670. Maximum Swap LeetCode Solution
In this guide, you will get 670. Maximum Swap LeetCode Solution with the best time and space complexity. The solution to Maximum Swap problem is provided in various programming languages like C++, Java, and Python. This will be helpful for you if you are preparing for placements, hackathons, interviews, or practice purposes. The solutions provided here are very easy to follow and include detailed explanations.
Table of Contents
- Problem Statement
- Complexity Analysis
- Maximum Swap solution in C++
- Maximum Swap solution in Java
- Maximum Swap solution in Python
- Additional Resources
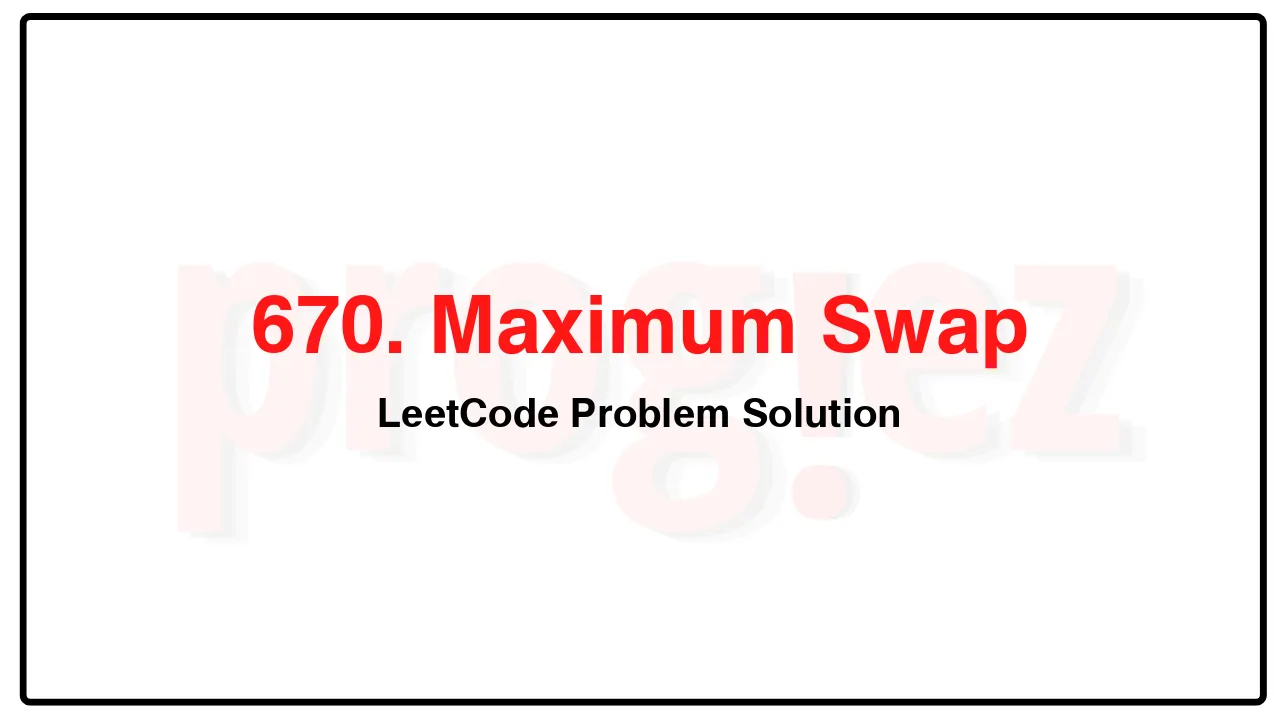
Problem Statement of Maximum Swap
You are given an integer num. You can swap two digits at most once to get the maximum valued number.
Return the maximum valued number you can get.
Example 1:
Input: num = 2736
Output: 7236
Explanation: Swap the number 2 and the number 7.
Example 2:
Input: num = 9973
Output: 9973
Explanation: No swap.
Constraints:
0 <= num <= 108
Complexity Analysis
- Time Complexity: O(n)
- Space Complexity: O(n)
670. Maximum Swap LeetCode Solution in C++
class Solution {
public:
int maximumSwap(int num) {
string s = to_string(num);
vector<int> lastIndex(10, -1); // {digit: last index}
for (int i = 0; i < s.length(); ++i)
lastIndex[s[i] - '0'] = i;
for (int i = 0; i < s.length(); ++i)
for (int d = 9; d > s[i] - '0'; --d)
if (lastIndex[d] > i) {
swap(s[i], s[lastIndex[d]]);
return stoi(s);
}
return num;
}
};
/* code provided by PROGIEZ */
670. Maximum Swap LeetCode Solution in Java
class Solution {
public int maximumSwap(int num) {
char[] s = Integer.toString(num).toCharArray();
int[] lastIndex = new int[10]; // {digit: last index}
for (int i = 0; i < s.length; ++i)
lastIndex[s[i] - '0'] = i;
for (int i = 0; i < s.length; ++i)
for (int d = 9; d > s[i] - '0'; --d)
if (lastIndex[d] > i) {
s[lastIndex[d]] = s[i];
s[i] = (char) ('0' + d);
return Integer.parseInt(new String(s));
}
return num;
}
}
// code provided by PROGIEZ
670. Maximum Swap LeetCode Solution in Python
class Solution:
def maximumSwap(self, num: int) -> int:
s = list(str(num))
dict = {c: i for i, c in enumerate(s)}
for i, c in enumerate(s):
for digit in reversed(string.digits):
if digit <= c:
break
if digit in dict and dict[digit] > i:
s[i], s[dict[digit]] = digit, s[i]
return int(''.join(s))
return num
# code by PROGIEZ
Additional Resources
- Explore all LeetCode problem solutions at Progiez here
- Explore all problems on LeetCode website here
Happy Coding! Keep following PROGIEZ for more updates and solutions.