
class Solution {
public:
int findLength(vector<int>& nums1, vector<int>& nums2) {
const int m = nums1.size();
const int n = nums2.size();
int ans = 0;
// dp[i][j] := the maximum length of a subarray that appears in both
// nums1[i..m) and nums2[j..n)
vector<vector<int>> dp(m + 1, vector<int>(n + 1));
for (int i = m - 1; i >= 0; --i)
for (int j = n - 1; j >= 0; --j)
if (nums1[i] == nums2[j]) {
dp[i][j] = dp[i + 1][j + 1] + 1;
ans = max(ans, dp[i][j]);
}
return ans;
}
};
/* code provided by PROGIEZ */
718. Maximum Length of Repeated Subarray LeetCode Solution in Java
class Solution {
public int findLength(int[] nums1, int[] nums2) {
final int m = nums1.length;
final int n = nums2.length;
int ans = 0;
// dp[i][j] := the maximum length of a subarray that appears in both
// nums1[i..m) and nums2[j..n)
int[][] dp = new int[m + 1][n + 1];
for (int i = m - 1; i >= 0; --i)
for (int j = n - 1; j >= 0; --j)
if (nums1[i] == nums2[j]) {
dp[i][j] = dp[i + 1][j + 1] + 1;
ans = Math.max(ans, dp[i][j]);
}
return ans;
}
}
// code provided by PROGIEZ
718. Maximum Length of Repeated Subarray LeetCode Solution in Python
class Solution:
def findLength(self, nums1: list[int], nums2: list[int]) -> int:
m = len(nums1)
n = len(nums2)
ans = 0
# dp[i][j] := the maximum length of a subarray that appears in both
# nums1[i..m) and nums2[j..n)
dp = [[0] * (n + 1) for _ in range(m + 1)]
for i in reversed(range(m)):
for j in reversed(range(n)):
if nums1[i] == nums2[j]:
dp[i][j] = dp[i + 1][j + 1] + 1
ans = max(ans, dp[i][j])
return ans
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.